Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial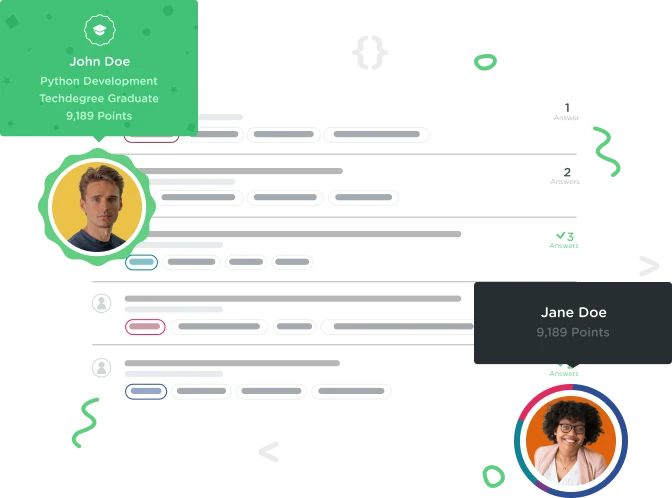
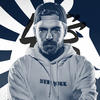
Kevin Haube
12,265 PointsApp Crash on Push Test?
Here's my error:
10-08 15:14:50.751 5984-5984/com.example.kevin.ribbit E/AndroidRuntime﹕ FATAL EXCEPTION: main
Process: com.example.kevin.ribbit, PID: 5984
java.lang.RuntimeException: Unable to start receiver com.parse.ParsePushBroadcastReceiver: java.lang.NullPointerException
at android.app.ActivityThread.handleReceiver(ActivityThread.java:2567)
at android.app.ActivityThread.access$1800(ActivityThread.java:161)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1341)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:157)
at android.app.ActivityThread.main(ActivityThread.java:5356)
at java.lang.reflect.Method.invokeNative(Native Method)
at java.lang.reflect.Method.invoke(Method.java:515)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:1265)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:1081)
at dalvik.system.NativeStart.main(Native Method)
Caused by: java.lang.NullPointerException
at com.parse.ParsePushBroadcastReceiver.getSmallIconId(ParsePushBroadcastReceiver.java:253)
at com.parse.ParsePushBroadcastReceiver.getNotification(ParsePushBroadcastReceiver.java:335)
at com.parse.ParsePushBroadcastReceiver.onPushReceive(ParsePushBroadcastReceiver.java:143)
at com.parse.ParsePushBroadcastReceiver.onReceive(ParsePushBroadcastReceiver.java:104)
at android.app.ActivityThread.handleReceiver(ActivityThread.java:2552)
at android.app.ActivityThread.access$1800(ActivityThread.java:161)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1341)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:157)
at android.app.ActivityThread.main(ActivityThread.java:5356)
at java.lang.reflect.Method.invokeNative(Native Method)
at java.lang.reflect.Method.invoke(Method.java:515)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:1265)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:1081)
at dalvik.system.NativeStart.main(Native Method)
... and here's my Application class ...
package com.example.kevin.ribbit;
import android.app.Application;
import com.example.kevin.ribbit.ui.MainActivity;
import com.parse.Parse;
import com.parse.ParseInstallation;
import com.parse.PushService;
import com.parse.ParseAnalytics;
/**
* Created by Kevin on 9/13/2014.
*/
public class RibbitApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
Parse.initialize(this,
"okogqsYV6QQBCfrRL5AoXhIDyf2oF3cETc27NKXt",
"tBNDXSecUxoZYUmGI36vqZsybiJxZGqMnmXhiYRo"
);
ParseInstallation.getCurrentInstallation().saveInBackground();
}
}
I honestly have no clue what it could be. Everything in the manifest is set correctly, and to match the package name of my app. It's not registering my device as a reciever for push notifications on Parse, as it doesn't show up when i try to send one via Parse.
4 Answers

Daniel Schmidt
1,683 PointsYou can also use Parse 1.7. There are just a few changes you need to make.
First, to fix the error directly caused by issuing the push from the Parse backend you need to declare a notification icon for the Parse push in your Manifest. For the ribbit application, add
<meta-data android:name="com.parse.push.notification_icon"
android:resource="@drawable/ic_launcher"/>
just before the closing application-Tag.
Issuing another push from the backend now will give you a push notification as you expect.
So far so good. Clicking the push will result in an app crash again. To fix this you need to remove the now deprecated call PushService.setDefaultPushCallback(...)
and add your own Receiver class.
I did this in the *.util package just as the following:
public class Receiver extends ParsePushBroadcastReceiver {
@Override
public void onPushOpen(Context context, Intent intent) {
Intent i = new Intent(context, MainActivity.class);
i.putExtras(intent.getExtras());
i.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
context.startActivity(i);
}
}
Next, change the default Parse receiver to the one just created:
Go to your Manifest file.
Locate the
<receiver android:name="com.parse.ParsePushBroadcastReceiver">
replace the whole
<receiver android:name="com.parse.ParsePushBroadcastReceiver"> ... </receiver>
- Tag with
<receiver
android:name="your.package.name.utils.Receiver"
android:exported="false" >
<intent-filter>
<action android:name="com.parse.push.intent.RECEIVE" />
<action android:name="com.parse.push.intent.DELETE" />
<action android:name="com.parse.push.intent.OPEN" />
</intent-filter>
</receiver>
Now a click on the Push notification leads to your inbox as intended. Hope this helps.
SO pointed me at this. See original post here.
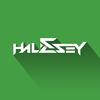
Liam Hales
356 PointsIn Parse 1.8.0 I don't get the error anymore when clicking the notification to bring me to my inbox and i am still using the deprecated call PushService.setDefaultPushCallback(...). Maybe its worth a try updating Parse to 1.8.0 as the above answer doesn't give me the notification rotate animation in the notification bar for some reason, still a very good answer tho Daniel Schmidt!
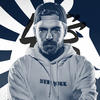
Kevin Haube
12,265 PointsSimply put, Parse 1.7.0 doesn't work with Push. At least not without major changes in code. I reverted back to Parse 1.5.1 and it's fine.

Kevin Nguyen
77 PointsHow did you got back to 1.5.1?

haunguyen
14,985 Points@ stephen fontein
Leave the line PushService.setDefaultPushCallback(this, MainActivity.class);
as is, don't delete it, it was probably a mistake in the instruction.
The line android:name="com.spencerfontein.ueat.Receiver"
is just the path to your Receiver class file.
If you created the Receiver file and put it inside the "com.spencerfontein.ueat.utils" package, then the location of the file is android:name="com.spencerfontein.ueat.utils.Receiver"
, if you put the file inside the root package, then your original code is correct.
Kevin Haube
12,265 PointsKevin Haube
12,265 PointsI'll give it a try!
John Corser
5,660 PointsJohn Corser
5,660 PointsAwesome, works great! It was unclear in your post though that you have to delete the entire
<receiver android:name="com.parse.ParsePushBroadcastReceiver">...</receiver>
block and replace it with the new one.Daniel Schmidt
1,683 PointsDaniel Schmidt
1,683 PointsGlad it helped! - Edited the answer to make it more clear, that the whole Tag has to be replaced!:)
Liam Hales
356 PointsLiam Hales
356 PointsThanks dude this really helped me out! :)
Harry James
14,780 PointsHarry James
14,780 PointsBen Jakuben - I recommend you add this answer to the Teacher's Notes as, I can understand a lot of people will have this problem when using the latest version of Parse :)
Also, thanks Daniel Schmidt for the solution! I have set this as the Best Answer for this post instead as, it has helped out more users (Proven by the number of ratings!).
Shariq Shaikh
13,945 PointsShariq Shaikh
13,945 Pointsthe last video of the course uses the out dated code that your method has us discard, could you provide a work around for adding a new Push icon?
stephen fontein
605 Pointsstephen fontein
605 PointsDaniel Schmidt Hey thanks for the code, I am having trouble getting it working, I am using Parse 1.7.1 but I don't think that is the problem I made a new java class Reciver and put what you have in it and deleted the PushService.setDefaultPushCallback(...) line from MyApplication.java class
And then this is what I have in my androidManifest.xml
<service android:name="com.parse.PushService" /> <receiver android:name="com.parse.ParseBroadcastReceiver"> <intent-filter> <action android:name="android.intent.action.BOOT_COMPLETED" /> <action android:name="android.intent.action.USER_PRESENT" /> </intent-filter> </receiver> <receiver android:name="com.spencerfontein.ueat.Receiver" android:exported="false" > <intent-filter> <action android:name="com.parse.push.intent.RECEIVE" /> <action android:name="com.parse.push.intent.DELETE" /> <action android:name="com.parse.push.intent.OPEN" /> </intent-filter> </receiver> <receiver android:name="com.parse.GcmBroadcastReceiver" android:permission="com.google.android.c2dm.permission.SEND"> <intent-filter> <action android:name="com.google.android.c2dm.intent.RECEIVE" /> <action android:name="com.google.android.c2dm.intent.REGISTRATION" />
But when I send pushes not I don't receive them do I need to add something back to MyApplicaiton. java?
If I add this line back to MyApplication.java it works?
"PushService.setDefaultPushCallback(this, MainActivity.class);"
But you said to delete this line?
I also wasn't sure what you meant by "add your own Receiver class. I did this in the *.util" where is *.util?
Thanks for your help :)
Harry James Liam Hales Ben Jakuben Kevin Haube
Sam McDavid
20,242 PointsSam McDavid
20,242 PointsThis same method also works with Parse 1.8.0. However, I did have to go to the installations table and remove all of the records and uninstall the app from the device before this method worked.
Figured I would share since I imagine some other developers may have issues as well.
Good Luck!
Kevin Nguyen
77 PointsKevin Nguyen
77 Points<meta-data android:name="com.parse.push.notification_icon" android:resource="@drawable/ic_launcher"/>
I added that line to my file..when I start it back up again it does not even send me a push like you described
Help! Harry James Daniel Schmidt
Harry James
14,780 PointsHarry James
14,780 PointsHey Kevin Nguyen!
It looks like you've missed out the
<meta-data
tag from the code above - go ahead and add this in and continue with the steps Daniel has discussed here and it should fix your problem.If you have any more problems though, give me a shout :)
Jacob Bergdahl
29,119 PointsJacob Bergdahl
29,119 PointsThis is an amazing reply, however I just want to note that those who don't want to do all of this don't have to! This merely sets a test push notification. In later videos on this course, Ben will walk over steps on how to make push notifications in the manner that they are to be used with the app, and none of this is necessary for those steps.
Kevin Hackett
10,441 PointsKevin Hackett
10,441 PointsThanks Daniel. Excellent guide :) Still works with Parse 1.10.1.jar