Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial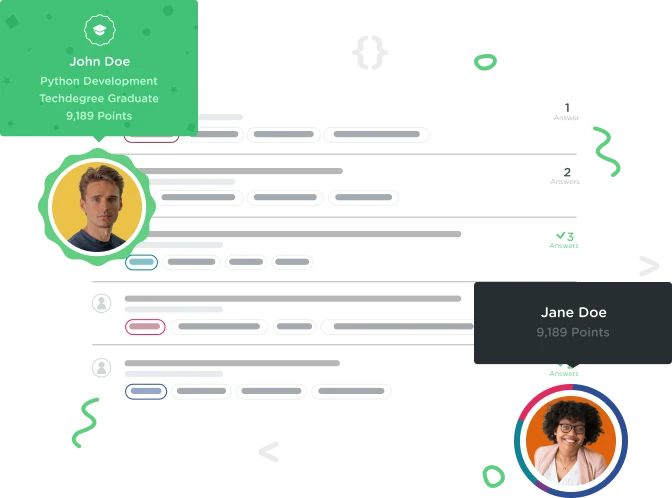

ISAIAH S
1,409 PointsHow do I do this?
Challenge Task 3 of 3
Alright, now let's order the blog post's creation date chronologically, oldest first.
NOTE: The app where this class is being used ensures that no two blog posts are posted at the same time, so you do not need to worry about any edge cases.
Thanks!!
package com.example;
import java.util.Date;
public class BlogPost implements Comparable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
public int compareTo(Object obj) {
BlogPost other = (BlogPost) obj;
if (equals(other)) {
return 0;
}
return 1;
}
}
6 Answers

Craig Dennis
Treehouse TeacherHmm... I'm assuming you left the return 1;
in there right before this. Can you include all your code if that doesn't help?

Andre Colares
5,437 Points public int compareTo(Object obj){
BlogPost other = (BlogPost) obj;
if (this.equals(other)){
obj = (BlogPost)obj;
return 0;
}
int compareDate = mCreationDate.compareTo(other.mCreationDate);
return compareDate;
}
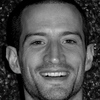
Joe Goodall
3,483 PointsThis worked for me ;-)

jinhwa yoo
10,042 Pointshey can you explain why you write
BlogPost other = (BlogPost) obj; if (this.equals(other)){ obj = (BlogPost)obj; // how did you make it????
int compareDate = mCreationDate.compareTo(other.mCreationDate); return compareDate; // can you explain be more specific????

Craig Dennis
Treehouse TeacherRemember that java.util.Date
instances have a compareTo
method as well. Oh hey, by default that method sorts chronologically, that's handy!
That help?

ISAIAH S
1,409 PointsI wrote:
int compareDate = mCreationDate.compareTo(other.mCreationDate);
return compareDate;

Arthur Podkowiak
3,633 PointsCraig, I'm having a lot of trouble understanding this stuff as well. Even though we write a method that compares the objects, how do we know it will compare by date? Why do we even say if(equals(other))
? Do we just write that to see if the (for example from the Treets program) Treets literally are completely equal? As in they say the same thing in the Treet? Or is it to make sure that you can use the type cast correctly (which I also have a lot of trouble understanding such as when to use it and why you need to use it such as in this case)? I see how we compare the dates by using mDescription.compareTo(other.mDescription)
but how does this actually allow it to sort? (Again in the Treet program, we don't have the Example class implementing comparables, how do we know that Array.sort will automatically sort by the date when it does not call the compare method at all?)

Craig Dennis
Treehouse TeacherHi Arthur Podkowiak !
So it will help if you picture sorting an array by moving through each element, comparing one to the next. So let's imagine we have the first two elements of the array, arr[0]
and arr[1]
. When comparing ordering two objects you need to decide which one comes first. The way you do that is pretty contextual to the objects you are dealing with. If they were String
s, you'd probably assume alphabetical, but if they are objects how would you know how to compare them?
The way you define how to sort objects is by defining that compareTo
method that is required by the Comparable
interface. So in the current example, we'd take the first object, arr[0]
and compare it to arr[1]
. If you picture the Arrays.sort
method doing something like this arr[0].compareTo(arr[1])
. The method returns a -1 if the passed in object (arr[1]
) should come before the current object arr[0]
. If it should come after it returns a 1. After it figures out which comes first, it sets their order correctly and moves to the next one, and does a sort arr[1].compareTo(arr[2])
(The algorithm is a bit more complex, but that is the general concept, it uses compareTo
to sort).
The equality bit is important because it's part of the agreement of using the Comparable
interface. It requests that if objects are equal that we return 0. All Objects have an equals
method, and typically you override this to define what you mean by equality. As you asked, the Treet class should probably have an overridden method called equals that checks to see if the author, description and creation date are the same, and if they are, then return true.
Hope that helped to clear things up a bit!

Arthur Podkowiak
3,633 PointsCraig, that does help a lot, and thank you for the quick response! So when using the sort
method it needs to find a way to sort objects and that is by using the Comparables
interface. Would you be able to tell me why we need to type cast in this situation? I've researched it more and I think I understand it a little better now. Our Treet class has certain fields and methods in it that it uses, but its parent class is Object (this is the super class of every object, correct?) and this has its own fields and methods, so typecasting kind of allows you to make one or the other maybe not have the same fields, but be able to use methods within the classes?
Someone explained to me this is like have a parent class "bicycle" and then having "mountain bikes" and "motorcycles" being subclasses. They have very similar fields, but are used for different applications. But we could type cast a motorcycle into a bike when riding down a normal street because they both can be used for this application.
Why do we need to make an object in the Object class using (Object obj)
, why can't we just make another object in the Treet class if we are comparing two treets anyways? Since we implement the Comparables interface shouldn't all treets be able to use this anyways, so what's the point of making an object in the Object class and then type casting it right back to the Treet class?
I also wanted to tell you that this is the best intro programming course I've taken, you guys do a great job and you're a fantastic teacher!

Craig Dennis
Treehouse TeacherAww shucks, thanks Arthur!
Good news is you are just a few steps ahead of yourself. Move into the next couple of Videos, and I'll clean that up with Generics, we just haven't talked about them yet in this part of the course, I'm headed straight there. ;)
Let me know if that doesn't clear up your confusion. More than happy to explore it deeper.

Arthur Podkowiak
3,633 PointsCraig, so I just finished up a lot of the 3rd course in Java Data Structures. I learned about generics and see how they can take out type casting, because essentially you are kind of type casting at the beginning instead of after already creating the object. Now, I'm wondering with generics, do you always need to use them when specifying what kind of interface you are using to create something? Like if I were to say public Set<String> getWords() {}
this obviously says that it will create a method where it takes arguments and places them into a set (different from a list since it has no duplicates) knowing that they are strings, right? But what if we just said public Set getWords() {}
, would this not work? What would it do?
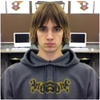
Ian Z
14,584 PointsHi, at 10:46 in video you say this "In fact, I feel almost ill not writing one, and I hope that after we go through a unit testing course, I can cause you to have the same feeling in your gut."
unit testing? is this new course in development? when i google through docs theres no oracle java tutorial pages for unit testing like there are for implementation and extends just lots of "lets set up JUnit" stuff
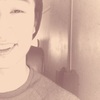
Jason S
16,247 Pointsyou declared that line after the return statement and you need to do it before:
int compareDate = mCreationDate.compareTo(other.mCreationDate);
return compareDate;

Craig Dennis
Treehouse TeacherLooks good, I think you can just return it without creating a variable. Does it work?

ISAIAH S
1,409 PointsNo, the error is:
./com/example/BlogPost.java:50: error: unreachable statement
int compareDate = mCreationDate.compareTo(other.mCreationDate);
^
1 error
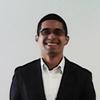
Darwin Palma
3,430 PointsIt remained like this.
public int compareTo(Object obj){
BlogPost blogPost = (BlogPost)obj;
if(equals(obj)){
return 0;
}
int compareCreationDate = mCreationDate.compareTo(blogPost.mCreationDate);
if(compareCreationDate == -1) {
return compareCreationDate;
}
return 1;
}
ISAIAH S
1,409 PointsISAIAH S
1,409 PointsThanks, I deleted
return 1;
in my code and it worked!Anthony Branze
4,887 PointsAnthony Branze
4,887 PointsIm stuck here now too... Totally do not understand what im doing wrong left the return 1; in and this is the error " expected -1 but recieved 1 when comparing sun jul 20 20:18 1969 to mon mar 9 16:00, maybe im just too tired right now..