Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial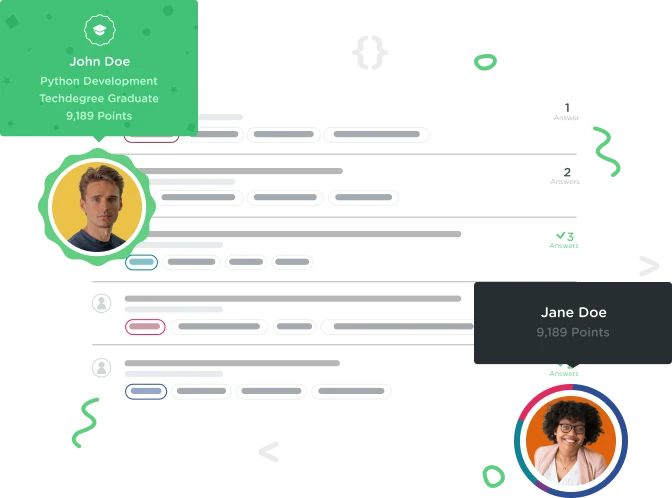
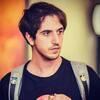
Jonathan Leon
18,813 PointsI finished this code challenge but I'm not sure if I'm correct with my thinking, can anyone take a look? :)
In my mind that's what happens:
obj is passed to the method;
If obj is an instance of BlogPost, it casts it as a BlogPost type, and stores it in the variable blogPost, of the class BlogPost. then, sets the result to the getTitle() string return, and returns the result.
And now is where I got confused...
Cast the type of obj to a String.
If it is now a string(?) set as the result
return result;
The second part really makes me feel I did not fully understand the subject even though I read some documentation and watched the last video several times.
Can anyone clarify this for me please?
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
if (obj instanceof BlogPost) {
BlogPost blogPost = (BlogPost) obj;
result = blogPost.getTitle();
return result;
}
result = (String) obj;
if (obj instanceof String) {
}
return result;
}
}
6 Answers
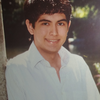
Jon Kussmann
Courses Plus Student 7,254 PointsHi Jonathan,
Your logic for the BlogPost looks good to me. It is actually similar to what you should be doing for String. First check to see if obj is a String:
if (obj instanceof String) {
}
If it is a String, assign it to result:
if (obj instanceof String) {
result = (String) obj;
}
Return that result:
if (obj instanceof String) {
result = (String) obj;
return result;
}
You can combine the two lines like so:
if (obj instanceof String) {
return (String) obj;
}
I hope this helps, please let me know if you have any more questions.
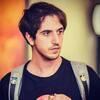
Jonathan Leon
18,813 PointsCould you please try to explain to me how an object could be an instance of String? when I define a variable String string = "string";
string = an instance of string?
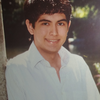
Jon Kussmann
Courses Plus Student 7,254 PointsHi Jonathan,
For most objects, when we want to create a new instance of that object we do SomeObject someObject = new SomeObject();
This is even true for String:
String string = new String("this is my string");
However in Java, there is a shortcut for only Strings... we can do the equivalent with:
String string = "this is my string";
This is why we can check to see if an Object is a String through instance of... it is an object of the String class.
Let me know if you have any more questions.
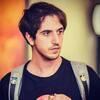
Jonathan Leon
18,813 PointsAlright, thank you very much :)

Dennis Van Laarhoven
988 PointsI still dont understand it. If it is a string then why make it a string? (string) obj -> it already was a string right?
What to do if it is not a string?

Dennis Van Laarhoven
988 Pointsplease explain like i am a little child of 3 years old.
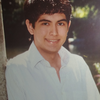
Jon Kussmann
Courses Plus Student 7,254 PointsHi Dennis,
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
if (obj instanceof String) {
return (String) obj;
}
}
I've copied some of the code above for reference. When you call the getTitleFromObject() method, you give the method an Object to perform the method on. Like in real life an Object can be a car, a building, a dog, etc. What we are doing here is checking of that Object (obj) is a String. We don't know in advance what it is (is it a candy or a String?) So what is why we check first. If it is a String, we can return it for this case. If it's something else, say a cup then we would do something like:
if (obj instanceof Cup) {
Cup cup = (Cup) obj;
return cup.getBrand();
}
Where the getBrand() returns a String that is the brand of the cup.

Dennis Van Laarhoven
988 PointsI am sorry i stil dont really understand. 1) if (obj instanceof String) { return (String) obj; ----------------why the word (string) its already a string, so why make it one? }
and i dont get this line: Cup cup = (Cup) obj;
thx for the earlier reply and for this one in advace :-)
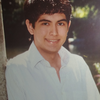
Jon Kussmann
Courses Plus Student 7,254 PointsBefore the method is run, obj is just an Object.
A String is an Object. We do not yet know if obj is a String or something else. All we know is that it's an Object. When we do (String) obj.... we are basically telling our program "This thing called obj is an Object... but I've checked and we can also call it a String"
It's a "small" step, but a necessary one. You might know that it's a String but your program doesn't know it yet until you cast it via (String) obj

Dennis Van Laarhoven
988 PointsAlright, so i am supposed to be smarter then the program :-)
thats gonna bee a though one :-)
Thx very much Jon!