Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial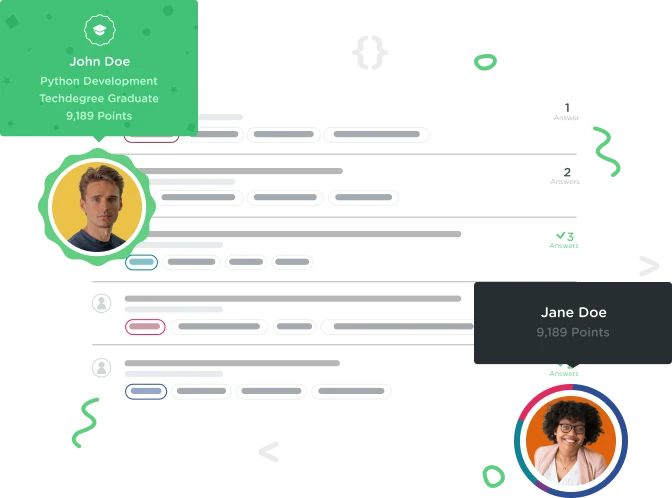

cathymacars
Full Stack JavaScript Techdegree Student 15,941 PointsBuild a Simple PHP Application > Wrapping Up The Project > Objects: Task 5/7
Hello, I'm having trouble with the Code Challenge mentioned in the title.
The question is: "PalprimeChecker objects have a method called isPalprime(). This method does not receive any arguments. It returns true if the number property contains a palprime, and it returns false if the number property does not contain a palprime. (Tip: 17 is not a palprime.) Turn the second echo statement into a conditional that displays “is” or “is not” appropriately. The conditional should call the isPalprime method of the $checker object. If the isPalprime method returns true, then echo “is”; otherwise, echo “is not”."
My answer was:
<?php
include("class.palprimechecker.php");
$checker = new PalprimeChecker;
$checker->number = 17;
$number = $checker->number;
echo "The number " .$number. " ";
if(isPalprime($number)){
echo "is";
} else {
echo "is not";
}
echo " a palprime.";
?>
The error I'm getting: "PHP Fatal error: Call to undefined function isPalprime() in palprimes.php on line 15 The number 17 "
Help?
9 Answers

Randy Hoyt
Treehouse Guest TeacherSo close! The problem is in this line:
if(isPalprime($number)){
Two things:
- You are calling isPalprime as if it is general function. It's not: it's a method of all PalprimeChecker objects. (Hint: You'll need to use the two characters that together look like a single arrow.)
- The question states that the isPalprime method does not receive any arguments, but you are passing in one argument. (It will know to check if 17 is a palprime when you call the isPalprime method because you set it as a property of the object.)
Does that help?

Steven Walters
10,573 PointsThis quiz makes me appreciate JavaScript.

Michael Dion
6,232 PointsGlad I'm not the only one who struggled here. These challenges are definitely forcing me to have to (somewhat) understand what's going on instead of just copying what's in the video :-).

cathymacars
Full Stack JavaScript Techdegree Student 15,941 PointsSo, yeah, I noticed that it didn't receive any arguments. But since I tried all kinds of combinations like
if(isPalprime->$number))
or
if(isPalprime()->$checker ))
And nothing worked... I tried using it like a function, just to be sure... But it wasn't until you said "because you set it as a property of the object" that I understood! So I tried:
if($checker->isPalprime())
And it worked. Thanks! :D
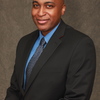
Bryan Knight
34,215 PointsI was SO stuck here as well. I couldn't figure it out to save my life. I read through this post three times and then it clicked. I'll definitely have to spend more time working with objects, methods and properties to make sure I have this nailed down.

Mauro Bonucci
5,953 PointsI was stucked to in this one, but what was wrong in mine was the conditional, instead puttin "==" i was puttin "="
```echo "The number" . $checker->number; if(!$checker -> isPalprime() == $checker->number){
echo "is not";} echo " a palprime.";```

KJ Prince
5,145 PointsIt's unnecessary to use the ! operator in this question. You're checking if it is not equal to instead of just checking whether the number is palinprime or not using he conditional if statement to do the work.
here's what worked for me:
<?php
include ("class.palprimechecker.php"); $checker = new PalprimeChecker; $checker->number = 17;
echo "The number " . $checker->number; if ( $checker->isPalprime() ) { echo "is"; } else { echo "is not"; }
echo " a palprime.";
?>
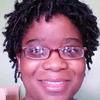
Martina Carrington
15,754 Pointsi am really having problem with Challenge Task 7 of 7
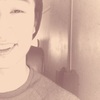
Jason S
16,247 Pointsjust put 151 where 16661 is

Stephen Garner
Courses Plus Student 11,241 PointsIf anyone is having trouble...my advice is to complete the first time, and then do it a second time. New concepts that you have a difficult time understanding must be practiced...
I have re-completed quizzes and assignments because I had difficulty completing them. Some people learn there repetition.
$checker->isPalprime()
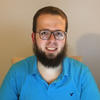
Micah Doulos
17,663 PointsThe important thing to remember is that isPalprime() is not a global function. If you think of it is as a function, it would be a function contained within or inside the object called $checker. So it does not make sense to try to call isPalprime($checker). The isPalprime method is itself subject to the $checker object and thus must be called inside of the object, not vice-versa.