Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial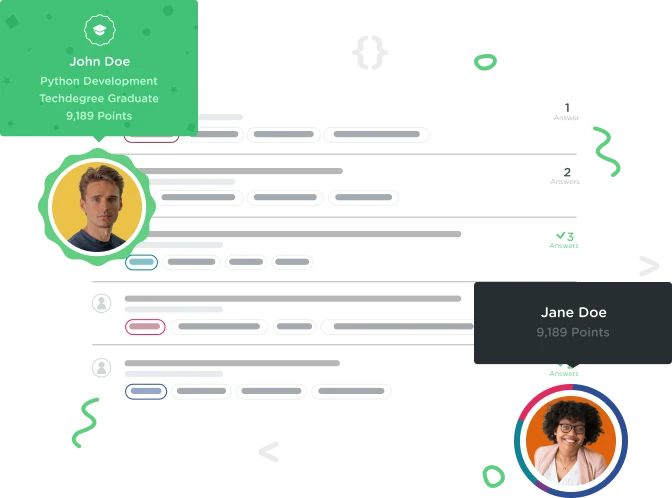

james white
78,399 PointsCreate a property NSArray, called chocolateBox. Use strong and nonatomic modifiers
I guess this course is too new, because I didn't find a lot of forum posts for "Build a Playlist Browser with Objective-C".
I skipped over the first objective (the one involving "Start!"): http://teamtreehouse.com/library/build-a-playlist-browser-with-objectivec/working-with-multiple-view-controllers/uibutton-recap
..because it was too confusing trying to figure out what they wanted,
and now I'm on to the chocolatebox-of-the-GiftBasket challenge.
Link to challenge: http://teamtreehouse.com/library/build-a-playlist-browser-with-objectivec/building-a-music-library-model/creating-a-data-model
The starter/skeleton code they give you (in the file GiftBasket.m) is:
#import "GiftBasket.h"
@implementation GiftBasket
- (instancetype)init
{
self = [super init];
if (self) {
//Add your code below
}
return self;
}
@end
I was thinking the code line that needed to be added would be a literal property:
@property (nonatomic, strong, readonly) NSArray *chocolateBox;
However it is not liking the '@" symbol:
unexpected '@' in program
@property (nonatomic, strong, readonly) NSArray *chocolateBox;
^
1 error generated.
The code is similar to this line:
@property (nonatomic, strong) NSArray *quotes;
..from this forum post:
..so I thought it would work.
Am I just using the wrong code or do I need to add something to the GiftBasket.h file?
Maybe is should be using 'self' in some way?
6 Answers
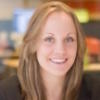
Becky Hirsch
14,069 PointsI was stuck on this one for a while and found the answer in the video: http://teamtreehouse.com/library/build-a-playlist-browser-with-objectivec/building-a-music-library-model/creating-a-music-library
5min and 20sec into the video he talks about using the NSDictionary literal syntax. Doing it this way we don't have to use the dictionaryWithObjects method call. This worked for the first part:
NSDictionary *caramelDelight = @{@"flavor": @"caramel"};
At 6min and 16sec he explains how to store it:
self.chocolateBox = @[caramelDelight];
Hope this helps!

Markus Schober
3,149 PointsThis worked for me:
@property (nonatomic, strong) NSArray *chocolateBox;

james white
78,399 PointsHi Eric, I searched the forum for "setTitle:@"Start!" and came up with this thread (which is probably the one you are talking about):

james white
78,399 PointsThanks Markus for the reply,
but I was being stupid.
The line actually goes into GiftBasket.h not GiftBasket.m
Now I'm on to part 2 of the Challenge:
Challenge Task 2 of 2
Now that we have a chocolate box, let’s add some chocolates. We’re going to represent a single chocolate as an NSDictionary instance. Create an instance of NSDictionary, named caramelDelight and instantiate with the string flavor as a key and the string caramel as its value. Next, store the caramelDelight dictionary in the chocolateBox array.
I think I can do the instantiation, but how to store it in the chocolateBox array I created in the first part of the challenge?

james white
78,399 PointsThanks Rebekah, Actually I did look at the video and he actually used this line of code to define NSDictionary:
NSDictionary *riseandshine = @{@"someKey": @"someValue"};
That line (unfortunately) represents only the generic form of the NSDIctionary call and not anywhere close to what the question was specifically asking for.
Note: I did also play around with dictionaryWithObjects (I was desperate), but nothing using that method call would pass for this challenge.
While I spent 3 days trying to figure this one out I had a chance to research the other UIButton recap challenge (in the "Working With Multiple View Controllers") section of the build a playlist browser with objective-c course): https://teamtreehouse.com/library/build-a-playlist-browser-with-objectivec/working-with-multiple-view-controllers/uibutton-recap
Challenge Task 1 of 2
Let’s assume we have an IBOutlet of type UIButton, named timerButton, as a property in our view controller header file. In the implementation file, set the title of the button to “Start!” for the state configuration UIControlStateNormal. Hint: Check out the UIButton class reference in the documentation.
Here's the code I finally came up with for the viewController.m file:
#import "ViewController.h"
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
UIColor *redColor = [UIColor redColor];
// Enter your code below!
[_timerButton setTitle:@"Start!" forState:UIControlStateNormal];
[_timerButton setTintColor:[UIColor redColor]];
}
@end
Note 1 : these extra added lines of code also pass the second part of the challenge:
Challenge Task 2 of 2
Before we finish let’s also programmatically change the tint color of our button to something more appealing. I’ve set up a UIColor object for you to use and like last time, I want you to use the documentation or the Internet to figure out which method to use. Assign the redColor UIColor object to the tint property of the timerButton.
Note 2: The videos that immediately proceeded these objectives were of no help at all.
Here's some links there were helpful:
This link was only so-so helpful (but should have been given in the question instead of the vague "Hint: Check out the UIButton class reference in the documentation.":
...............
What really helped me was these two StackOverFlow threads/posts...
This one:
http://stackoverflow.com/questions/9975842/button-changes-title-every-time-pressed
..had these two helpful lines of code:
@property (nonatomic, retain) UIButton *poga1;
[poga1 setTitle:@"My Title" forState:UIControlStateNormal];
..but it still wasn't passing until I found this: http://stackoverflow.com/questions/20024141/uibutton-enable-doesnt-work
which had these two helpful lines of code:
[_startButton setTitle:@"Fetching Location" forState:UIControlStateDisabled];
@property (weak, nonatomic) IBOutlet UIButton *startButton;
So the first StackOverFlow link told me how to use
//code line fragment only
setTitle:@"
..with:
//code fragment line only
forState:UIControlStateNormal
The second StackOverFlow link clued me into using the underscore:
[_timerButton setTitle:@"Start!" forState:UIControlStateNormal];
..instead of:
//wouldn't pass
[timerButton setTitle:@"Start!" forState:UIControlStateNormal];
Anyway I got one finally challenge left, the infamous 3 objective, "Create UIImageViews": http://teamtreehouse.com/library/build-a-playlist-browser-with-objectivec/managing-playlist-data/creating-uiimageviews
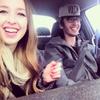
eric hughes
12,345 Pointsthanks alot for these answers, I posted an answer for the challenge http://teamtreehouse.com/library/build-a-playlist-browser-with-objectivec/working-with-multiple-view-controllers/uibutton-recap
its somewhere in the forums ,
Happy coding everyone!