Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial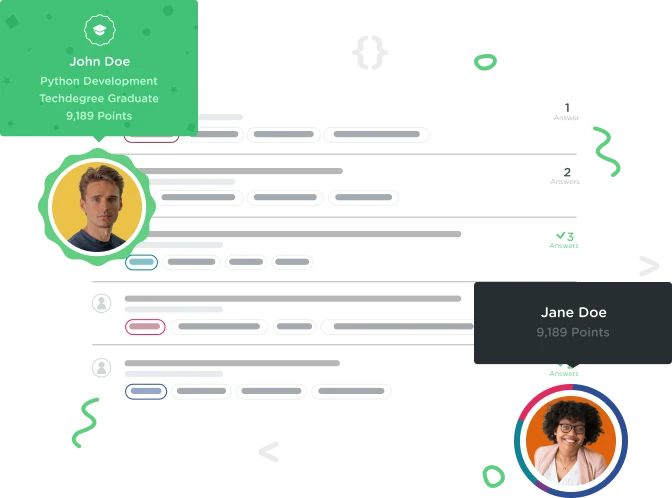
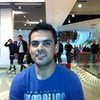
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsFlask and Jquery Ajax
I want to populate a html table using jquery ajax. The user enters a job number and on focusout a ajax is sent to my flask route but not sure how i send the response back and populate the table.
my html form looks like this
<form action="/findjob" method="POST" role="form">
<div class="form-group search-margin">
<input class="form-control" type="text" name="jobnumber" id="jobnumber" placeholder="search by job number">
</div>
</form>
my flask route looks like this
@app.route('/findjob',methods=['GET','POST'])
def findjob():
try:
jobnumber=str(request.form["jobnumber"])
jobnumber=jobnumber.upper()
jobs=models.Job.select().where(models.Job.jobnumber**jobnumber)
if jobs.count()==0:
abort(404)
else:
return json.dumps({'status':'OK','jobs':jobs,'jobs_count':jobs.count()})
except:
abort(404)
and my jquery function looks like this
$('#jobnumber').focusout(function(){
var jobnumber=$('#jobnumber').val()
$.ajax({
url: '/findjob',
data: $('form').serialize(),
type: 'POST',
success: function(response) {
//I need to some how be able to populate my table with the returned list called jobs;
},
error: function(error) {
alert(error);
}
});
});
table i need to populate looks like this
<table class="table table-striped table-margin">
<tr>
<th>Job number</th>
<th>Job title</th>
<th>Week</th>
<th>Day</th>
<th>Supplier</th>
<th>Created By</th>
<th>View Transfer</th>
{% if current_user.admin %}
<th>Record Transfer</th>
{% endif %}
<th>Edit Job</th>
<th>Delete Job</th>
</tr>
{% for job in jobs %}
<tr>
<td class="hidden">{{job.job_id}}</td>
<td>{{job.jobnumber}}</td>
<td>{{job.jobname}}</td>
<td>{{job.week}}</td>
<td>{{job.day}}</td>
<td>{{job.supplier_name}}</td>
<td>{{job.created_by}}</td>
<td><a href="/filestransfered/{{job.job_id}}" class="glyphicon glyphicon-eye-open"></a></td>
{% if current_user.admin %}
<td><a href="/transferfiles/{{job.job_id}}" class="glyphicon glyphicon-record"></a></td>
<td><a href="/editjob/{{job.job_id}}" class="glyphicon glyphicon-edit"></a></td>
<td><a href="/deletejob/{{job.job_id}}" class="glyphicon glyphicon-trash"></a></td>
{% endif %}
</tr>
{% endfor %}
</table>
2 Answers

Iain Simmons
Treehouse Moderator 32,305 PointsYou would have to use jQuery to populate the table again with the new values. Remove all the td
and tr
elements (except the first tr
of course) and recreate them by using loops and concatenation on the JSON values and then appending to the table
.
Flask isn't a front-end framework, so it won't dynamically modify the DOM/HTML elements like frameworks such as Angular, Ember, Backbone, etc.
You can of course use Flask alongside a JavaScript-based front-end framework. Here's a 3-part article about creating a blog using Flask and Angular:
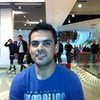
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsI was thinking of building the th and td in the flask view itself and then passing that as a response to the ajax request and populating the table that way for now I have just used normally python. But thanks anyway very helpful links.

Iain Simmons
Treehouse Moderator 32,305 PointsYeah you could build a Flask view/template that just contains the table HTML and then instead of returning the JSON to the Ajax callback, you would return the HTML content preformatted by Flask, and insert it into a wrapping div
.
That would also allow you to make a format for an empty result: instead of just having the table headers, you could return a more helpful 'no results' message.