Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial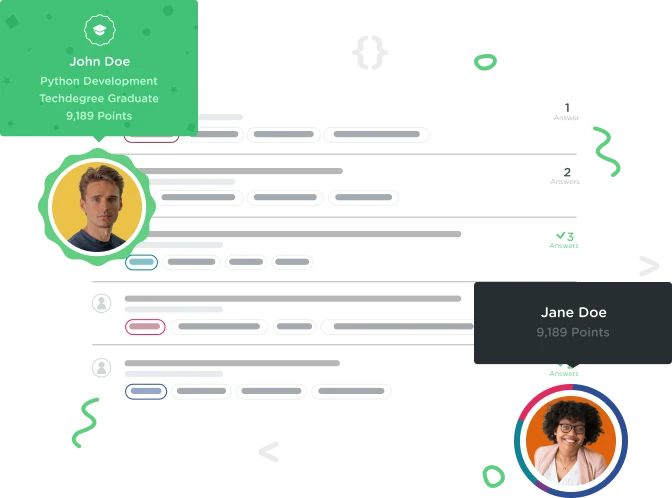

james white
78,399 PointsJava Data Structures, Efficiency, Add Tags to course
Link to challenge:
http://teamtreehouse.com/library/java-data-structures/efficiency/add-tags-to-a-course
Challenge Task 2 of 3
In this task, let's fix the addTag and addTags methods.
My code:
package com.example.model;
import java.util.List;
import java.util.Set;
import java.util.HashSet;
public class Course {
private String mTitle;
private String mTag;
private Set<String> mTags;
public Course(String title) {
mTitle = title;
// TODO: Task 1 of 2 (completed)
// initialize the set mTags
mTags = new HashSet<String>();
}
public void addTag(String tag) {
// TODO: add the tag
mTag = tag;
}
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
List<String> mTags = tags;
}
If gives me the error:
Bummer! I called addTag with "karaoke" and expected the tag to exist in mTags, but it didn't. mTags was: []
So I'm think 'addTag' is right (after adding the needed 'private String mTag;'),
but the string list seems empty?
Does it have to be some kind of loop?
I searched around for some 'public void addTags(' code and found this page:
..which had this code snippet:
public void addTags(List<ITag> gs) {
for (int i = 0; i < gs.size(); ++i) {
this.addTag(gs.get(i));
}
}
I tried to adapt this code to the challenge as such:
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (int i = 0; i < tags.size(); ++i) {
this.addTag(mTags.get(i));
}
}
However, it really didn't like that !
Bummer! There is a compiler error. Please click on preview to view your syntax errors!
The Preview is totally empty though.
So I'm pretty much stymied with part 2 of this challenge..any hints?
The only other existing forum post related to passing part 1 of this challenge (at this point in time is):
https://teamtreehouse.com/forum/set-initialization
I really wish I could ask Ken Alger or William Li.
They probably already finished this challenge by now (after 3 days).
Maybe they (or someone else) will stop by to help, though.
13 Answers

Andre Colares
5,437 PointsHere is my answers for these 3 challenges:
package com.example.model;
import java.util.List;
import java.util.ArrayList;
import java.util.Set;
import java.util.HashSet;
public class Course {
private String mTitle;
private Set<String> mTags;
public Course(String title) {
mTitle = title;
mTags = new HashSet<String>();
// TODO: initialize the set mTags
}
public void addTag(String tag) {
// TODO: add the tag
mTags.add(new String(tag));
}
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String allTags : tags){
mTags.add(new String(allTags));
}
}
public boolean hasTag(String tag) {
// TODO: Return whether or not the tag has been added
if (mTags.contains(tag)){
return true;
}
return false;
}
public String getTitle() {
return mTitle;
}
}

Craig Dennis
Treehouse TeacherYou can add to Set
s using the Set.add
instance method. Since you already have a set, mTags
, this challenge task is asking you to add to the existing set.
Hope it helps!

Luis Santos
3,566 PointsCraig, I don't know what the hell I am doing. I am completely lost at this point. Any advice?

Craig Dennis
Treehouse TeacherHey Luis Santos !
This is a weird weird post, don't let it distract you.
Why don't you make a new post with your question and tag me (@ then my name) and I'll help you get things sorted out. I'd love for this post to disappear ;)
Thanks!
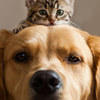
Devin Scheu
66,191 PointsThanks for the help James! I'm coming to get you at number 1 soon!
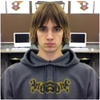
Ian Z
14,584 Points-When youve finished as many courses as you have how do you tell which ones are left?
-Dosent it worry you that all things being equal a veteran could out badge you because things like Crystal ball app and blog reader app are no longer attainable yet their badges dont go away
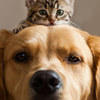
Devin Scheu
66,191 PointsWell, in order to see which courses I have not complete I just scroll trough the library until I spot some. I only have 2 left right now and both show up under my courses in progress so it is not much of a burden now. As for courses being removed, yes it is true, many of the people above me have been there longer and have points that I cannot attain anymore. So I resort to answering questions on forums to catch up and also answer forum posts strengthens my knowledge of the programming languages.
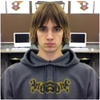
Ian Z
14,584 Pointsthat really sucks, maybe they can mark the courses as retired and still let you access them to get badges? i really wish i could do android crystal ball app course but then took it away

James Simshaw
28,738 PointsHello,
What you're wanting to do in the addTag function is just add the given tag to the set. Which you can then use this function on each item in the list passed into the addTags to add them to the set. Hopefully this will get you closer to solving the Challenge. If you have any further questions feel free to ask.

james white
78,399 PointsPer James' suggestion I tired:
public void addTag(String tag) {
// TODO: add the tag
mTags.add(tag);
}
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String tag : tags)
addTag(tag);
}
}
And got a Bummer! with syntax errors.
..per Craig's suggest of trying addAll, I tied:
public void addTag(String tag) {
// TODO: add the tag
mTags.add(tag);
}
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
mTags.addAll(tags);
}
..and it finally passed (on part 2 of 3 at least).
By the way I got notion to try addAll from this page:
http://www.dotnetperls.com/hashset-java
with this code example:
import java.util.ArrayList;
import java.util.HashSet;
public class Program {
public static void main(String[] args) {
// An ArrayList of three Strings.
ArrayList<String> list = new ArrayList<>();
list.add("socrates");
list.add("plato");
list.add("cebes");
// Add all elements to HashSet.
HashSet<String> hash = new HashSet<>();
hash.addAll(list);
// Use contains.
boolean a = hash.contains("cebes");
System.out.println(a);
// Use containsAll.
boolean b = hash.containsAll(list);
System.out.println(b);
}
}
Thanks to James and Craig for all their help.
I got my 390 points for the full course, which puts me at 56,000 points overall:
http://teamtreehouse.com/jameswhite7
..and am sorta near the top of the Treehouse's all time Points Leaderboard once again:
http://teamtreehouse.com/leaderboards
Oh..and what about part 3 of 3 for the challenge?
.
Luckily (and as Louis Pasteur said "Fortune favors the prepared mind")
now that I've spend days and days researching HashSets,
I knew that there is a HashSet.contains method:
http://www.tutorialspoint.com/java/util/hashset_contains.htm
Here's the sample code from that page:
import java.util.*;
public class HashSetDemo {
public static void main(String args[]) {
// create hash set
HashSet <String> newset = new HashSet <String>();
// populate hash set
newset.add("Learning");
newset.add("Easy");
newset.add("Simply");
// check the existence of element
boolean exist=newset.contains("Simply");
System.out.println("Is the element 'Simply' exists: "+ exist);
}
}
So I figured..let's use that example's boolean line slightly modified:
public boolean hasTag(String tag) {
// TODO: Return whether or not the tag has been added
boolean exists=mTags.contains(tag);
return exists;
}
Can you guess what happened?
By the way - the java code in neither the 'Song.java' or 'SongBook.java' (from the 'S4V6' folder of the zip download for the course) has (or uses) any example of hashset.contains...
I guess now I'll go back to "meditating upon" the Tacocat challenge.
The final (ridiculously involved) challenge for the latest Python Flask Social Network course:
http://teamtreehouse.com/library/build-a-social-network-with-flask

james white
78,399 PointsHi James,
Thanks for the info,
but it is in trying to figure out the code for
use this function on each item in the list passed into the addTags
..that has me stuck.
Exactly HOW do I use such a function?
Thanks in advance for any code hints you can provide.

James Simshaw
28,738 PointsYou can do a "for each" loop in Java like this:
for (Type variableName : collection)
//do something
Which goes through the collection, takes an item and assigns it to the variable variableName of the type Type(ie int, String, etc) and then you can do something on that variable while in that code block.
Have you looked over your addTag() method? It should take the tag in and just add it to the mTags set.

james white
78,399 PointsHi James,
Thanks for the additional guidance,
but my brain is fried in just completing the last challenge of the Java Data Structures course using this forum thread:
https://teamtreehouse.com/forum/bummer-are-you-sure-you-updated-the-videos-title
I'm going to lie down a while and let the smoke stop coming out of my ears..and work on the addTags challenge a little latter..

james white
78,399 PointsI think I'm getting closer, but I keep getting:
Bummer! There is a compiler error. Please click on preview to view your syntax errors!
..and the preview window is totally blank!
Here's the code I'm working with now for 'addTag':
public void addTag(String tag) {
// TODO: add the tag
mTags.add(tag);
}
I still think it's not passing, though, because of my 'addTags' code.
I wish the two "fixes" of part 2 of the challenge had been split!
Anyway, here's some of the code I've tried:
Try#1:
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String tag : tags)
addTag(tag);
}
}
Try#2:
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String mTags : tags)
//here's a real shot in the dark - using .addall
mTags.addall(tags);
}
}
Try#3:
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String mTags : tags)
mTags.add(mTag.add(tags));
}
}
Try#4:
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String mTags : tags)
addTag(mTags);
}
}
Try#5:
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String name : tags)
addTag(name);
}
}
Try#6:
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String mTags : tags)
mTags.add(tags);
}
}
I don't know what else to try..
Is List<String> some kind of weird generic?
http://docs.oracle.com/javase/tutorial/extra/generics/subtype.html

James Simshaw
28,738 PointsHello,
In your addTag method, you want to be adding to the mTags set, not a mTag variable. If you make that change and go with your first attempt for addTags, you should be good to go.

Craig Dennis
Treehouse TeacherHi James,
Why is this a shot in the dark?
//here's a real shot in the dark - using .addall
It's addAll (note the case). You shouldn't need the for loop. It takes a collection as a parameter, so just push in tags.
I think I fixed the problem so you should start seeing the compiler errors on this challenge.
Hope it helps.

Arthur Podkowiak
3,633 PointsWhy is it that you cannot use a for loop for this method? It worked for me with the .addAll
method, but it makes no sense to me why it won't work with the for loop, it's essentially the same thing?
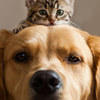
Devin Scheu
66,191 PointsHe's not saying that you can't use a loop, he is just saying you shouldn't need one.
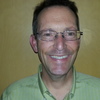
Scott Mobley-Schreibman
7,791 PointsHi James and all,
I would not have gotten through this challenge without your help. Even though these posts are now a couple weeks old, for what it's worth, I just passed Part 3 using the .contains() method with an if statement.
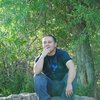
Brody Ricketts
15,612 PointsThis was a fun challenge. I find that my problem most of the time is over thinking what the challenge is asking me to do.
Everyone struggling on this challenge should take a look at Sets.
Even though I am giving you the specific times of the Sets video, its a good idea to watch the FULL video. Even though the video was relatively recent, it never hurts to try and catch something you may have missed.
Challenge 1 is covered at 1:43
Challenge 2 is covered at 2:05. (This is a 2 part challenge, the // TODO gives you the a REALLY good hint on what to do.)
public void addTag(String tag) {
// TODO: ADD the tag
}
public void addTags(List<String> tags) {
// TODO: ADD ALL the tags passed in
}
Challenge 3 I had to do some critical thinking. A small hint, the TODO is asking you if a tag has been added or not, or better yet if the tag is CONTAINED in the mTags HashSet<>();. Using an if statement here is what I did.
public boolean hasTag(String tag) {
// TODO: Return whether or not the tag has been added
return false;
}
- Remember your syntax.
- Remember to check your IMPORTS!
- Remember to check your variable names.
Finally, everything you need to complete these challenges has been presented to you. While outside resources are always great to read up on, the instructors at Treehouse will not let you down or set you up for failure.
Good luck, have fun, and happy coding!
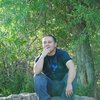
Brody Ricketts
15,612 PointsI did request that they add links to the videos that specifically reference what the tasks of the challenge is asking you to complete.
I think this will help in the long run when challenges ask you to do something that the video prior didn't cover.
A common saying instructors/leaders gave me while I was in the military was - "I wont give you the answer but I will let you know where you can find it."

Natenda Manyau
3,005 PointsThank you!

Phillip Hurst
4,204 PointsI like going thru the videos... but the challenges are really putting me off. Guess I am still to new to programming to be able to put all these different ideas from previous lessons together yet.
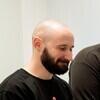
Robert Stamate
13,227 PointsOk so I got the the point where I read this whole thread and I am more confused than before. I followed Brody Ricketts advice but I am stuck on 3/3;
The error: "I called hasTag with "karaoke" and got back false, expected true"
The code:
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String allTags : tags){
mTags.add(new String(allTags));
}
}
What to do? Can someone clarify it or lend me a hand where should I read more about it?
Iheke Iheke-agu
Full Stack JavaScript Techdegree Student 2,307 PointsIheke Iheke-agu
Full Stack JavaScript Techdegree Student 2,307 PointsI got this for the second part if this helps
public void addTags(List<String> tags) { for(String allTags : tags){ mTags.add(allTags); } // TODO: add all the tags passed in }