Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial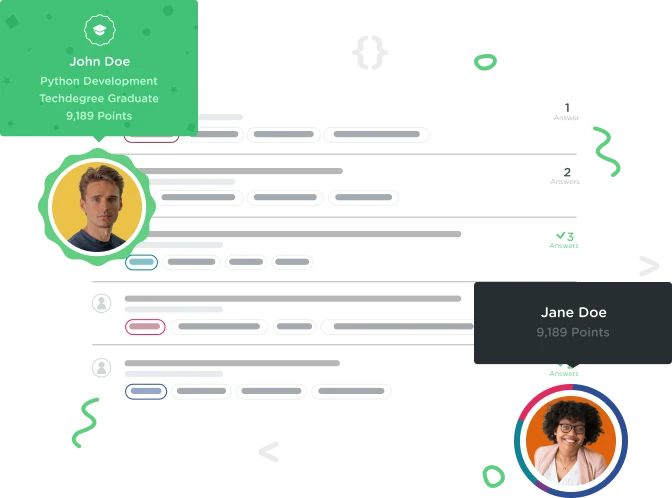
Timothy Baker
2,969 PointsChallenge Part I Managing Playlist Data. Assigning profilePictureName.
Can't figure out Part I. Declared NSString *profileImageName and assigned the key @"profilePicture" but something must be off and I don't know what to fix. Help?
#import <Foundation/Foundation.h>
@interface Treehouse : NSObject
@property (strong, nonatomic) NSDictionary *friends;
@end
#import "Treehouse.h"
@implementation Treehouse
- (instancetype)init
{
self = [super init];
if (self) {
_friends = @{@"firstName": @"Susan",
@"lastName": @"Olson",
@"profilePicture": @"susan_profile.png"
};
}
return self;
}
@end
#import <Foundation/Foundation.h>
@interface User : NSObject
@property (nonatomic, strong) NSString *firstName;
@property (nonatomic, strong) NSString *lastName;
@property (nonatomic, strong) NSString *profileImageName;
@property (nonatomic, strong) UIImage * profileImage;
@property (nonatomic, strong) UIImageView *userProfilePhoto;
@end
#import "User.h"
#import "Treehouse.h"
@implementation User
- (instancetype)init
{
self = [super init];
if (self) {
Treehouse *treehouse = [[Treehouse alloc] init];
NSDictionary *friendsDict = treehouse.friends;
_firstName = [friendsDict objectForKey:@"firstName"];
_lastName = [friendsDict objectForKey:@"lastName"];
NSString *profileImageName = [friendsDict objectForKey:@"profilePicture"];
_profileImageName = [UIImage imageNamed:profileImageName];
}
return self;
}
@end
5 Answers
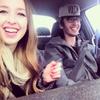
eric hughes
12,345 Points_profileImage = [UIImage imageNamed:_profileImageName];
this is what I wrote for challenge 2
Bradley Maskell
8,858 Points- (instancetype)init
{
self = [super init];
if (self) {
Treehouse *treehouse = [[Treehouse alloc] init];
NSDictionary *friendsDict = treehouse.friends;
_firstName = [friendsDict objectForKey:@"firstName"];
_lastName = [friendsDict objectForKey:@"lastName"];
_profileImageName = [friendsDict objectForKey:@"profilePicture"];
_profileImage = [UIImage imageNamed:_profileImageName];
_userProfilePhoto = [[UIImageView alloc] initWithImage:_profileImage];
//Enter your code below!
}
return self;
}

james white
78,399 PointsThanks eric and Bradley! This was helpful information.
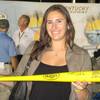
Shannon Siders
17,192 PointsI cant get the first part of the challenge to pass with: _profileImageName = [friendsDict objectForKey:@"profilePicture"];
any suggestions?
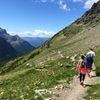
Stephen Wall
Courses Plus Student 27,294 PointsFor task 2 you need to be mindful of the underscores since its within an init method _profileImage = [UIImage imageNamed: _profileImageName];
james white
78,399 Pointsjames white
78,399 PointsHi Timothy!
I assume that this is about the Create UIImageviews challenge: http://teamtreehouse.com/library/build-a-playlist-browser-with-objectivec/managing-playlist-data/creating-uiimageviews
Challenge Task 1 of 3
Let’s assume we have a data model, Treehouse, that contains a property, friends of type NSDictionary which contains information about our friends. We also have a User model that retrieves information from the friends dictionary and creates a User instance. For this challenge, we want to store the User’s profile picture as a property. Let’s start by retrieving the image name as a string from the friends dictionary and storing that in the profileImageName property of the User model. In User.m, use the key profilePicture to get the relevant information.
After downloading the zip files for the course, I combed through them and found file "Playlist.m" seem most relevant (watching the videos, was as usual, not very helpful in figuring out what these challenges want...).
There were two NSDictionary lines in that file and since the question didn't have anything to do with color I focused in on the other one:
NSDictionary *playlistDictionary = library[index];
So looking at the code already given in User.m,
I'm thinking this corresponds roughly (very roughly) to this line:
NSDictionary *friendsDict = treehouse.friends;
..which is basically putting all the treehouse.friends data into friendsDict.
Then there are a couple of lines below that which assign certain keys from within friendsDict :
I'm assuming both these pieces of data are NSString type (even though there isn't specifically a line that says NSString like the line int the zip files Playlist.m):
I'm assuming looking at the above two lines is where you came up with
the two lines you tried to use that didn't pass:
..but I wondered...what if it's as simple as one of the lines that begins with an underscore.
Hmmmm....
So I (after re-reading over the challenge question several hundred times) I came up with this line (after numerous try and error attempts):
_profileImageName = [friendsDict objectForKey:@"profilePicture"];
...which, when added to the user.m file, makes it look like:
..and for some strange reason it passed!
(don't ask me why, though..but hopefully it helps you get further along..)
Challenge 1 of 3 done.
On to the next challenge...
................
Challenge Task 2 of 3
Now that we have the image name as a string, let’s create a UIImage instance using the profileImageName property to retrieve an image. Store this UIImage instance in the profileImage property of the User model. Hint: Use the imageNamed: UIImage class method.
Huh?!
That's where I'm stuck right now...
None of these lines is passing:
Oh, and this thread is the only one under these forum categories:
https://teamtreehouse.com/forum/syllabus:1192
https://teamtreehouse.com/forum/stage:4832
https://teamtreehouse.com/forum/code-challenge:6412