Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial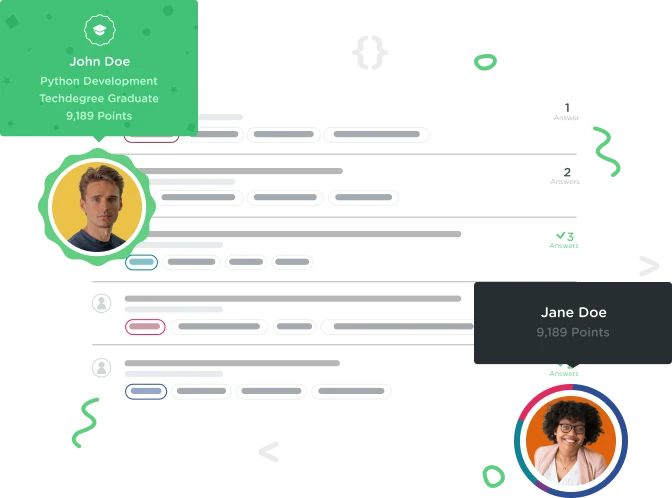

Benyam Ephrem
3,185 PointsI still don't understand the FOR loop?
Can you explain the formatting and logic of the FOR loop a little more, it isn't sinking in for me. This challenge has thoroughly stumped me.
Thank You!!!
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public String getTileCount()
{ int counter = 0;
for (char letter : mHand.toCharArray())
{ if (mHand.indexOf(letter) >= 0)
{
counter++;
}
}
return counter;
}
3 Answers

Ken Alger
Treehouse TeacherBenyam;
Welcome to Treehouse! It is great you are asking questions early on as many of these early subjects are built upon later and the theory/logic behind them caries over into most programming languages.
Let's take a look at a different example of a For Each loop written in English and see if it helps...
Imagine that we have a group of Students, say here at Treehouse. The Treehouse staff wants to know how many student's are from the United States. Prior to computers we would sit down with all of the enrollment forms and search each form, right? We can do that with code using a For Each loop. In English we would say
For each student in the entire group of students do something, in this case look to see their country of origin.
The computer then will look through the group of students, look at each student and if they are from the United States increase a counter, for example, and then report back (return) the total number of students from the US.
Similarly, for the challenge we want to make a function, in this case called getTileCount()
that will look at each tile in our group of tiles, or hand and then return that number. So if our hand has eight tiles with "A C D N N Q O O" and we wanted to know the number of "N" tiles we have, it will loop through each tile and when it finds a matching tile it will increase the counter by 1 and ultimately return the number 2. If we search for an "E" tile, we should expect to get a zero back with the above hand.
Does that make any sense in terms of the logic behind a For Each Loop?
For our challenge the general formatting we are needing to use is, without giving away the entire challenge:
public int getTileCount(char tileToFind) {
int tileCount = 0;
for ( /* looping through each tile */ ) {
if ( /* a tile equals another tile */ ) {
// do something magical
}
}
return tileCount;
}
Post back if you are still stuck on the challenge, or rewatch the video for more specifics on formatting.
Happy coding,
Ken

Craig Dennis
Treehouse TeacherYou should check to see if the letter passed in to the method is equal to the character in your current loop, and only then increment.
Make sense?
Hope it helps!

Benyam Ephrem
3,185 PointsI'm sorry but I'm still stumped. Could you tell me what I've done wrong in my code above. How would you check if an individual letter in the array matches tileToFind? I've tried the .equals method (Isn't that for strings...this a character...?), I've tried the == operators, I've spent hours trying to figure this out and I don't want to give up. What specifically is the logic the computer is following or should be following here? Is my FOR statement wrong, is it the IF statement? Is anything right?
Sorry, I'm new to this so these concepts are kind of hard to grasp.
Thank You so much for the help!!!

Ken Alger
Treehouse TeacherBenyam;
Let's dive a little deeper into this challenge, you are very close I think, just some syntax issues which come with experience and practice. The challenge asks us:
So back to that ScrabblePlayer. I found that it's not enough to know if they just have a tile of a specific character. We need to know how many they actually have. Can you please add a method called
getTileCount
that uses the for each loop you just learned to loop through the characters and increment a counter if it matches? Return the count, please, thanks!
Like I said, it looks like you are on the right path, so let's see where we can tweak your code.
public int .getTileCount(char tileToFind)
{
int tileCount = 0;
for (char tileToFind: mHand.toCharArray())
{
if (mHand.indexOf(tileToFind) >= 0)
{
tileCount++;
}
}
return tileCount;
}
- In your method definition you have a period preceding the name. You want to remove that and make it simply
getTileCount
. That causes a dereferencing error. - In your
for
statement, you already have declared thetileToFind
variable inside the method definition, so that is causing an error. So you would want to create another variable, saysearchingTile
that is also of the typechar
. - In your
if
statement, you should be checking to see if thesearchingTile
is equal to thetileToFind
In this usage we can check if the two are equal with the operator==
.
If we put all the pieces together we would wind up with something similar to:
public int getTileCount(char tileToFind) {
int tileCount = 0;
for (char searchingTile : mHand.toCharArray()) {
if (searchingTile == tileToFind) {
tileCount++;
}
}
return tileCount;
}
I hope this helps more than causes confusion and while some of this is syntax related knowledge that comes through practice, if you still don't have a good grasp on what the above code is doing I would highly encourage you to watch the video(s) again or ask additional questions as loops are an important part of programming.
Happy coding,
Ken

Benyam Ephrem
3,185 PointsOOOOOO.........ok haha I was wondering if I have to create another variable there.
THANK YOU SO MUCH KEN and CRAIG!!!!!!
Benyam Ephrem
3,185 PointsBenyam Ephrem
3,185 PointsI kind of get it now but I still can't do it, can you tell me what I did wrong in this: