Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial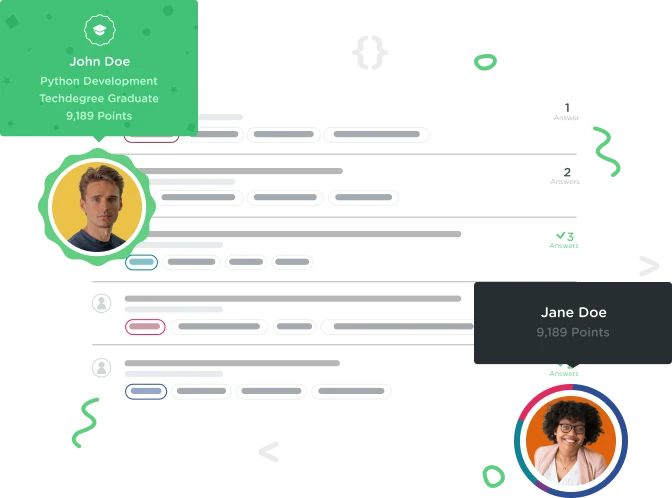

Eric Dotson
1,933 PointsCrystal Ball code errors please help
package com.example.ed;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v7.app.ActionBarActivity;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends ActionBarActivity {
/* (non-Javadoc)
* @see android.support.v7.app.ActionBarActivity#onCreate(android.os.Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Declare our view variables and assign them the Views from the layout file
final TextView answerLabel = (TextView) findViewById(R.id.TextView1);
final Button getAnswerButton = (Button) findViewById(R.id.button1);
View.OnClickListener onClickListener = new View.OnClickListener() {
@Override
public void onClick(View v) {
//The button was clicked, so update the answer label with an answer
String answer = "Yes";
answerLabel.setText(answer);
}
};
getAnswerButton.setOnClickListener(onClickListener);
if (savedInstanceState == null) {Object R =
getSupportFragmentManager().beginTransaction()
.add(R.Id.container, new PlaceholderFragment())
.commit();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
/**
* A placeholder fragment containing a simple view.
*/
public static class PlaceholderFragment extends Fragment {
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_main, container, false);
return rootView;
}
}
}
4 Answers

Ernest Grzybowski
Treehouse Project ReviewerI think you are confused because the default project set up for Android now includes "fragments". This was added very very recently and changes the way your project is set up compared to what the videos are showing. Ben Jakuben knows about this and is working on updating the videos, but for the meantime he included some hints in the teachers notes.
Check the teachers notes here: http://teamtreehouse.com/library/build-a-simple-android-app/getting-started-with-android/android-setup-and-the-crystal-ball-project-2
or
just use this link to help you walk through the differences of creating a project: http://treehouse-code-samples.s3.amazonaws.com/Android/android_bundle_diffs.html

Ben Jakuben
Treehouse TeacherHey all, the updated videos are live. Check out the Initial Layout video for step-by-step instructions on how to get rid of the Fragment.
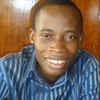
Victor Okech
1,352 PointsI am also having the same problem as Eric Doston and I am guessing that the best way to overcome this will be by downloading and installing the Android Development Bundles provided here. That way we will have a more or less similar environment setup and the changes that have been incorporated to Android lately will pose no problem. Let me try that out and see what happens.
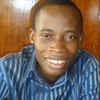
Victor Okech
1,352 PointsWell, I have looked at my code and the reason why I was having a problem is that the new android project setup with support for Android API 7 (Eclair) has the Fragment Layout (fragment_main.xml) and the normal layout (activity_main.xml) but it tends to open the MainActivity.java class and the fragment_main.xml layout. So be sure to open your activity_main.xml file, since it is the once referenced by the class MainActivity.java. Newbies will just assume and use whatever the project setup wizard avails and that is where the problems begin.

Ernest Grzybowski
Treehouse Project ReviewerYou should use this tutorial for now: http://treehouse-code-samples.s3.amazonaws.com/Android/android_bundle_diffs.html
I'm not sure why Google is pushing their templates to use fragments, but it's the route that they are taking now. I think this is too confusing for new users, but that's out of my control.
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherThanks Ernest! I'm hoping we can publish the video fixes today. fingers crossed