Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial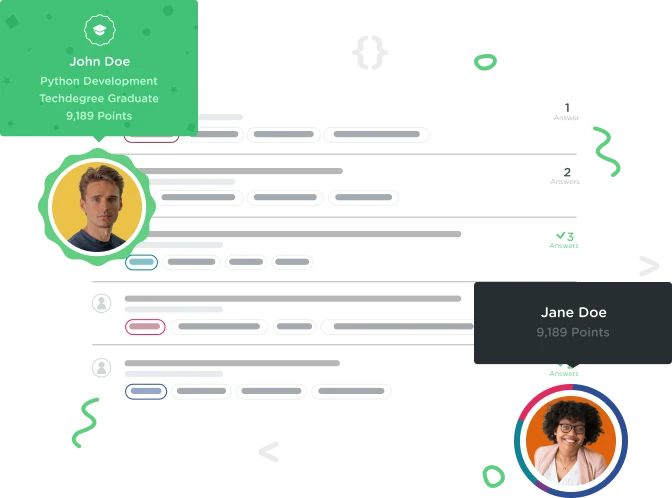

bauke plugge
291 Pointshi my edit friendspage is taking the full page of the phone. did I forget something?
and my progressbar is also not loading. the is nothing also when I leave the false part away.
8 Answers
Henrik Hansen
23,176 PointsIf the problem is that you dont see the Action bar, then I think you must change the class extension to ActionBarActivity.
Make sure you use the correct inputs.
The cons of this is you will have to change some code of the class. You might need to set up your layout with a ListView, then get the id of that ListView and use the ListViewAdapter with that one.
I think the reason for the "Action Bar Issues" is because a lot of stuff in the API has changed with Lollipop and material design, and Android Studio is mainly targeted for the newer API. I think it is possible to change the "Target SDK settings" in some places and that might be a fix. I didnt look into that thou.

Robert Proulx
6,538 PointsOkay, struggled with the same thing at this same spot, and couldn't get the "up" navigation because the action bar won't work in when using "extends ListActivity". 2 Step Fix:
1) Change the activity back to "extends ActionBarActivity." 2) Build the core code for this section in the onResume of a ListFragment that gets called from the ActionBarActivity.
Here is the finished code for the EditFriendsActivity tab of this section using an ActionBar/ListFragment solution. The ListFragment section follows right along with Ben with a few minor tweaks, so you should be able to absorb the lesson pretty easily. This code IS working for me now.
public class EditFriendsActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
}
@Override
protected void onResume() {
super.onResume();
FragmentManager fm = getSupportFragmentManager();
EditFriendsFragment list = new EditFriendsFragment();
fm.beginTransaction().add(android.R.id.content, list).commit();
}
public static class EditFriendsFragment extends ListFragment {
public static final String TAG = EditFriendsFragment.class.getSimpleName();
protected List<ParseUser> mUsers;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
return super.onCreateView(inflater, container, savedInstanceState);
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.orderByAscending(ParseConstants.KEY_USERNAME);
query.setLimit(1000);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
if (e == null) {
//Success
mUsers = users;
String[] usernames = new String[mUsers.size()];
int i = 0;
for (ParseUser user : mUsers) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
getListView().getContext(), android.R.layout.simple_list_item_checked,
usernames);
setListAdapter(adapter);
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
addFriendCheckmarks();
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getListView().getContext());
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
@Override
public void onListItemClick (ListView l, View v, int position, long id){
super.onListItemClick(l, v, position, id);
if (getListView().isItemChecked(position)) {
// add the friend
mFriendsRelation.add(mUsers.get(position));
} else {
// remove the friend
mFriendsRelation.remove(mUsers.get(position));
}
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null) {
Log.e(TAG, e.getMessage());
}
}
});
}
private void addFriendCheckmarks() {
mFriendsRelation.getQuery().findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
if (e == null) {
//list returned - look for a match
for (int i = 0; i < mUsers.size(); i++) {
ParseUser user = mUsers.get(i);
for (ParseUser friend : friends) {
if (friend.getObjectId().equals(user.getObjectId())) {
getListView().setItemChecked(i, true);
}
}
}
} else {
Log.e(TAG, e.getMessage());
}
}
});
}
}
}

Vipul Garg
354 PointsThis code is working perfectly except it prints empty list message every time even with the filled list.

Robert Proulx
6,538 PointsThis is because the empty view in the layout is not actually part of the list fragment being made, so you'll have to set the empty text in the actual code. In the next course after this, "Implementing Designs for Android", they give Ribbit a more polished look and this includes updating all the "List Views" to "Grid Views", and they use the code below to put a TextView into the empty GridView setting. You should have a similar option you could adapt to work in the ListFragment.
TextView emptyTextView = (TextView) rootView.findViewById(android.R.id.empty);
mGridView.setEmptyView(emptyTextView);

bauke plugge
291 Pointswhat part will you need ?
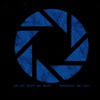
James N
17,864 Pointsfirst let's make sure that the action bar isn't hidden. can I see your styles.xml ? it should be under values In the res folder.

bauke plugge
291 Points<resources> <style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> </style> </resources>

bauke plugge
291 Points<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<!-- Customize your theme here. -->
</style>
</resources>
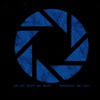
James N
17,864 Pointsok... so the theme is fine. what do you mean by "the editfriends page takes up the full page of the phone"?? does "page" mean "screen"?

bauke plugge
291 Pointssorry yes, i meant the full screen of the phone and not like the other fragments leave the top bar as is. and display it under it
bauke
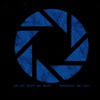
James N
17,864 Pointshmm... I don't 100% know what to do here... but there is someone that has commented on my most recent forum post and helped me out. ill send him a link to this chat and see if he can help.
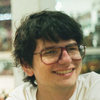
Marcos Philipson
7,725 PointsRobert Proulx solution wasn't working for me until I changed:
//import android.support.v4.app.FragmentManager;
//to
import android.app.FragmentManager;
and also:
//FragmentManager fm = getSupportFragmentManager();
//to
FragmentManager fm = getFragmentManager();
Don't know why but maybe this helps someone
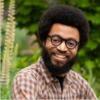
Nicolas Hampton
44,638 PointsComplete working code to start the class with:
package com.staggarlee.ribbit;
import android.app.AlertDialog;
import android.app.ListFragment;
import android.app.FragmentManager;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseQuery;
import com.parse.ParseUser;
import java.util.List;
public class EditFriendsActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
}
@Override
protected void onResume() {
super.onResume();
FragmentManager fm = getFragmentManager();
EditFriendsFragment list = new EditFriendsFragment();
fm.beginTransaction().add(android.R.id.content, list).commit();
}
public static class EditFriendsFragment extends ListFragment {
public static final String TAG = EditFriendsFragment.class.getSimpleName();
protected List<ParseUser> mUsers;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
return super.onCreateView(inflater, container, savedInstanceState);
}
@Override
public void onResume() {
super.onResume();
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.orderByAscending(ParseConstants.KEY_USERNAME);
query.setLimit(1000);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
if (e == null) {
//Success
mUsers = users;
String[] usernames = new String[mUsers.size()];
int i = 0;
for (ParseUser user : mUsers) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
getListView().getContext(), android.R.layout.simple_list_item_checked,
usernames);
setListAdapter(adapter);
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getListView().getContext());
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
}

Alex Hexan
Courses Plus Student 769 PointsThank you so much! I've been having this error for a while and your code fixed it. Amazing. Thanks again.
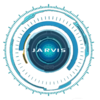
jenyufu
3,311 Pointsis this code before the lesson or after the lesson. Because I haven't started this video (Search Friends From a List) yet and I don't know if I should use your code and replace mine or wait until after the video to replace it with yours?
James N
17,864 PointsJames N
17,864 PointsI don't know... it seems lots of people are getting this error.
can you show me your code?? ill do my best to help you.