Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial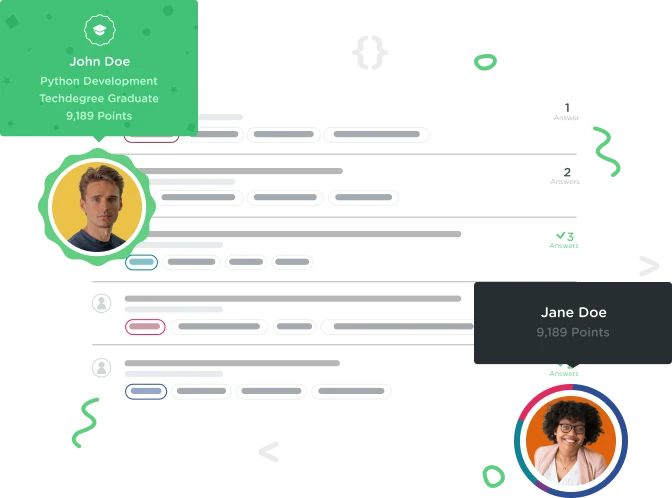

Sohaib Rashid
627 PointsIf someone can help me with this assignment, I would highly appreciate it!
The task as :I have added a method drive that defines a parameter laps. These high-powered GoKarts use 1 energy bar off their battery per lap. Using method signatures, add a new default method called drive that performs just 1 lap and takes no arguments.
Happy Coding!
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void drive() {
int laps = 1;
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers

Ken Alger
Treehouse TeacherSohaib;
Exciting to see you asking questions like this early on. Method signatures and method overloading (using the same method name for different functions) are a powerful feature so understanding them is important. From the Oracle documentation on methods:
Overloading Methods
The Java programming language supports overloading methods, and Java can distinguish between methods with different method signatures. This means that methods within a class can have the same name if they have different parameter lists (there are some qualifications to this that will be discussed in the lesson titled "Interfaces and Inheritance").
Let's take a look at this as it relates to this challenge specifically.
Challenge
I have added a method drive
that defines a parameter laps
. These high-powered GoKarts use 1 energy bar off their battery per lap.
Using method signatures, add a new default method called drive
that performs just 1 lap and takes no arguments.
In looking at the prompting code we see that the current drive()
method takes a number of laps as an argument and inside that method it decreases the battery level by one bar for each lap. If, for example, we wanted to send a kart around the track three times, we would tell it to drive(3)
and it's battery would be depleted by three bars. Hopefully that much makes some sense.
Now, what if in our program we simply want to have our kart go around the track once. We need to do drive(1)
, right? The challenge is asking us to create another method called drive
, thereby overloading that method name. In the end we need to be able to do drive()
and have it be the same as drive(1)
, with me?
To start we can do:
public void drive() {
mBarsCount -= 1;
}
That would do exactly what the challenge is asking, correct? We have overloaded our drive()
method and created a new method that handles one lap while taking no parameters. Great! But wait a minute, what happens if at some point down the road we want to do something else beyond reducing the battery charge level. What if the tires also need to be decreased in some way? The way we have things coded now we would have to change mTireThickness
in both drive()
methods. Not ideal. Perhaps that is what is in the // Other driving code omitted for clarity purposes portion of the original drive()
method.
What to do then? Well, since we already have a drive()
method that takes parameters, and our new drive method is basically using the same signature, we should be able to do something like:
public void drive() {
drive(1);
}
Now we're loggin'! We have two distinct drive()
methods that accomplish our necessary tasks, but really only have to maintain the code in one of them. Now if we add a consideration for tire thickness in drive(int laps)
when we call the drive()
method it will take that into consideration as well.
I hope that made some sense, please post back if it did not.
Happy coding, Ken

Sohaib Rashid
627 PointsThank you Ken! I understand how our new drive method basically uses the same parameter list or signature as the original drive method! Happy Coding and thank you for your help!
HONG ZUO GAN
12,805 PointsHONG ZUO GAN
12,805 PointsThank you Ken !