Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial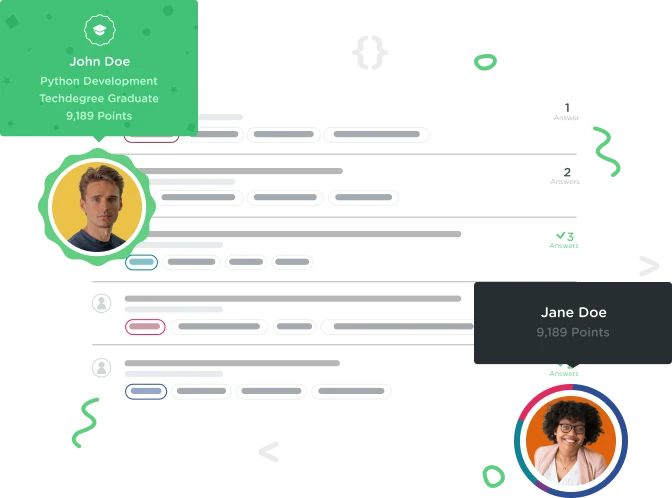
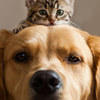
Devin Scheu
66,191 PointsHelp With Java
Question: So at your new Java job, you've written a brand new Shopping Cart system for the website. After some developers have used the objects you created for a while, they ask you to make it easier to add things to the cart.
Check out more instructions in Example.java. Once you implement their request, check your work, and you will pass the challenge.
Code:
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
// Uncomment this line after using method signatures to solve their request in ShoppingCart.java
// cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
quantity = 1;
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
4 Answers
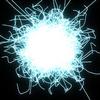
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHello, what you are being asked to do is create a method that adds only one item. Leave the initial method as is, so it should look something like
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
public void addItem(Product addedItem) {
addItem(addedItem, 1);
}
Also, be unsure to uncomment the cart.addItem(dispenser); from the origional code.

Jess Sanders
12,086 PointsExample.java has a line of code that is commented out
cart.addItem(dispenser);
If all you do is uncomment this line and check your work, you get a compiler error. We need to write a second addItem method that only takes one argument, rather than two.
So, in ShoppingCart.java, we can copy and paste the existing addItem method, but remove the second argument. We also need to change what is printed, because we know that we are Adding 1 of the item to the cart.
The output of your new addItem method must read
"Adding 1 of %s to the cart.\n"

daviddavis3
4,904 PointsI passed with the solution as done above, with the addItem method with two parameters. But I also tried creating a new one parameter addItem method in the ShoppingCart class. I wonder why not use this?
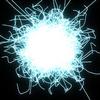
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsThe main reason that you would want to keep this the same as above is because in Object Oriented programming which is what Java is you want different objects or classes to do specific jobs. This leads to hunting down bugs easier and updating your code. Mostly it's just to make your job easier down the road when each class has specific duty and jobs.

daviddavis3
4,904 PointsI did this challenge a second time. First I tried just to just uncomment the "cart.addItem(dispenser);" in the Example class. But that had an error. So, I created another method signature for addItem,, that only had one parameter, in the ShoppingCart class. The "1" is a literal, so it doesn't count as a parameter. When I did that, it passed.
So, when I did the challenge the first time with "cart.addItem(dispenser);" in Example class, but it erred. When I had "cart.addItem(dispenser, 1);", it passed. I wonder why "cart.addItem(dispenser);" can't work, since it more closely matches the method signature?
Devin Scheu
66,191 PointsDevin Scheu
66,191 PointsThank You Rob Bridges for clearing this up for me.