Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial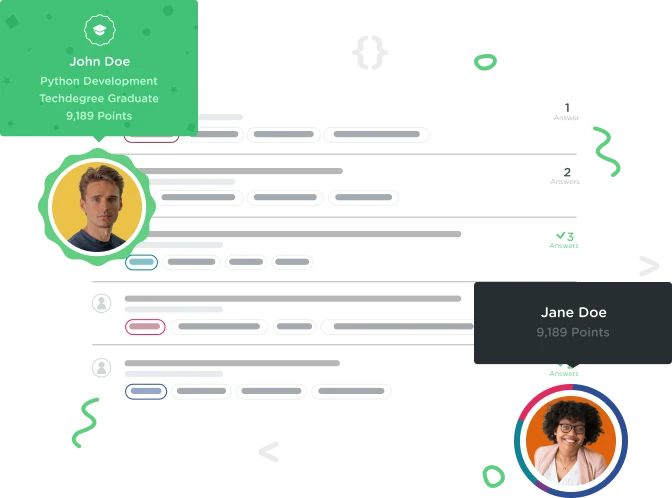
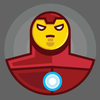
Jess Hardy
12,811 PointsHTML table from JSON store using pure JavaScript, no jQuery
Hello everyone,
Does anyone have a script, tutorial, or example of creating an HTML table from a JSON store using pure JavaScript?
I am working on a project that uses Dojo and we are not allowed to use jQuery. The "grid" systems in Dojo are not responsive, bloated code, and not accessible.
I am now trying to build my own snippet for a sortable, accessible, and responsive table that also supports pagination.
Since I cannot use jQuery,, I will need to use pure JavaScript.
Can anyone help me out.
2 Answers
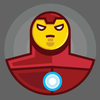
Jess Hardy
12,811 PointsThanks Tony,
I have looked through both of those before, unfortunately 99% of them use jQuery which I'm SOL on.
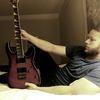
lukebiggerstaff
27,534 PointsFull answer is below.
-Luke
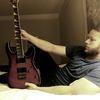
lukebiggerstaff
27,534 PointsHere's a better example of how to do what you want using an AJAX call to retrieve JSON data and vanilla javascript to parse the JSON and create a table and add classes to it that make it mobile first and responsive.
This script would need to be modified based on the format of the JSON information being sent to you. Here's a link to the Gist on GitHub if you want to clone or download it and I'll paste the code below the link.
https://gist.github.com/lukebiggerstaff/9ae4808aedca0d98fc98
//create AJAX function to bind to button click
var getTableDataAJAX = function() {
var getTableData = new XMLHttpRequest();
getTableData.onreadystatechange = function() {
//checking for when response is received from server
if(getTableData.readyState === 4) {
var tableInfo = JSON.parse(getTableData.responseText);
var tableHeading = tableInfo.tableHeading;
var tableCellsPets = tableInfo.tableCellsPets;
var tableCellsPeople = tableInfo.tableCellsPeople;
var createTable = function() {
//Create Table and Heading Rows
var newTable = document.createElement('table');
var tableHeadingRow = document.createElement('tr');
var tableHeader = document.createElement('th');
tableHeadingRow.appendChild(tableHeader);
//Create first row with header and data
var tableRowFirst = document.createElement('tr');
var tableRowFirstHead = document.createElement('th');
tableRowFirst.appendChild(tableRowFirstHead);
for (i = 1; i < tableCellsPets.length; i++) {
//create new heading
var petData = document.createElement('td');
// append Heading to table
tableRowFirst.appendChild(petData);
//set new heading text content to json information
petData.textContent = tableCellsPets[i];
};
var tableRowSecond = document.createElement('tr');
var tableRowSecondHead = document.createElement('th');
tableRowSecond.appendChild(tableRowSecondHead);
for (i = 1; i < tableCellsPets.length; i++) {
//create new heading
var peopleData = document.createElement('td');
// append Heading to table
tableRowSecond.appendChild(peopleData);
//set new heading text content to json information
peopleData.textContent = tableCellsPeople[i];
};
// Add classes to elements
newTable.classList.add('jsTable');
tableHeader.classList.add('jsTableHead');
tableRowFirstHead.classList.add('jsTableRowHead');
tableRowSecondHead.classList.add('jsTableRowHead');
//Add text content and colspan attribute to tableHeader
tableHeader.textContent = tableHeading;
tableHeader.setAttribute("colspan", "4");
//Add text content to row headings
tableRowFirstHead.textContent = tableCellsPets[0]
tableRowSecondHead.textContent = tableCellsPeople[0];
//Append table to DOM
document.body.appendChild(newTable);
//Append rows to new table
newTable.appendChild(tableHeadingRow);
newTable.appendChild(tableRowFirst);
newTable.appendChild(tableRowSecond);
}();
};
};
getTableData.open("GET", "petsandpeople.json", true);
getTableData.send();
};
var wrapperDiv = document.querySelector('div');
var AJAXbutton = document.getElementById('button');
AJAXbutton.addEventListener('click', getTableDataAJAX);
<!DOCTYPE html>
<html lang="en">
<head>
<title>Creating a table using javascript and AJAX</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<div class="wrapper">
<button id="button">Create Table!</button>
</div>
<script src="AJAXTable.js"></script>
</body>
</html>
.wrapper {
text-align: center;
}
.jsTable * {
border: 3px solid black;
text-align: center;
padding: 0;
}
.jsTable {
width: 95%;
margin: 10px auto;
}
.jsTableHead {
font-weight: bold;
font-size: 24px;
background-color: lightgrey;
}
.jsTableRowHead {
font-size: 18px;
background: lightgrey;
}
@media (min-width: 470px) {
.jsTable {
width: 85%;
}
}
@media (min-width: 640px) {
.jsTable {
width: 65%;
}
}
-Luke
qdzfplofsk
22,025 Pointsqdzfplofsk
22,025 PointsJess - this responsive table repo or codepen might help ya.