Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial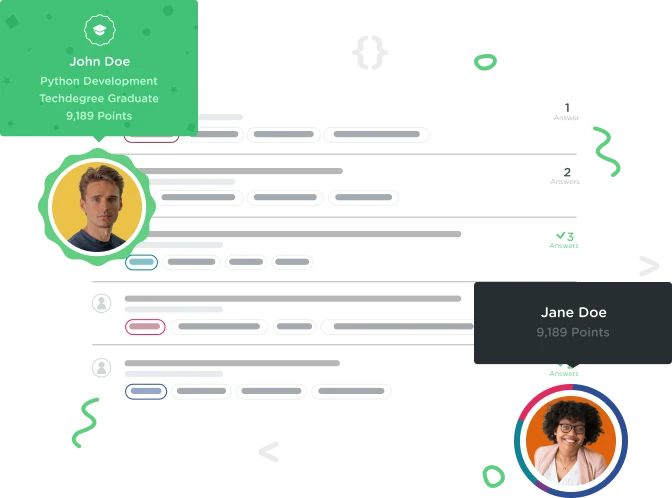

Cyrus Hung
2,571 Pointswhy do we need initalizers?
Why do we need an initialiser? It seems the struct works even we don't use an initializer
6 Answers
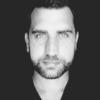
Jhoan Arango
14,575 PointsHello :
You initialize to be able to create an instance of a class, or struct. Without them you can't create an instance of it. Structs create initializers by default, classes in the other hand, you have to create an initializer for it.
Initializers are what gives the store properties their values to create an object.
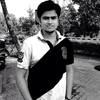
Ritesh R
Courses Plus Student 17,865 Points@Piers FC this is what i did & it worked!! Hope it helps..
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
let color = RGBColor(red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0)
color.description
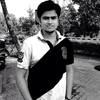
Ritesh R
Courses Plus Student 17,865 Pointscolor.description gives the output "red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0"

Piers FC
10,402 PointsHello treehouse community.
I can't work out how to complete the final task for the section of Complex Data Structures in Object-Orientated Swift 2.0? Would be great if someone could show me the solution,
I have come up with a hacky solution but I know its not what is required and probably not the best way to solve the problem
Here is my code:
struct RGBColor { let red: Double let green: Double let blue: Double let alpha: Double
var description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double, description: String) { self.red = red self.green = green self.blue = blue self.alpha = alpha self.description = description }
mutating func concatProperties() -> String{ description = "red: (red), green: (green), blue: (blue), alpha: (alpha)" return description
}
}
var p4 = RGBColor(red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0, description: "light blue") p4.concatProperties()
I look forward to hearing your answers / help.
Thanks

swiftfun
4,039 PointsWhy after writing the following code I can't see the output in the right panel of xCode? Screenshot: https://www.dropbox.com/s/3n511frb0f1yha5/Screenshot%202016-02-18%2015.09.43.png?dl=0
Code used:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(self.red), green: \(self.green), blue: \(self.blue), alpha: \(self.alpha)"
}
// Add your code below
}
let color = RGBColor(red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0)
color.description
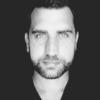
Jhoan Arango
14,575 PointsHello :
Try deleting the self. out of the concatenation.
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
When you use the word self, you are referring to the store property and not the parameter.
Good luck

swiftfun
4,039 PointsStill nothing is showing up on the right panel: https://www.dropbox.com/s/4df7twr7gz7z41u/Screenshot%202016-02-18%2016.37.32.png?dl=0
I would like to see the string output (ie. Red: 86.0, etc)
Is there something wrong with my Xcode or what?
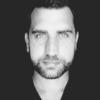
Jhoan Arango
14,575 PointsYou took off the self out of description, and not the ones I suggested on my code.
Please replace your code with mine.
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
Should be red: (red), and NOT red: (self.red).

swiftfun
4,039 PointsHello,
Apparently it was an issue with my Xcode, restarted mac and it is now showing the output correctly (even with the self in the concatenation - which should be fine)
Thank you
Chi Chiem Duc
864 PointsChi Chiem Duc
864 PointsIsn't it us programmers give the value to the stored properties when we create instances of structs? I still don't get how initializers work.
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsHi Chi:
In order for you to be able to create an instance of a class, or struct, you need to give values to their store properties correct ? Well, that's what the initializer are for, to INITIALIZE the store properties. Therefore a complete instance will be created.
For example.
We now have created a proper class, and also created an instance of it via its initializer.
If you have any more questions, please let me know.
Good luck
Chi Chiem Duc
864 PointsChi Chiem Duc
864 PointsNow I get it, when we give the value to the instance, it goes to the value in the init function. I've always thought it will go straight to the stored properties itself. Thank you so much :D.
Kuang-Wen, Huang
2,525 PointsKuang-Wen, Huang
2,525 PointsHello Jhoan: I still have some questions though... I thought initialize is like giving a value to the constant or variety we declare before. But it seems like you just declare another variety for the first one (like, storePropertie = initializeFirst). I just can't get the reason why we do that. Why we don't give a number, for example "5" as it's a Int here? It really bothers me a lot...
thanks a lot!
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsHello Kuang-Wen:
You are right, initialize is when you first declare a variable or a constant, and you give it an initial value. But when creating a class or a struct you may declare a variable within the class and not give it an initial value. This can be done by making it an optional.
Let's create a class ( Person ), this class creates an object or in this case a Person. This class has 3 store properties, which 2 ( name & lastName ) of them are being set up to be initialized ( or given a value ) by the initializer. The third store property ( age ) which is of type int, has no initial value nor is being set up by the initializer. But since it's a variable and IT'S an optional, it does not required to be initialized in order for you to create an instance of Person.
Example:
I encourage you to copy this code and paste it into a playground in Xcode, it will make more sense to you.
If you have another question about this, please let me know by tagging me or ask a more detailed question in the forum and then tag my name on there @jhoanarango
Good luck and hope this helps
Kuang-Wen, Huang
2,525 PointsKuang-Wen, Huang
2,525 PointsHello, Jhoan Arango First of all thanks for your instant help! Now it really make sense. But there is another question I want to know. Why "var" don't need to be initialized while "let" need to be? I have rewrite your code in the playground and find out that if var something is an OPTIONAL, than without initialized will be Okay, but it doesn't work on let something. What's the different? Can't "let something?" output nil without being initialized?
Again, thanks a lot! The codes you write is clear and very helpful! Also please forgive my bad English!
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsHello:
The age property is an optional Int property, and so it automatically receives a default value of nil, even though this value is not written in the code. If this was to be done with a constant, then it's value could have not been changed during initialization. That's why you can't make a constant an optional, because then they will always be "nil".
I hope this answers your question.