Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial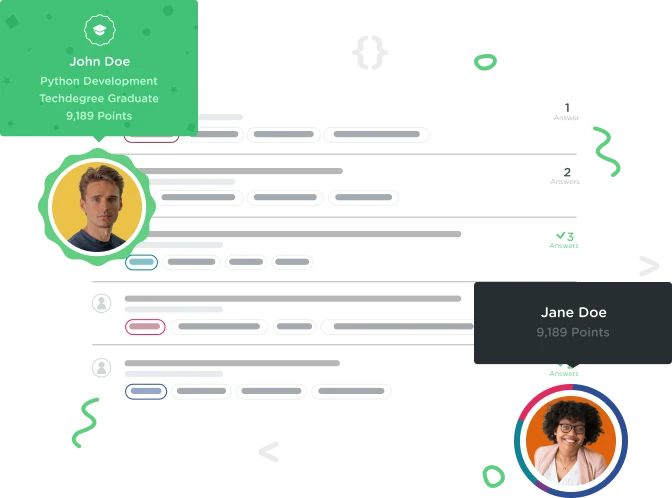

ch11
3,901 PointsHow can I modify code to ensure that randomColor and randomFact never return a number that is the same as the previous?
Referring to the "Build a simple iPhone App" Random Fact example: Sometimes the random fact or color that is generated after pushing the button ends up being the same as the preceding fact or color. I attempted to insert a do while function, as seen below, to compare the new random number to the previous and continue to generate random numbers as long as they match...or until they are different.
However, when I try to set currentNumber equal to the new random number, in order to compare on the next button push, I get the error...Cannot assign to 'currentNumber' in 'self'
struct FactBook {
let factsArray = [
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built."
]
var currentNumber: UInt32 = 0
func randomFact() -> String {
var unsignedRandomNumber: UInt32
var unsignedArrayCount = UInt32(factsArray.count)
do {
unsignedRandomNumber = arc4random_uniform(unsignedArrayCount)
} while currentNumber == unsignedRandomNumber
currentNumber = unsignedRandomNumber
var randomNumber = Int(unsignedRandomNumber)
return factsArray[randomNumber]
}
}

ch11
3,901 Pointsyeah, I think the problem is around the currentNumber. I need to initialize it in the beginning without giving it a value so when the function is called again, it retains the value given when it is set equal to the previous 'unsignedRandomNumber.
Maybe initializing it as an optional within the function?
Anthony Munoz
1,935 PointsAnthony Munoz
1,935 PointsHave you tried moving var currentNumber: UInt32 = 0 into function ?