Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial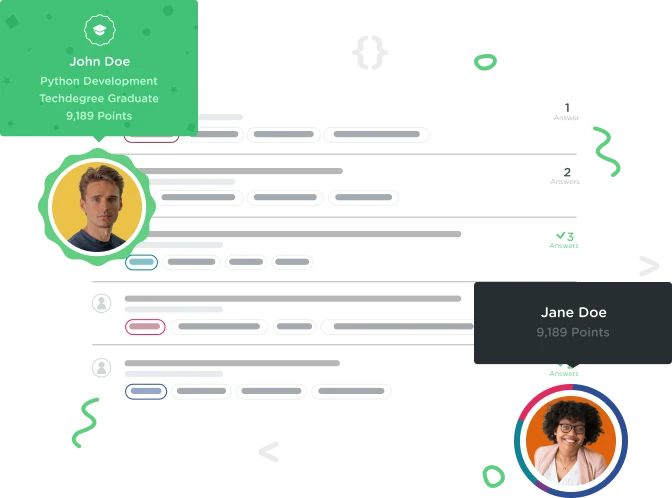
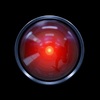
Paul Stevens
4,125 PointsAdding friend requesting in Ribbit App.
My attempt at adding the Request Friendship function to the Ribbit App.
This code is really untidy and needs a lot of work. I'm sure there are better ways to do this than I have done, but if someone else is trying to add this then maybe this will help get them started.
When user1 selects a person who is not a friend it now displays a dialog box allowing you to type a message into to it to send with the request. when user1 chooses "ok" the request is sent to user2. User2 can then "accept" or "deny" the request. If user2 accepts then user1 is added as a friend and a message is sent back to user1 saying they accepted. When user1 reads the message user2 is added as their friend. If user2 denies the request then a message is sent to user1 informing them it was not accepted.
When user1 selects someone who is a friend it asks them if they want to delete that friend. If user1 says "ok" then a message is sent to user2 requesting the app to delete them from the friends list of user2. A message is displayed saying that the friend has been removed.
Here is the main parts of the code, again sorry for it being so messy. :D
Also the code has been changed to allow text messages to be sent as well as pictures and videos.
This is the getView() Method from the MessageAdapter:
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
convertView = LayoutInflater.from(mContext).inflate(R.layout.message_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView)convertView.findViewById(R.id.message_icon);
holder.nameLabel = (TextView)convertView.findViewById(R.id.sender_label);
convertView.setTag(holder);
}else {
holder = (ViewHolder)convertView.getTag();
}
ParseObject message =mMessages.get(position);
if (message.getString(ParseConstants.KEY_FILE_TYPE).equals(ParseConstants.TYPE_IMAGE)) {
holder.iconImageView.setImageResource(R.drawable.ic_action_picture);
}else if (message.getString(ParseConstants.KEY_FILE_TYPE).equals(ParseConstants.TYPE_VIDEO)){
holder.iconImageView.setImageResource(R.drawable.ic_action_play_over_video);
}else if (message.getString(ParseConstants.KEY_FILE_TYPE).equals(ParseConstants.TYPE_TEXT)){
holder.iconImageView.setImageResource(R.drawable.ic_action_chat_light);
}else if (message.getString(ParseConstants.KEY_FILE_TYPE).equals(ParseConstants.TYPE_FRIEND_REQUEST)) {
holder.iconImageView.setImageResource(R.drawable.ic_action_add_person);
}else if (message.getString(ParseConstants.KEY_FILE_TYPE).equals(ParseConstants.TYPE_FRIEND_ACCEPT)) {
holder.iconImageView.setImageResource(R.drawable.ic_action_add_person);
}else if (message.getString(ParseConstants.KEY_FILE_TYPE).equals(ParseConstants.TYPE_FRIEND_DENY)) {
holder.iconImageView.setImageResource(R.drawable.ic_action_add_person);
}else if (message.getString(ParseConstants.KEY_FILE_TYPE).equals(ParseConstants.TYPE_FRIEND_REMOVE)) {
holder.iconImageView.setImageResource(R.drawable.ic_action_add_person);
}
holder.nameLabel.setText(message.getString(ParseConstants.KEY_SENDER_NAME));
}
This is the onListItemClick() Method from the InboxFragment:
@Override
public void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
ParseObject message = mMessages.get(position);
String messageType = message.getString(ParseConstants.KEY_FILE_TYPE);
senderName = message.getString(ParseConstants.KEY_SENDER_NAME);
senderId = message.getString(ParseConstants.KEY_SENDER_ID);
String displayMessage = message.getString(ParseConstants.KEY_MESSAGE);
ParseFile file = message.getParseFile(ParseConstants.KEY_FILE);
if (messageType.equals(ParseConstants.TYPE_IMAGE)) {
//view the image
Uri fileUri = Uri.parse(file.getUrl());
Intent intent = new Intent(getActivity(), ViewImageActivity.class);
intent.setData(fileUri);
startActivity(intent);
}else if (messageType.equals(ParseConstants.TYPE_VIDEO)){
Uri fileUri = Uri.parse(file.getUrl());
//view the video
Intent intent = new Intent(Intent.ACTION_VIEW, fileUri);
intent.setDataAndType(fileUri, "video/*");
startActivity(intent);
}else if (messageType.equals(ParseConstants.TYPE_TEXT)){
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setTitle("Message from: " + senderName + ".");
builder.setMessage(displayMessage);
builder.setPositiveButton("Ok", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
}
});
AlertDialog dialog = builder.create();
dialog.show();
}else if (messageType.equals(ParseConstants.TYPE_FRIEND_REQUEST)){
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setTitle("Friend Request from " + senderName + ".");
builder.setMessage(displayMessage);
builder.setPositiveButton("Accept", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
//Accept request
//Add senders id to friends list
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDSRELATION);
getActivity().setProgressBarIndeterminate(true);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.whereEqualTo("objectId", senderId);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
getActivity().setProgressBarIndeterminate(false);
if (e == null){
mFriendsRelation.add(users.get(0));
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null) {
Log.e("Inbox Fragment", e.getMessage());
}
}
});
}else {
Log.e("Inbox Fragment", e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
//send accepted message
String acceptedMessage = mCurrentUser.getUsername() + " has accepted your friend request.";
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
recipientId.add(senderId);
message.put(ParseConstants.KEY_RECIPIENT_IDS, recipientId);
message.put(ParseConstants.KEY_MESSAGE, acceptedMessage);
message.put(ParseConstants.KEY_FILE_TYPE, ParseConstants.TYPE_FRIEND_ACCEPT);
message.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e ==null){
//success
//Tell the user that the new friend was added.
Toast.makeText(getActivity(), senderName + " is now your friend.", Toast.LENGTH_LONG).show();
}else{
String errorMessage = e.getMessage();
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage("Error accepting friend request." + errorMessage);
builder.setTitle(R.string.error_selecting_file_title);
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
});
builder.setNegativeButton("Deny", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
//Refuse request
Toast.makeText(getActivity(), "Request Denied.", Toast.LENGTH_LONG).show();
//Send denied message.
mCurrentUser = ParseUser.getCurrentUser();
String acceptedMessage = mCurrentUser.getUsername() + " has denied your friend request.";
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
recipientId.add(senderId);
message.put(ParseConstants.KEY_RECIPIENT_IDS, recipientId);
message.put(ParseConstants.KEY_MESSAGE, acceptedMessage);
message.put(ParseConstants.KEY_FILE_TYPE, ParseConstants.TYPE_FRIEND_DENY);
message.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e ==null){
//success
//Tell the user that the new friend was added.
Toast.makeText(getActivity(), "Request denied.", Toast.LENGTH_LONG).show();
}else{
String errorMessage = e.getMessage();
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage("Error denying friend request." + errorMessage);
builder.setTitle(R.string.error_selecting_file_title);
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
});
builder.show();
}else if (messageType.equals(ParseConstants.TYPE_FRIEND_ACCEPT)){
//Display dialog showing that friend has accepted.
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(senderName + " is now your friend.");
builder.setTitle("Friend Request.");
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
//Add new friends name to relation list
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDSRELATION);
getActivity().setProgressBarIndeterminate(true);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.whereEqualTo("objectId", senderId);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
getActivity().setProgressBarIndeterminate(false);
if (e == null){
mFriendsRelation.add(users.get(0));
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null) {
Log.e("Inbox Fragment", e.getMessage());
}
}
});
}else {
Log.e("Inbox Fragment", e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
} else if (messageType.equals(ParseConstants.TYPE_FRIEND_DENY)){
//Display dialog showing that friend has denied.
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(senderName + " has denied the friend request.");
builder.setTitle("Friend Request.");
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
} else if (messageType.equals(ParseConstants.TYPE_FRIEND_REMOVE)){
//Display dialog showing that a friend has requested removal.
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(senderName + " has been removed from your friends list.");
builder.setTitle("Friend Update.");
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
//Remove friends name from relation list
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDSRELATION);
getActivity().setProgressBarIndeterminate(true);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.whereEqualTo("objectId", senderId);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
getActivity().setProgressBarIndeterminate(false);
if (e == null){
mFriendsRelation.remove(users.get(0));
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null) {
Log.e("Inbox Fragment", e.getMessage());
}
}
});
}else {
Log.e("Inbox Fragment", e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
//delete the message
List<String> ids = message.getList(ParseConstants.KEY_RECIPIENT_IDS);
if(ids.size() == 1) {
//last recipient, delete the message
message.deleteInBackground();
}else {
//remove recipients name
ids.remove(ParseUser.getCurrentUser().getObjectId());
ArrayList<String> idsToRemove = new ArrayList<String>();
idsToRemove.add(ParseUser.getCurrentUser().getObjectId());
message.removeAll(ParseConstants.KEY_RECIPIENT_IDS, idsToRemove);
message.saveInBackground();
}
}
This is the onListItemClick() Method from the EditFriendsActivity:
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
//Create a variable to store the position of the selected user is the list.
final int friendPosition = position;
//Create a variable to store the selected users name.
final String friendName = userList.get(position);
final String friendId = userIdList.get(position);
if (getListView().isItemChecked(position)) {//If the selected user is not already a friend.
//Create a dialog to ask if the User is sure they want to send a friend request.
AlertDialog.Builder alert = new AlertDialog.Builder(this);
alert.setTitle(R.string.dialog_add_friend_title);
//TODO Had a problem using a string resource on the next line. Just kept giving me the string Id instead of the text.
//alert.setMessage(R.string.dialog_add_friend_message + friendName + ".");
alert.setMessage("Enter message below to send with your friend request to " + friendName + ".");
final EditText input = new EditText(this);
alert.setView(input);
alert.setPositiveButton("Ok", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
//Send a request to the friend.
String requestMessage = input.getText().toString();
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
recipientId.add(friendId);
message.put(ParseConstants.KEY_RECIPIENT_IDS, recipientId);
message.put(ParseConstants.KEY_MESSAGE, requestMessage);
message.put(ParseConstants.KEY_FILE_TYPE, ParseConstants.TYPE_FRIEND_REQUEST);
message.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e ==null){
//success
Toast.makeText(EditFriendsActivity.this, R.string.success_friend_request_sent, Toast.LENGTH_LONG).show();
}else{
String errorMessage = e.getMessage();
AlertDialog.Builder builder = new AlertDialog.Builder(EditFriendsActivity.this);
builder.setMessage(R.string.dialog_error_sending_friend_request + errorMessage);
builder.setTitle(R.string.error_selecting_file_title);
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
});
alert.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
//Friend request cancelled.
}
});
alert.show();
//Set friend List Item back to unchecked.
getListView().setItemChecked(friendPosition, false);
}else {//If the selected user is a friend already.
//Create a dialog to ask if the User is sure they want to delete the friend.
AlertDialog.Builder alert = new AlertDialog.Builder(this);
alert.setTitle(R.string.dialog_delete_friend_title);
//TODO Had a problem using a string resource on the next line. Just kept giving me the string Id instead of the text.
//alert.setMessage(R.string.dialog_delete_friend_message + friendName + ".");
alert.setMessage("Are you sure you want to delete your friend " + friendName + ".");
alert.setPositiveButton("Ok", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
//Remove friend from list.
mFriendsRelation.remove(mUsers.get(friendPosition));
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null) {
Log.e("EditFriendsActivity", e.getMessage());
}
}
});
// Send message to friends application requesting deletion from their list.
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
recipientId.add(friendId);
message.put(ParseConstants.KEY_RECIPIENT_IDS, recipientId);
message.put(ParseConstants.KEY_MESSAGE, "");
message.put(ParseConstants.KEY_FILE_TYPE, ParseConstants.TYPE_FRIEND_REMOVE);
message.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e ==null){
//success
Toast.makeText(EditFriendsActivity.this, friendName + " removed.", Toast.LENGTH_LONG).show();
}else{
String errorMessage = e.getMessage();
AlertDialog.Builder builder = new AlertDialog.Builder(EditFriendsActivity.this);
builder.setMessage("Error removing friend." + errorMessage);
builder.setTitle(R.string.error_selecting_file_title);
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
});
alert.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
//Deletion cancelled. Set friend List Item back to checked.
getListView().setItemChecked(friendPosition, true);
}
});
alert.show();
}
//Update the User
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null) {
Log.e(TAG, e.getMessage());
}
}
});
}
As I said I'm sure this could be done in a better way :D but I'm pretty new to this and happy that it actually works lol. Code needs a lot of tidying up, things put into string resources etc, but hopefully this might help if you are trying the same thing.
Paul :D
12 Answers

Fuseini Isaac
Courses Plus Student 2,225 PointsI still can get the it to work..i am getting a lot of errors, can you paste give a bit more of the code where you made changes including the "protected" part at the top. i mean defining the terms up in the classes
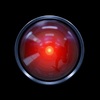
Paul Stevens
4,125 PointsHello,
Here is some more from the EditFriendsActivity:
public static final String TAG = EditFriendsActivity.class.getSimpleName();
protected List<ParseUser> mUsers;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
//***********************I ADDED A FEW EXTRA VARIABLES HERE.
protected ArrayList<String> userList = new ArrayList<String>();
protected ArrayList<String> userIdList = new ArrayList<String>();
protected ArrayList<String> recipientId = new ArrayList<String>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_edit_friends);
// Show the Up button in the action bar.
setupActionBar();
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
protected void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDSRELATION);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.orderByAscending(ParseConstants.KEY_USERNAME);
query.setLimit(1000);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
setProgressBarIndeterminateVisibility(false);
if (e == null){
mUsers = users;
String[] usernames = new String[mUsers.size()];
int i = 0;
for (ParseUser user: mUsers) {
usernames[i] = user.getUsername();
//***********************I ADDED THESE IN HERE.
userList.add(user.getUsername());
userIdList.add(user.getObjectId());
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(EditFriendsActivity.this, android.R.layout.simple_list_item_checked, usernames);
setListAdapter(adapter);
addFriendCheckmarks();
}else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(EditFriendsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
This is the top of the InboxFragment:
public class InboxFragment extends ListFragment {
protected List<ParseObject> mMessages;
protected List<ParseUser> mUsers;
public String senderId;
public String senderName;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected ArrayList<String> recipientId = new ArrayList<String>();
I think that's all, but just ask if there is anything else.
Paul :)

Fuseini Isaac
Courses Plus Student 2,225 Pointscan you add the parse constants used.. getting a lot of errors here Ben Jakuben can you help with the debugging.
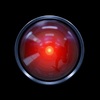
Paul Stevens
4,125 PointsHello,
Sure, here are the Constants:
public final class ParseConstants {
//Class name
public static final String CLASS_MESSAGES = "Messages";
//Field names
public static final String KEY_USERNAME = "username";
public static final String KEY_FRIENDSRELATION = "friendsRelation";
public static final String KEY_RECIPIENT_IDS = "recipientIds";
public static final String KEY_SENDER_ID ="senderId";
public static final String KEY_SENDER_NAME ="senderName";
public static final String KEY_FILE ="file";
public static final String KEY_FILE_TYPE ="fileType";
public static final String KEY_CREATED_AT = "createdAt";
public static final String KEY_MESSAGE = "themessage";
public static final String TYPE_IMAGE ="image";
public static final String TYPE_VIDEO ="video";
public static final String TYPE_TEXT ="message";
public static final String TYPE_FRIEND_REQUEST = "friendRequest";
public static final String TYPE_FRIEND_ACCEPT = "friendAccept";
public static final String TYPE_FRIEND_DENY = "friendDeny";
public static final String TYPE_FRIEND_REMOVE = "friendRemove";
}
Hope this helps,
Paul :)

Fuseini Isaac
Courses Plus Student 2,225 PointsPaul I am getting a new error after pasting the code. that is on the onclicklistener under the friend type request it the curly bracket right after the onclicklistener.. pls help
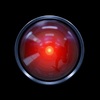
Paul Stevens
4,125 PointsHello,
I will try and help. Can you paste the Activity the error is in and put a // comment next to the line that has the error. Also could you tell me what error you are getting.
Paul :)

Fuseini Isaac
Courses Plus Student 2,225 Pointsthe error is on the last "{" on the commented out line
package com.instantmedia.swerve;
import java.util.ArrayList;
import java.util.List;
import android.app.AlertDialog;
import android.app.AlertDialog.Builder;
import android.content.DialogInterface;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.support.v4.app.ListFragment;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ListView;
import android.widget.Toast;
import android.content.DialogInterface.OnClickListener;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseFile;
import com.parse.ParseObject;
import com.parse.ParseQuery;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import com.parse.SaveCallback;
public class InboxFragment extends ListFragment {
protected List < ParseObject > mMessages;
protected List < ParseUser > mUsers;
protected List < ParseConstants > messageType;
public String senderId;
public String senderName;
protected ParseRelation < ParseUser > mFriendsRelation;
protected ParseUser mCurrentUser;
protected Uri mMediaUri;
protected ArrayList < String > recipientId = new ArrayList < String > ();
@
Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_inbox,
container, false);
return rootView;
}
@
Override
public void onResume() {
super.onResume();
getActivity()
.setProgressBarIndeterminateVisibility(true);
ParseQuery < ParseObject > query = new ParseQuery < ParseObject > (ParseConstants.CLASS_MESSAGES);
query.whereEqualTo(ParseConstants.KEY_RECIPIENT_IDS, ParseUser.getCurrentUser()
.getObjectId());
query.addDescendingOrder(ParseConstants.KEY_CREATED_AT);
query.findInBackground(new FindCallback < ParseObject > () {
@
Override
public void done(List < ParseObject > messages, ParseException e) {
getActivity()
.setProgressBarIndeterminateVisibility(false);
if (e == null) {
//we found messages!
mMessages = messages;
String[] usernames = new String[mMessages.size()];
int i = 0;
for (ParseObject message: mMessages) {
usernames[i] = message.getString(ParseConstants.KEY_SENDER_NAME);
i++;
}
if (getListView()
.getAdapter() == null) {
MessageAdapter adapter = new MessageAdapter(
getListView()
.getContext(),
mMessages);
setListAdapter(adapter);
} else {
//refill the adapter
((MessageAdapter) getListView()
.getAdapter())
.refill(mMessages);
}
}
}
});
}@
Override
public void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
ParseObject message = mMessages.get(position);
String messageType = message.getString(ParseConstants.KEY_FILE_TYPE);
senderName = message.getString(ParseConstants.KEY_SENDER_NAME);
senderId = message.getString(ParseConstants.KEY_SENDER_ID);
String displayMessage = message.getString(ParseConstants.KEY_MESSAGE);
ParseFile file = message.getParseFile(ParseConstants.KEY_FILE);
if (messageType.equals(ParseConstants.TYPE_IMAGE)) {
//view the image
Uri fileUri = Uri.parse(file.getUrl());
Intent intent = new Intent(getActivity(), ViewImageActivity.class);
intent.setData(fileUri);
startActivity(intent);
} else if (messageType.equals(ParseConstants.TYPE_VIDEO)) {
Uri fileUri = Uri.parse(file.getUrl());
//view the video
Intent intent = new Intent(Intent.ACTION_VIEW, fileUri);
intent.setDataAndType(fileUri, "video/*");
startActivity(intent);
} else if (messageType.equals(ParseConstants.TYPE_TEXT)) {
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setTitle("Message from: " + senderName + ".");
builder.setMessage(displayMessage);
builder.setPositiveButton("Ok", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
}
});
AlertDialog dialog = builder.create();
dialog.show();
}
else if (messageType.equals(ParseConstants.TYPE_FRIEND_REQUEST)) {
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setTitle("Friend Request from " + senderName + ".");
builder.setMessage(displayMessage);
//builder.setPositiveButtonButton(R.string.friend_accept, new OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
getActivity().setProgressBarIndeterminateVisibility(true);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.whereEqualTo(senderName, senderId);
query.findInBackground(new FindCallback<ParseUser>() {
public void done(List<ParseUser> users,
ParseException e) {
getActivity().setProgressBarIndeterminateVisibility(false);
if (e == null) {
mFriendsRelation.remove(users.get(0));
mCurrentUser.saveInBackground(new SaveCallback() {
@SuppressWarnings("null")
@Override
public void done(ParseException e) {
if (e != null) {
Log.e("Inbox Fragment",e.getMessage());
}
else {
Log.e("Inbox Fragment", e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage());
builder.setTitle(R.string.error_title);
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
});
}
;
public void onClick1(DialogInterface dialog, int whichButton){
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
getActivity().setProgressBarIndeterminate(true);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.whereEqualTo("objectId", senderId);
query.findInBackground(new FindCallback<ParseUser>() {
public void done (List<ParseUser> users, ParseException e){
getActivity().setProgressBarIndeterminate(false);
if (e == null) {
mFriendsRelation.remove(users.get(0));
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null){
Log.e("Inbox Fragment", e.getMessage());
}else {
Log.e("Inbox Fragment", e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage());
builder.setTitle(R.string.error_title);
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}}
)
;}}
}
);
//send accepted message
String acceptedMessage = mCurrentUser.getUsername() + " has accepted your friend request.";
ParseObject message1 = new ParseObject(ParseConstants.CLASS_MESSAGES);
message1.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser()
.getObjectId());
message1.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser()
.getUsername());
recipientId.add(senderId);
message1.put(ParseConstants.KEY_RECIPIENT_IDS, recipientId);
message1.put(ParseConstants.KEY_MESSAGE, acceptedMessage);
message1.put(ParseConstants.KEY_FILE_TYPE, ParseConstants.TYPE_FRIEND_ACCEPT);
message1.saveInBackground(new SaveCallback() {@
Override
public void done(ParseException e) {
if (e == null) {
//success
//Tell the user that the new friend was added.
Toast.makeText(getActivity(), senderName + " is now your friend.", Toast.LENGTH_LONG)
.show();
} else {
String errorMessage = e.getMessage();
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage("Error accepting friend request." + errorMessage);
builder.setTitle(R.string.error_selecting_file_title);
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setNegativeButton("Deny", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
//Refuse request
Toast.makeText(getActivity(), "Request Denied.", Toast.LENGTH_LONG)
.show();
//Send denied message.
mCurrentUser = ParseUser.getCurrentUser();
String acceptedMessage = mCurrentUser.getUsername() + " has denied your friend request.";
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser()
.getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser()
.getUsername());
recipientId.add(senderId);
message.put(ParseConstants.KEY_RECIPIENT_IDS, recipientId);
message.put(ParseConstants.KEY_MESSAGE, acceptedMessage);
message.put(ParseConstants.KEY_FILE_TYPE, ParseConstants.TYPE_FRIEND_DENY);
message.saveInBackground(new SaveCallback() {@
Override
public void done(ParseException e) {
if (e == null) {
//success
//Tell the user that the new friend was added.
Toast.makeText(getActivity(), "Request denied.", Toast.LENGTH_LONG)
.show();
} else {
String errorMessage = e.getMessage();
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage("Error denying friend request." + errorMessage);
builder.setTitle(R.string.error_selecting_file_title);
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
});
if (messageType.equals(ParseConstants.TYPE_FRIEND_ACCEPT)) {
//Display dialog showing that friend has accepted.
AlertDialog.Builder builder1 = new AlertDialog.Builder(getActivity());
builder1.setMessage(senderName + " is now your friend.");
builder1.setTitle("Friend Request.");
builder1.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog1 = builder1.create();
dialog1.show();
//Add new friends name to relation list
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
getActivity()
.setProgressBarIndeterminate(true);
ParseQuery < ParseUser > query1 = ParseUser.getQuery();
query1.whereEqualTo("objectId", senderId);
query1.findInBackground(new FindCallback < ParseUser > () {@
Override
public void done(List < ParseUser > users, ParseException e) {
getActivity()
.setProgressBarIndeterminate(false);
if (e == null) {
mFriendsRelation.add(users.get(0));
mCurrentUser.saveInBackground(new SaveCallback() {@
Override
public void done(ParseException e) {
if (e != null) {
Log.e("Inbox Fragment", e.getMessage());
}
}
});
} else {
Log.e("Inbox Fragment", e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
} else if (messageType.equals(ParseConstants.TYPE_FRIEND_DENY)) {
//Display dialog showing that friend has denied.
AlertDialog.Builder builder1 = new AlertDialog.Builder(getActivity());
builder1.setMessage(senderName + " has denied the friend request.");
builder1.setTitle("Friend Request.");
builder1.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog1 = builder1.create();
dialog1.show();
} else if (messageType.equals(ParseConstants.TYPE_FRIEND_REMOVE)) {
//Display dialog showing that a friend has requested removal.
AlertDialog.Builder builder1 = new AlertDialog.Builder(getActivity());
builder1.setMessage(senderName + " has been removed from your friends list.");
builder1.setTitle("Friend Update.");
builder1.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog1 = builder1.create();
dialog1.show();
//Remove friends name from relation list
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
getActivity()
.setProgressBarIndeterminate(true);
ParseQuery < ParseUser > query1 = ParseUser.getQuery();
query1.whereEqualTo("objectId", senderId);
query1.findInBackground(new FindCallback < ParseUser > () {@
Override
public void done(List < ParseUser > users, ParseException e) {
getActivity()
.setProgressBarIndeterminate(false);
if (e == null) {
mFriendsRelation.remove(users.get(0));
mCurrentUser.saveInBackground(new SaveCallback() {@
Override
public void done(ParseException e) {
if (e != null) {
Log.e("Inbox Fragment", e.getMessage());
}
}
});
} else {
Log.e("Inbox Fragment", e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
//delete the message
List < String > ids = message1.getList(ParseConstants.KEY_RECIPIENT_IDS);
if (ids.size() == 1) {
//last recipient, delete the message
message1.deleteInBackground();
} else {
//remove recipients name
ids.remove(ParseUser.getCurrentUser()
.getObjectId());
ArrayList < String > idsToRemove = new ArrayList < String > ();
idsToRemove.add(ParseUser.getCurrentUser()
.getObjectId());
message1.removeAll(ParseConstants.KEY_RECIPIENT_IDS, idsToRemove);
message1.saveInBackground();
}
};
}
```
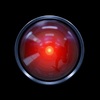
Paul Stevens
4,125 PointsHello,
Your line of code there looks different to mine. Mine looks like this:
builder.setPositiveButton("Ok", new DialogInterface.OnClickListener() {
I have the extra bit about DialogInterface and am not using a string resource. The string resource shouldn't be the problem. Can you tell me the error message you are getting?
Paul :)

Fuseini Isaac
Courses Plus Student 2,225 Pointsthe last "{" is just underlined red. i tried to update parse and all these errors started.
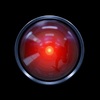
Paul Stevens
4,125 PointsHello,
This piece of code seems to be put in the middle of the code that deals with Accepting or Denying friend requests.
public void onClick1(DialogInterface dialog, int whichButton){
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
getActivity().setProgressBarIndeterminate(true);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.whereEqualTo("objectId", senderId);
query.findInBackground(new FindCallback<ParseUser>() {
public void done (List<ParseUser> users, ParseException e){
getActivity().setProgressBarIndeterminate(false);
if (e == null) {
mFriendsRelation.remove(users.get(0));
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null){
Log.e("Inbox Fragment", e.getMessage());
}else {
Log.e("Inbox Fragment", e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage());
builder.setTitle(R.string.error_title);
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}}
)
;}}
}
);
This is my code from the check if it is a Friend Request to the bit that sends the accepted message:
}else if (messageType.equals(ParseConstants.TYPE_FRIEND_REQUEST)){
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setTitle("Friend Request from " + senderName + ".");
builder.setMessage(displayMessage);
builder.setPositiveButton("Accept", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
//Accept request
//Add senders id to friends list
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDSRELATION);
getActivity().setProgressBarIndeterminate(true);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.whereEqualTo("objectId", senderId);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
getActivity().setProgressBarIndeterminate(false);
if (e == null){
mFriendsRelation.add(users.get(0));
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null) {
Log.e("Inbox Fragment", e.getMessage());
}
}
});
}else {
Log.e("Inbox Fragment", e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
//YOUR ONCLICK1 CODE IS HERE.
//send accepted message
I also notice that you have a lot more curly brackets than I do. I'm not sure if that onClick1 method is getting in the way, but I am pretty sure that the problem is that the brackets are not balancing out.
I would try commenting out the lines of code that do things and leave myself with the basic structure of the code. Make sure the structure is causing no errors.
Not much of an answer, sorry.
Paul :)

Fuseini Isaac
Courses Plus Student 2,225 PointsWell do you think it has anything to do with my update of eclipse?
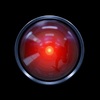
Paul Stevens
4,125 PointsHello,
I'm not sure. I don't think so, but you did say some errors appeared after you updated Eclipse. Maybe save the project files, and try reinstalling the previous version of Eclipse. Not sure.
Paul :)