Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial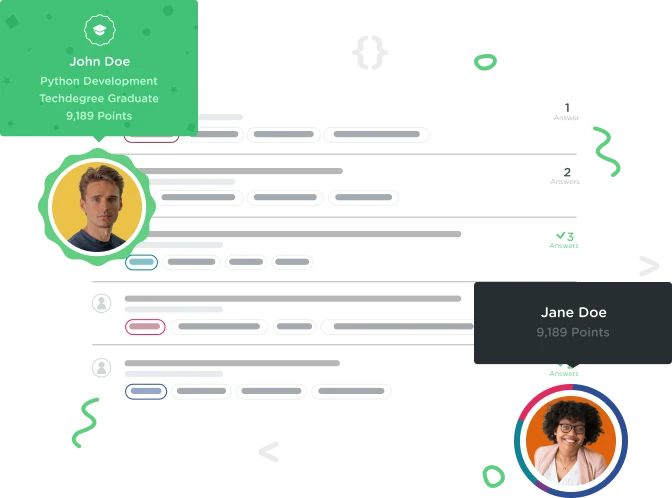
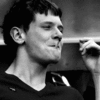
Derek Dawes
4,944 Pointsarray_walk($names, 'print_info'); question regarding $value, $key
I understand (for the most part) what the function is doing. My question is:
How did he come up with the variables in the function argument? He states function print_info($value, $key){}
Where did $value and $key come from? I assume its the way array_walk was written when created by the PHP_Gods.
I don't see it declared anywhere so how does the computer know its the actual array value and key?
Keys come first in arrays then values I assume; just doesn't make sense to me. Heres the entire code in workspace (nothing omitted). I think I am confused on how variables and functions work. Feels like every variable needs to be declared and have an assignment to it.
<?php
$names = array('Mike' => 'Frog',
'Chris' => 'Teacher',
'Hampton' => 'Teacher'
);
function print_info($value, $key){
echo $key . " is a " . $value . ".<br>";
}
array_walk($names, 'print_info');
?>```
4 Answers

Petros Sordinas
16,181 PointsYes, you could use $dog and $cat if you wanted (or any other name). You see $key and $value being used because they are the variable names that make sense.
You can pass any variable name in any function, same thing here.
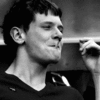
Derek Dawes
4,944 PointsThank you that makes sense of the whole thing.

Petros Sordinas
16,181 PointsDerek,
Have a look at the php documentation: http://php.net/manual/en/function.array-walk.php
You could replace array_walk with the following:
foreach ($names as $key => $value)
{
print_info($value, $key);
}
This is what array_walk does; it accepts an array as input and then passes the $value and $key (in that order) to the callback function that you define (print_info).
I hope this makes sense
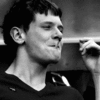
Derek Dawes
4,944 PointsIs $value and $key set in php? Or was it made up for easier understanding? Could I use $dog $cat if I really wanted is what I am meaning? Thank you

Niels Klom
6,134 PointsGreat explanation! If i'm right it like array walk has 1 and 2, and it will automatically assign 1 to the first and 2 to the second argumant.

Gavin Ralston
28,770 PointsThe function you define can have absolutely any variable names you want. They're for you to use so they make your code make sense.
array_walk is going to hand to your function (in this order) a key and a value from an associative array.
So say you wanted to array_walk your list of dogs and their type of breeds:
<?php
$dogs = array(
'Chopper' => 'Chihuahua',
'Bear' => 'Chow',
'Superduper' => 'Jack Russell'
);
function puppy_info($breed, $dog) {
echo "$dog is a $breed<br>";
}
array_walk($dogs, 'puppy_info');
);
The names you put in that function you created (puppy_info) are only there for you. They're just labels you assign to the values passed in so you can work with them.

Christopher Mayfield
19,928 PointsSo the order doesn't matter in the argument list?
If you were to put
function puppy_info($breed, $dog) {
echo "$dog is a $breed<br>";
}
or
function puppy_info($dog, $breed) {
echo "$dog is a $breed<br>";
}
it doesn't matter?

Gavin Ralston
28,770 PointsIt won't matter how you define it, but it will matter how you pass those strings in.
Keep in mind that the only thing you're doing in the function declaration is making variable names you can use inside the function, preferably so you can make sense of the code inside of it.
There won't be anything stopping you from passing in dog and breed (in that order) just because you set up the arguments expecting breed and then dog.
The good news is anyone looking at your function definition's signature (the first line, including parameters) will know exactly what you're expecting.
bea ta
2,641 Pointsbea ta
2,641 PointsHello everyone, This is my code, <?php
$name = array ( 'Mike' => 'frog', 'Adrian' => 'teacher', 'Beata'=>'cook' );
function print_info( $value, $key){
echo "key is a $value ";
}
array_walk($name, 'print_info'); ?>
and I get this message: key is a frog, key is a cook, key is a teacher
???????????????????