Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial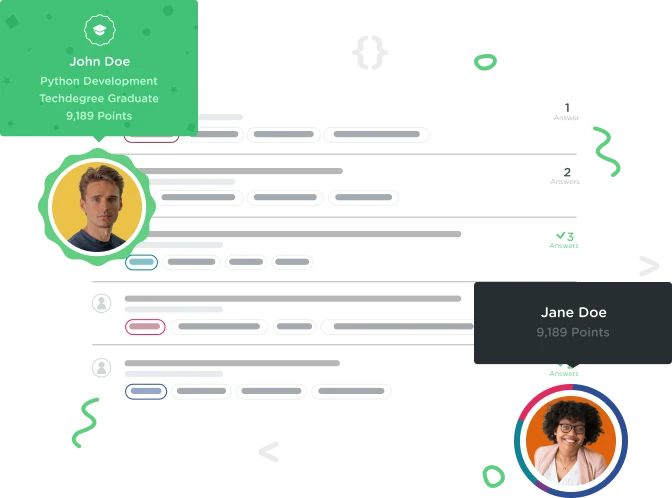
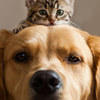
Devin Scheu
66,191 PointsAnother Java Code Question
Question: The code in Main.java is going to throw an IllegalArgumentException when the drive method is called. Catch it and warn the user
Code:
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(100);
} catch (IllegalArgumentException iae) {
System.out.println("Alert");
System.out.printf("There was an error: %s\n", iae.getMessage());
}
kart.drive(2);
}
}
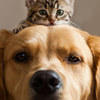
Devin Scheu
66,191 PointsPosted It

Stone Preston
42,016 PointsI recategorized your question as Java, not Javascript
2 Answers

Stone Preston
42,016 Pointsthe original code is:
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
kart.drive(2);
}
}
the drive method should only be called once. remove kart.drive(2) from the end of your code.
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(100);
} catch (IllegalArgumentException iae) {
System.out.println("Alert");
System.out.printf("There was an error: %s\n", iae.getMessage());
}
}
}
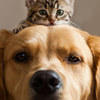
Devin Scheu
66,191 PointsThanks again Stone, sorry for the trouble.

Stone Preston
42,016 Pointsno worries. was not any trouble at all

SZE SZE XU
9,759 Pointshi whats iae mean in this case and whats println ?

Jeff Collins
24,709 PointsCraig explains what iae is at around 3:55 in the exceptions video. It's an abbreviation for Illegal Argument Exception.
Stone Preston
42,016 PointsStone Preston
42,016 Pointswhat is the question