Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial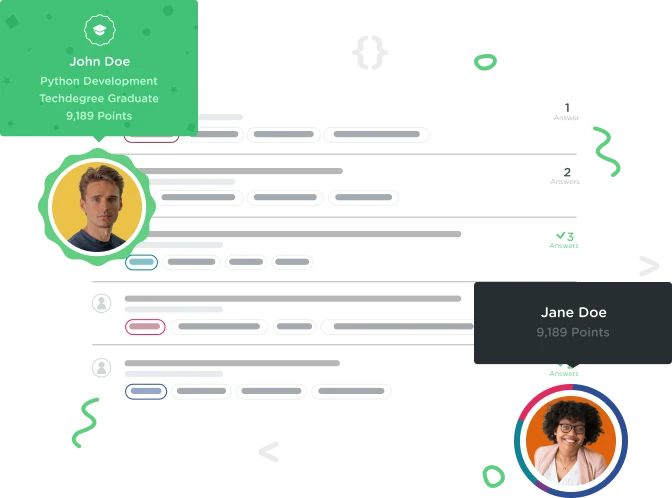

Burak Hurgezen
654 PointsDevise (updated) with Ruby 2.1.1 and Rails 4.0.3
I am using Ruby 2.1.1 and Rails 4.0.3 with the latest version of Devise. I do not have attr_accessible section in user.rb folder. This causes some troubles in the following steps. If I manually write up it into the user.rb file. I get an error once i start p my server and try out devise. The error says attr_accessible was moved in to a gem. How do I change/add values to attr_accessible?
3 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsWell, you can either add gem 'protected_attributes'
to your Gemfile and then run bundle
. After that, you can proceed the same way as it's done in the video, or if you look up the documentation for Devise (look for Strong Parameters) you can do something like this:
before_filter :configure_permitted_parameters, if: :devise_controller?
protected
def configure_permitted_parameters
devise_parameter_sanitizer.for(:sign_up) { |u| u.permit(:username, :email, :first_name, :last_name, :profile_name, :password, :password_confirm) }
end
This would go into your application controller.

Dino Paškvan
Courses Plus Student 44,108 PointsTo be honest, I've never found a comprehensive Ruby on Rails tutorial that wasn't outdated at least a little bit in some way. Rails moves fast and for most tutorials, the best thing you can do is lock your Ruby/Rails version with RVM to the one used in the tutorial.
However, in the case of this tutorial, it's not really that bad. I've managed to go through the whole thing using the latest version of Rails. To be honest, the biggest difference was Bootstrap (now at version 3.1.1, as opposed to 2.something at the time of the tutorial), and that's just cosmetics. Bootstrap is well documented so this is hardly an issue.
I found that the effort involved in fixing the differences just made me learn more about Rails and how the whole framework works.
For instance, later on, when you're writing tests for the statuses, you'll deal with params in a different way, as you don't access the params directly, but rather through a method status params
so you do something like this to remove the user_id
key:
sp = status_params
sp.delete(:user_id) if sp && sp.has_key?(:user_id)
Basically you're assigning the return value of a method to a variable which allows you to remove the offending key from it. And then throughout the update
action you use sp
instead of status_params
. And you can modify the method as well, to get rid of another error during testing:
def status_params
params.require(:status).permit(:user_id, :content) if params[:status]
end
You'll also get some deprecation warnings (when using @user.statuses.all
) but you can ignore those more or less. The tests are now located in different directories, but the file names are the same so you can still keep up with that.
The point is, it's not that bad and none of these differences are deal breakers. I honestly believe it's worth the effort to find the solutions for these difficulties yourself.

Blayne Holland
19,321 PointsI agree, trying to figure out why things weren't working really helped me to become more familiar with RoR. So in a way, I'm glad I ran into problems.

Burak Hurgezen
654 PointsThanks man, I really appreciate your help. Being new to all this and not having it work over and over again is pretty frustrating but I, sure, will be trying this tutorial again with your input. Also as Blayne said it is rather helpful to run into problems. Thanks!
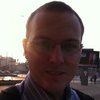
Todd Nestor
10,689 PointsDino Paškvan I'm at that stage where I need to be using the status_params since I am using rails 4, what file is that in?
I've moved on from that part for now and am in the middle of building the profile page now, but it bugs me that my status tests don't work. All the other tests work, just not the status tests where I need to do the sign_on and all that.

Dino Paškvan
Courses Plus Student 44,108 PointsThat's in the statuses_controller.rb
file.
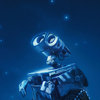
Daniel Cardenas
Courses Plus Student 9,236 PointsI agree with you Dino. Working through problems in Ruby on Rails has definitely helped me understand this program better.
Blayne Holland
19,321 PointsBlayne Holland
19,321 Pointsawesome. thanks!
Burak Hurgezen
654 PointsBurak Hurgezen
654 PointsThank you so much. Even though I managed to get through that step, the version differences of all the software between the time all the videos were created and now causes lots of trouble. Would you happen to know a more up-to-date tutorial on creating a website using Ruby on Rails?