Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial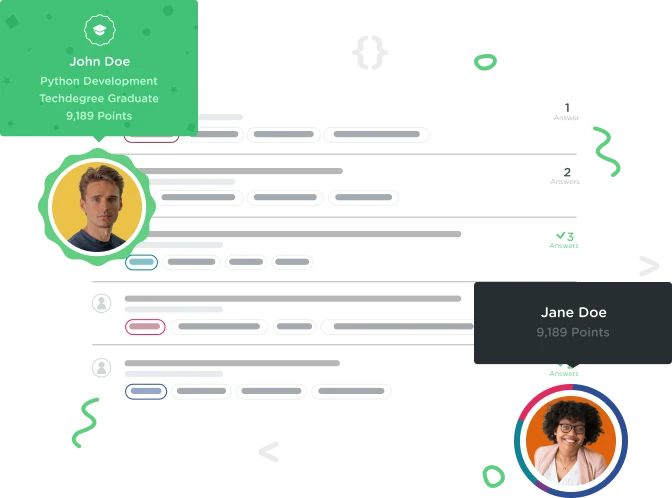
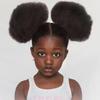
Kyla Thompson
3,831 PointsJava Basics: Knock Knock Joke help
Need help getting pass this exercise. Not sure what I need to do to fix my code:
console.printf("Knock Knock.\n");
String who = console.readLine("Who's there? ");
boolean banana;
do {
console.printf("%s who?\n", who);
banana = (who.equalsIgnoreCase("banana"));
if (banana) {
console.printf("%s who?\n", who);
}
}while (banana);
9 Answers
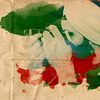
Gunjeet Hattar
14,483 PointsRead the comments and code below. We want to move that prompting code into a do while loop. Wrap the code into a do while and check in the condition to see if who equals "banana" so the loop continues. Remember to move your who declaration outside the do block.
Now if I break down the question
1) First the question asks you to move the promoting code inside a do while loop & your who declaration outside the do block.
String who; // declare who string variable outside who block
do
{
// put promoting code inside do while block
console.printf("Knock Knock.\n");
who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
}
2) Check in the condition to see if who equals "banana" so the loop continues
String who;
do
{
console.printf("Knock Knock.\n");
who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
}
while(who.equals("banana")); // check the condition using Java's equal() method
Try breaking down the question, it helps. And any special reason why you used a boolean variable. It's fine in its way, but you did not initialise the banana boolean in the first place.
I don't like giving away codes, but i just wanted you to get a clear understanding
Hope this helps

Ken Alger
Treehouse TeacherKyla;
Let's walk through this challenge together.
Task 1
Read the comments and code below. We want to move that prompting code into a do while loop. Wrap the code into a do while and check in the condition to see if who equals "banana" so the loop continues. Remember to move your who declaration outside the do block.
Here is the code and comments we are given:
/* So the age old knock knock joke goes like this:
Person A: Knock Knock.
Person B: Who's there?
Person A: Banana
Person B: Banana who?
...This repeats until Person A answers Orange
Person A: Orange
Person B: Orange who?
Person A: Orange you glad I didn't say Banana again?
*/
//Here is the prompting code
console.printf("Knock Knock.\n");
String who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
For task 1 we need to create the do...while loop and meet the task requirements. Let's look at this with some basic problem solving methods (Prepare, Plan, Perform, and Perfect). We'll likely leave the Perfect stage until later.
Prepare
We have watched the video lessons, and have the tools we need, we have the same output for which we are aiming in the code comments.
Plan
Here is what we are asked to do broken down into steps...
- Move the prompting code into a do...while loop.
- Check to see if the variable
who
is equal to banana. - They gave us a hint/tip of moving the
who
declaration outside the do block.
Perform
Our code would then need to look something like:
String who;
do {
console.printf("Knock Knock.\n");
who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
} while(who.equalsIgnoreCase("banana"));
Does that make any sense?
Ken

HONG ZUO GAN
12,805 PointsThanks =)
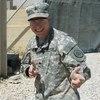
Adam Tatusko
16,589 PointsThose were epic answers to the Code Challenge. :-)
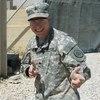
Adam Tatusko
16,589 PointsLuis,
You are declaring the String variable who twice in your code. You can't do that. After you write
String who;
you then only refer to the variable by its name to utilize it or set it equal to a string, e.g.,
who = "Banana";
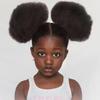
Kyla Thompson
3,831 PointsThanks Gunjeet! This is extremely helpful!
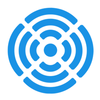
Enrique Bernal
3,584 PointsBummer for me:
JavaTester.java:101: error: variable who is already defined in method run() String who; ^ 1 error
/* So the age old knock knock joke goes like this: Person A: Knock Knock. Person B: Who's there? Person A: Banana Person B: Banana who? ...This repeats until Person A answers Orange Person A: Orange Person B: Orange who? Person A: Orange you glad I didn't say Banana again? */
//Here is the prompting code console.printf("Knock Knock.\n"); String who = console.readLine("Who's there? "); console.printf("%s who?\n", who); String who; do { console.printf("Knock Knock.\n"); who = console.readLine("Who's there? "); console.printf("%s who?\n", who); } while(who.equalsIgnoreCase("banana"));
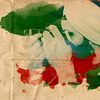
Gunjeet Hattar
14,483 PointsString who = console.readLine("Who's there? "); String who;
You have two instances of the same variable called who. Not possible in Java. You change one to Who or WHO but cannot be the same.

Jim Lamkin
481 PointsThanks Ken!!

bienvenue byishimo
1,153 PointsString who; do { console.printf("Knock Knock.\n");
// Person B asks and Person A's response is stored in the String who: who = console.readLine("Who's there? "); String WHO;
// Person B responds: console.printf("%s who?\n", who); } while (who.equalsIgnoreCase ("banana"));

MUZ140265 Blessing Kabasa
4,996 Pointsfor the first one you can do as follows. String who;
do {
console.printf("Knock Knock.\n");
who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
} while (who.equalsIgnoreCase("banana"));
but for the second task you can use the if statement as follows
if (who.equalsIgnoreCase("orange")){
console.printf("%s i am glad you are not another Banana", who);
}
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherEdited for markup.
Ken