Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial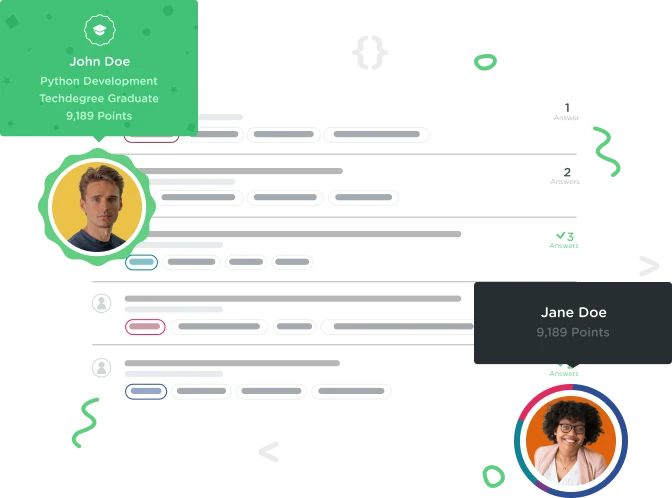
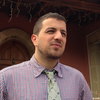
Alex Tasioulis
4,950 Pointsextra credit fizzbuzz
'''var number = 100;
while (number) {
if (number%3) { console.log("fizz"); } else if (number%5) { console.log("buzz"); } else if (number%5 + number%3) { console.log("fizzbuzz"); } else { console.log(number) } number = number-1; }'''
Here's what I got for the extra credit.. but it's not working. I can't think clearly I don't think. Any suggestions on how to fix this?
11 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsA single =
assigns values. When you're comparing, it should be a ==
or ===
. The second one is more recommended.
You could write a for loop:
for(var i = 1; i < 101; i++) {
// check the value of i here and console.log the stuff you need
// you don't need to increase the value of i, the for loop does it for you
}

Jason Anello
Courses Plus Student 94,610 PointsYou want to compare against 0. Like this if (i%15 == 0) {
The idea is that you're checking if the remainder is equal to 0. Meaning, it was evenly divisible.
The rest looks good to me except that they want "fizz" displayed for multiples of 3. You have fizz and buzz reversed.

Dino Paškvan
Courses Plus Student 44,108 PointsFizzBuzz should be displayed when a number is divisible both by 3 and 5 (meaning it should be divisible by 15). That should be your first check, otherwise you'll end up displaying fizz
for numbers that should say fizzbuzz
.
When you're using the modulo (%
) operator, you should compare the result of the operation to 0 not just if (number%3)
as you're doing. A number is divisible by another number if the result of the modulo operation is equal to 0. In fact, your comparison will have just the opposite effect because when the result of the modulo operation is 0
, JavaScript will treat that 0
as a falsy value, while any other number is considered to be a truthy value and as such satisfies the if
.
Also, FizzBuzz typically goes from 1 to 100, not backwards as your loop would execute it.

Jason Anello
Courses Plus Student 94,610 PointsAs Dino mentioned, you need to do a comparison. A single = is an assignment. You need ==
or ===
to do a comparison.
If the videos haven't covered for loops yet then I would wait till you get to that. Since you know how many times you're looping. a for loop is a good choice for this. You can easily set it to loop 100 times. This way you don't have to manually control how many times your while loop runs..

Jason Anello
Courses Plus Student 94,610 PointsDino Paškvan, I would appreciate your thoughts on this.
I've never coded this problem or did any tests on it but I've wondered if putting the modulo 3 check before the modulo 5 check would make the code run faster. I think either one could come before the other correct?
My theory is that since there are more multiples of 3 than 5 there would be less conditional checks by putting the mod 3 check before mod 5.
Any thoughts on that?

Jason Anello
Courses Plus Student 94,610 PointsI realize it's probably negligible for 100 numbers but I'm talking about for a large number.
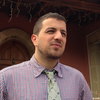
Alex Tasioulis
4,950 PointsThank you so much for the reply. That's very helpful. I still need some help, though.
Questions:
If I start with 1 and go up, how will I end the loop? I would imagine something like if (number >=100) { number = 0; console.log("loop to 100 is done")}
Comparing the result to 0, that's what I wanted to do: something like if (number%3=0) { console.log("fizz") }
But that gives me an error! (Uncaught ReferenceError: Invalid left-hand side in assignment). Any thoughts? Thanks!
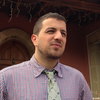
Alex Tasioulis
4,950 PointsThank you both so much. I think I got it:
for(var i = 1; i < 101; i++) {
if(i%15==true) {
console.log("fizzbuzz");
}
else if (i%5==true) {
console.log("fizz");
}
else if (i%3==true) {
console.log("buzz")
}
else {
console.log(i);
}
}
I'm pretty sure it's working, but is this the correct way to do it?
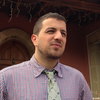
Alex Tasioulis
4,950 PointsOhhhhhhhhhhhhh OK now I get it. It's defo working properly this time, too. Thank you!

Dino Paškvan
Courses Plus Student 44,108 PointsYou know, that's an interesting question. I haven't really coded this problem either, but I've seen people optimising it in various ways, but this was never really mentioned. Usually the optimisation includes omitting the newline after the output, so that you don't have to check explicitly for divisibility by 15. Instead you just check for 3 and 5 and if it passes both of them the words will be printed out one after another, outputting fizzbuzz
. Instead of omitting new lines, you could concatenate the words/numbers and output one long string after the loop has finished.
Anyway, back to the question...
Most implementations do check for 3 before 5, as it's somewhat logical to do so, but I wonder how many of those do it because that would be faster.
I think the idea has merit but even on large numbers the difference would probably be negligible. In fact, I went to test, using node.js. After all, this is a JavaScript discussion. :)
After numerous tests to yield average values on 1 000 000 000 iterations, the version you predict to be faster really is faster by 3%. The difference in average execution times is 0.536
seconds.

Jason Anello
Courses Plus Student 94,610 PointsThanks for doing those tests. So not really much of an improvement.
The optimization you mentioned is something I never would have thought of. Seems like the output would be messy though. I guess you're forced to do the mod 3 first with that method.

alkin kasap
4,765 Pointsinstead of
if(i%5==true)```
you should use
if(i%5==0)```
because first code will check if output is 1 not 0. by using first code you'll get buzz in numbers which are 5x + 1 not 5x. so dont use "true" but use "0".
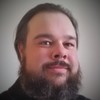
Noah Savage
1,512 PointsThis is my solution. It is a bit longer, but I wanted to adhere to most of the conventions shown in the videos. I'm very new to programming, so I like to use descriptive variables (as in "counter" below) rather than a letter. I also find it easier (for me) to follow code if I modify a variable in terms of itself (e.g. counter = counter + 1) instead of counter++.
Writing code like this is simply easier for me to process visually (for now lol).
Thank you, everyone, for your posts, I was lost in endless loop-land until I saw other examples.
console.log("Before");
for (var counter = 1; counter <= 100; counter = counter + 1) {
if (counter % 3 != 0 && counter % 5 != 0) {
console.log(counter);
} else if (counter % 3 == 0 && counter % 5 == 0) {
console.log("fizzbuzz");
} else if (counter % 3 == 0) {
console.log("fizz");
} else if (counter % 5 == 0) {
console.log("buzz");
} else {
console.log(counter)
}
}
console.log("After");
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsBeat me to it. And a better answer. :)