Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial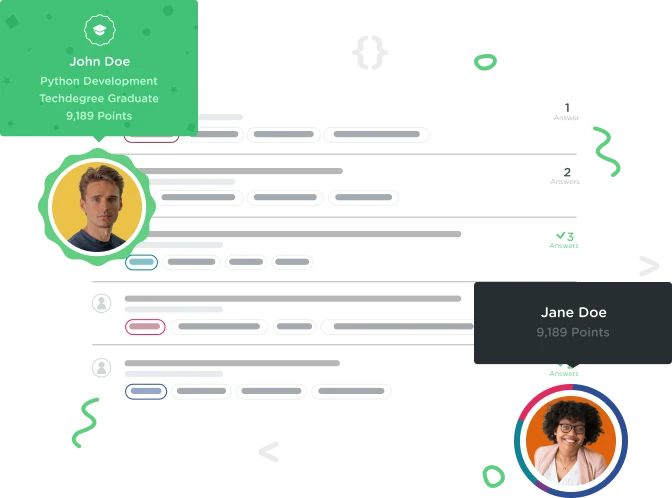

Matt James
1,948 PointsBummer function not defined? Return values please help
function arrayCounter(array) { return array.length; }else{ array.length === 'string', 'number' , 'undefined'; return 0; }
4 Answers
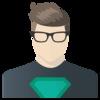
Richard Duncan
5,568 PointsYou need to use the typeof method with an if statement to detect the type of the value passed into the arrayCounter function. If the type of the value matches a string, number or is undefined then return 0, else return the array length.
I've produced an example here you will need to click on the "Run" button on the console window to see the result but you can change the t1 value in the function call to t2, t3, t4 to see the behaviour when a string, number and undefined value are passed into the array.
Here's the code from that example. Hope this helps.
var t1 = [1, 2, 3];
var t2 = "a string";
var t3 = 7;
var t4;
function arrayCounter( myArr ) {
if( typeof myArr === 'string' || typeof myArr === 'number' || typeof myArr === 'undefined') {
return 0;
} else {
return myArr.length;
}
}
console.log( arrayCounter( t1 ) );
You can read more about typeof here.

Matt James
1,948 PointsThanks for getting back to me Richard...the question was - Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.
I must admit the questions are getting harder and not fully explained in the Video before

Matt James
1,948 PointsMany thanks for your time on this Richard. This is getting complicated. I noticed you have used the " ||" character. I haven't come across this in the tutorials. What is the syntax here? what does the || do in javaScript
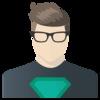
Richard Duncan
5,568 PointsIt's the logical operator for or so if myArr is a string that would be all she wrote, if it's not a string it would look at the next condition after the double pipes for number and so on.
You can achieve the same thing in longhand as so: -
if (typeof myArr === 'string') {
return 0;
} else if (typeof myArr === 'number') {
return 0;
} else if (typeof myArr === 'undefined') {
return 0;
} else {
return myArr.length;
But see how I am repeating myself with each condition and each return 0? it's good practice to think DRY Dont Repeat Yourself when programming.

Matt James
1,948 PointsExcellent explanation. thank you.
Matt
Richard Duncan
5,568 PointsRichard Duncan
5,568 PointsCan you post the challenge task? You seem to have combined a function with an if statement and a whole load of other issues.