Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial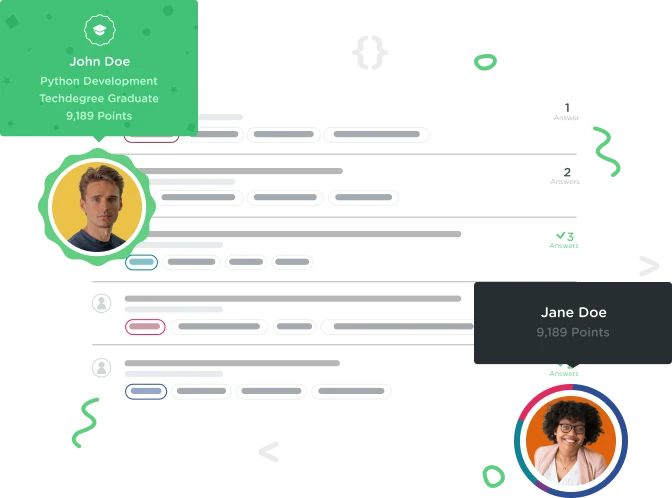
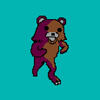
Tanner Brandt
12,044 Pointsattr_accessible missing in Rails 4 - Here's the workaround for the Treebook tutorial.
If you are following along in the tutorial, you might run into the problem of missing attr_accessible. This means you are using Rails 4, which no longer uses attr_accessible within the model to pass along data into a database, and instead uses Strong Parameters within the Controller.
If anyone runs into this problem and wants to continue using Rails 4 for this tutorial (instead of reverting back to Rails 3), here is a quick 5 minute workaround, so that you can still use Devise and add extra fields to your forms using Strong Parameters instead of attr_accessible.
1:
Ignore everything in this Treebook tutorial that has to do with attr_accessible. You can ignore all of those steps until you reach the 'Customizing Forms' section of this Treebook tutorial.
2:
Once you reach the 'Customizing Forms' section of this Treebook tutorial, open this tutorial: http://www.jacopretorius.net/2014/03/adding-custom-fields-to-your-devise-user-model-in-rails-4.html
3:
Skip ahead in this new tutorial to "Customizing the RegistrationsController," because we already did the first few steps before reaching the 'Customizing Forms' section in the Treebook tutorial.
4:
Following along in this new short tutorial, go to Sublime Text and open up the controllers folder, where you should have application_controller.rb and statuses_controller.rb. Create a new ruby file below these controllers in the controllers folder, calling it: registrations_controller.rb
5:
Copy and paste the following into the registrations_controller.rb file that you created:
class RegistrationsController < Devise::RegistrationsController
private
def sign_up_params params.require(:user).permit(:first_name, :last_name, :profile_name, :email, :password, :password_confirmation)
end
def account_update_params params.require(:user).permit( :email, :password, :password_confirmation, :current_password)
end
end
6:
Then, go to the config folder in Sublime text and open the routes.rb file. Delete the
devise_for :users
line and replace it with the following:
devise_for :users, :controllers => { registrations: 'registrations' }
7:
Then, go to your statuses_controller.rb file and, at the very bottom, find this def:
def status_params
params.require(:status).permit(:name, :content)
end
and change it to this:
def status_params
params.require(:status).permit(:user_id, :content)
end
You are all set! All of your parameters within your form should now be passed to the database when a new user signs up. You can continue forward in the Treebook tutorial.
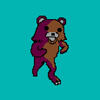
Tanner Brandt
12,044 PointsOh, right! I'll reword the title of this post to avoid confusion.
11 Answers
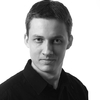
Maciej Czuchnowski
36,441 PointsThat's a very good entry, Tanner, and Treehouse team should add something like this to the treebook course, because I regularny have to explain those things to people who are not aware of differences between Rails 3 and 4 (I was SO frustrated the first time I encountered this problem...). There's also another way to do this thing using one initializer file, I described it here:
https://teamtreehouse.com/forum/validates-firstname-presence-true-not-working
Unfortunately these entries will sooner or later get lost in time like tears in the rain (time to die), so our mission is to do this regularly ;). Join me in my quest!
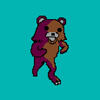
Tanner Brandt
12,044 PointsThanks Maciej! It was so frustrating to find the translation that would work for this app. And I agree... I wish Treehouse would create an id for every video they post, and link it to questions that users generate in the forum so that we can figure these problems out much more quickly.

Alexander Lee
1,420 Pointsi followed this tutorial but have a couple of issues...
in the show.html.erb
<p> <strong>Name:</strong> <%= @status.user_id%> </p>
works and generates the id #
but when I try to do @status.user_id.first_name
I get a big no-no error
on a side note:
when I refresh my home page my hover works but when I push back from the edit page the edit/delete buttons disappear!
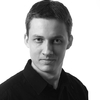
Maciej Czuchnowski
36,441 PointsShouldn't it be @status.user.first_name ?
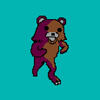
Tanner Brandt
12,044 PointsYeah, fixing the typo should fix your first problem -- the way you have written it is trying to call an attribute from another attribute in a way that rails doesn't understand. Instead, you want to call status.user.first_name: [@status (your status variable) >> .user (which connects to the user record associated with that variable within the user table) >> .first_name (the attribute you are hoping to call here from the user record).
As for your second problem... I'm not quite sure, but when I have this problem it is usually related to turbolinks in rails 4. Go to the 'layout' for the specific page causing you trouble within your views folder, and in the first body tag, change it to this instead:
<body data-no-turbolink="true">
That will disable turbolinks, see if it helps with your hover issue when going back a page.
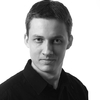
Maciej Czuchnowski
36,441 PointsYeah, this is most probably turbolinks. Something like this in your coffeescript should help:
http://stackoverflow.com/questions/18770517/rails-4-how-to-use-document-ready-with-turbo-links
Turbolinks fire up a different event than simple page ready, that is why they require special treatment.

Alexander Lee
1,420 PointsThank you :)

Andrew Hunt
357 PointsHi guys -
Followed these steps but still getting an error. I've tripled checked and all the associations/code that you provided is exactly correct.
I noticed that when I do:
<%= status.user %>
I don't get an error. It's only when I do
<%= status.user/first_name %> (or full_name with the method)
That I'm getting the error. Do you have any idea why?
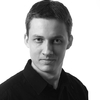
Maciej Czuchnowski
36,441 PointsDid you make sure that you don't have any old entries in your database that have nil values under names?

Andrew Hunt
357 PointsAnd sorry, that's a <%= status.user.first_name %> ha, just a typo
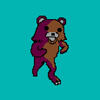
Tanner Brandt
12,044 PointsHi Andrew,
Does this error occur within the show.html.erb file? The following is my code (for retrieving a full_name with the status).
<p>
<b>Name:</b>
<%=@status.user.full_name %>
</p>
You might not be making the status variable an instance variable in this case, if the code you provided above is the code in your program... but I'm not entirely sure that's the problem if it works fine when <%= status.user %> is working and the only difference is trying to call the .full_name...
Let me know if that was the problem or if you still can't get it to work.

Andrew Hunt
357 PointsThanks for the rapid reply,
I was using it in the index file, not the show, so not an instance variable
If you don't know it's no worries and I'll head over to codementor.io
Thanks!

Andrew Hunt
357 PointsI did! I did User.delete_all and Status.delete_all, still didn't work!
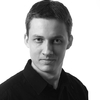
Maciej Czuchnowski
36,441 PointsOK, please publish your project on github and link it here, I'd love to take a look if it's OK :)

Andrew Hunt
357 PointsWill do! I am speaking to an expert tonight and will see if I can review
Best, Andy

Miguel Hernández
Courses Plus Student 1,101 PointsThank you very much for this tutorial. It works perfect!!! :D

mamat golo
Courses Plus Student 3,755 PointsCould anyone please push to github for this solution. I tried for several times but it just doesn't work.
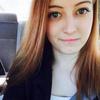
Anna K
17,115 PointsThank you so much for this! I was going to give up the application entirely.
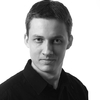
Maciej Czuchnowski
36,441 PointsThere's still a lot that will go wrong along the way because of the differences between the gems used in the tutorial and their current versions, so make sure you post your questions on the forum and never give up ;)

Bjorn Bakker
1,134 PointsThe tutorial is great, however it doesn't seem to work for me. I keep getting the same error:
NoMethodError in Statuses#new app/views/statuses/_form.html.erb where line #15 raised:
Extracted source (around line #15): <div class="field"> <%= f.label :name %><br /> <%= f.text_field :name %> #this is where it keeps pointing to. </div> <div class="field"> <%= f.label :content %><br />
I checked the multiple tutorials, deleted all previous statuses, and can't seem to find it.
It's probably one of those easy things a rookie like me keeps overlooking :P.
Thanks for the help in advance.
Björn
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsTechnically, attr_accessible still exists and is supported (it's a Ruby thing), it just doesn't enable you to write things into the database ;)