Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial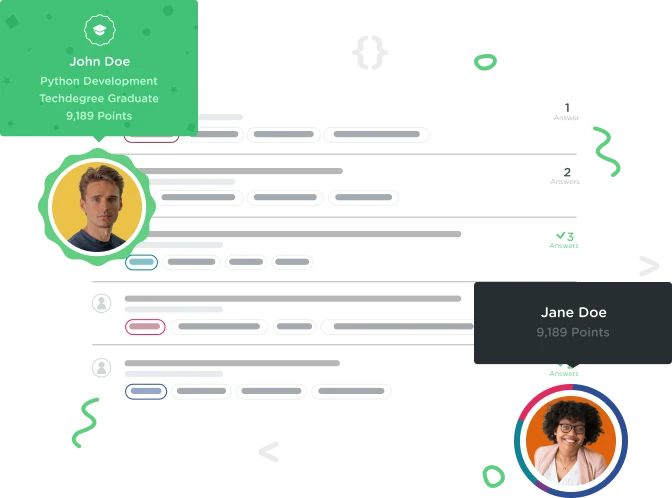
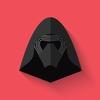
Stu Cowley
26,287 PointsJavaScript Basics: Doing Math Code Challenge, What's Wrong With My Code??
Hey guys,
So I'm doing JavaScript Basics with Dave McFarland and I have hit a snag that I just can't get my head around.
I'm not sure if there is a bug with the code challenge or if my code is actually incorrect.
The Question
Create another variable named profit. It should hold the value of the salesTotal variable minus the wholesalePrice multiplied by the quantity. In other words, if you sold 47 items for 9.99 but only paid 5.45 for each item, how much money did you make?
My Answer
var wholesalePrice = 5.45;
var retailPrice = 9.99;
var quantity = 47;
var salesTotal = retailPrice * quantity;
var profit = salesTotal - wholeSalePrice * quantity;
I look over the code and the question and my answer makes sense to me, apparently not to the code challenge.
I go ahead and click on "Check Work" and then I get that annoying "Oops! It looks like Task 1 is no longer passing." message.
As mentioned earlier, this code makes sense to me and I feel that it should be working, but I have been wrong wrong with saying this before, may need a second set of eyes.
Thanks in advanced
Stu : )
14 Answers

Ken Alger
Treehouse TeacherStu;
Not sure that it matters, but it looks like you changed the variable wholesalePrice
to wholeSalePrice
.
Here is my code through Task 2:
var wholesalePrice = 5.45;
var retailPrice = 9.99;
var quantity = 47;
//Task 1
var salesTotal = retailPrice * quantity;
//Task2
var profit = salesTotal - (wholesalePrice * quantity);
Looks like the code is good with that change. Hard to tell exactly what the Challenge Checker is looking at sometimes.
Ken
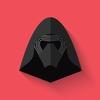
Stu Cowley
26,287 PointsThanks for this Ken Alger, as mentioned to Dave, I must've changed the variable name when I was half asleep haha.
Cheers
Stu : )

Ken Alger
Treehouse TeacherStu;
You need to use parenthesis in your math formula.
If you recall 100 - 5 * 6 is mathematically different from 100 - (5 * 6).
Hope it helps.
Ken
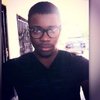
Ladna Meke
5,752 PointsTry this:
var profit = salesTotal - (wholeSalePrice * quantity);
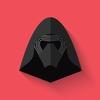
Stu Cowley
26,287 PointsHey Ladna,
This unfortunately does not work. Thanks for your help anyway.
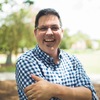
Dave McFarland
Treehouse TeacherHi Stu Cowley
JavaScript is very picky -- variables are case sensitive: the variable wholesalePrice
is not the same as the variable wholeSalePrice
. Check out your code and I think you will see what I mean.

Ken Alger
Treehouse TeacherStu;
I missed that earlier too, that's why it is great to have teachers like Dave McFarland around, even on a holiday!
Ken
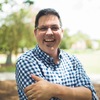
Dave McFarland
Treehouse TeacherHa, ha. Happy Thanksgiving Ken Alger !
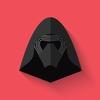
Stu Cowley
26,287 PointsThanks for that Dave McFarland that makes perfect sense, don't know why I didn't see that earlier, I'll have a crack at this later on, and I'll let you know how I go : ).
Thanks for having a look at this for me, especially seeing that it's Thanksgiving!
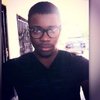
Ladna Meke
5,752 PointsNice! I could have sworn there wasn't any case error. I practically checked like 5 times. Oh well, thanks Dave
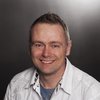
Gøran Smistad
8,807 PointsI got the exact same problem as Stu had. At first, I was quite certain I did not change the variable name in line 1, it just seemed to have changed just by clicking the "Next task" button. However, when I encountered the error message about task 1 failing, I may have changed the variable name without really thinking about it.
I have tried redoing these tasks several times to see if I could recreate the condition that caused the error, and it seems likely that I have actually changed the variable name. But, there is still one problem with the challenge. Having passed task 1, and just declaring the profit variable in task 2, will cause task 1 to fail. Going back to task one with the exact same code, and task 1 will again pass.
I think there is something odd in the code checker, since task 1 should not fail as long at the salesTotal variable is calculated correctly. Or am I wrong? It's quite late here, just as it was for Stu :)
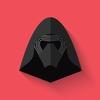
Stu Cowley
26,287 PointsOk guys,
So I have had a crack once again at this challenge, but I am still getting that annoying "Oops! It looks like Task 1 is no longer passing." message coming up when I click on "Check Work"
Here is the code I am using with the help you've all given me.
var wholeSalePrice = 5.45;
var retailPrice = 9.99;
var quantity = 47;
var salesTotal = retailPrice * quantity;
var profit = salesTotal - (wholeSalePrice * quantity);
No doubt I am missing something dumb once again.
Stu : )
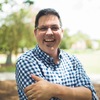
Dave McFarland
Treehouse TeacherSorry about that Stu. It looks like you renamed the original wholesalePrice
variable to wholeSalePrice
in the first line of the code. I think the Code Challenge is analyzing your code and expecting the original variable name wholesalePrice
(with a small s
) not with the capital S
you changed it to.
You basically have the correct answer -- just the variable name needs to match what the challenge is looking for:
var wholesalePrice = 5.45;
var retailPrice = 9.99;
var quantity = 47;
var salesTotal = retailPrice * quantity;
var profit = salesTotal - (wholesalePrice * quantity);
Sorry it's so picky.
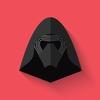
Stu Cowley
26,287 PointsHey Dave McFarland
Thanks for that, I'm not sure why I changed the variable name to be honest, it was quite late when I was doing it so that might explain it, haha.
Just wanted to quickly say thanks for the way that you have done your videos in JavaScript Basics. I have never really been able to get a good grasp on the language but now I am finding that I am : )
Cheers,
Stu :)
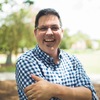
Dave McFarland
Treehouse TeacherGood to hear Stu Cowley! Thanks for the feedback.

jamesoneill
5,167 PointsI just ran into trouble with this task. At first I tried this line of code:
var wholesalePrice = 5.45;
var retailPrice = 9.99;
var quantity = 47;
var salesTotal = retailPrice * quantity;
var profit = retailPrice - wholesalePrice * quantity;
Basically I have written it out in the same way I would work it out on a calculator. Could somebody please explain why the above is different to:
var wholesalePrice = 5.45;
var retailPrice = 9.99;
var quantity = 47;
var salesTotal = retailPrice * quantity;
var profit = quantity * (retailPrice - wholesalePrice);
To me, these two calculations have the same result. Am i missing something here?
Thanks in advance for any help and thanks to Dave for making this course, I think you've done a great job.
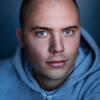
Jonathan Wogenius
1,981 PointsI think the question is formated in a way that many people makes a misstake here, the correct answer is:
var wholesalePrice = 5.45;
var retailPrice = 9.99;
var quantity = 47;
var salesTotal = retailPrice * quantity;
var profit = salesTotal - (wholesalePrice *quantity);
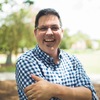
Dave McFarland
Treehouse TeacherHi Jonathan -- interesting point. Do you have a suggestion for way to format the question so it's less confusing?
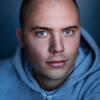
Jonathan Wogenius
1,981 Points"Create another variable named profit. It should hold the value of the salesTotal variable, minus the wholesalePrice multiplied by the quantity. In other words, if you sold 47 items for 9.99 but only paid 5.45 for each item, how much money did you make?"
So I aded the comma. Hehe. Well my native language is Swedish so it might be confusing for me. But I somehow misunderstand it like: var profit = (salesTotal - wholesalePrice) * quantity;
However it might just be me and my Swedish. And of course! Thanks for the best online courses available! :)
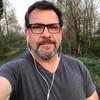
Scott George
19,060 PointsJust got it, wholesale, is one work, so it should be entered as "wholesale". Cool :)
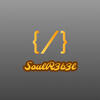
Bruno Carvalho
Front End Web Development Techdegree Student 13,366 PointsI've done it like this
var profit = 9.99 * 47 - 5.45 * 47;
and it works for me !

David Zakeyu
1,535 Pointsvar wholesalePrice = 5.45; var retailPrice = 9.99; var quantity = 47; var salesTotal = 9.99 * 47; var profit = (9.99-5.45)*47

Bashir Orfani
13,170 Pointsvar profit = 9.99 * 47 - 5.45 * 47; this one works.

ROBERT BUTLER
2,010 PointsComplete the variable declaration by adding the keyword that does not allow the value stored in price to change:
var retailPrice = 9.99
Is not the correct answer. It the one below that I just discovered.
____ price = 9.99
const is the variable name.
accountsbestflux
5,021 Pointsaccountsbestflux
5,021 PointsHope this will work
var profit = ( retailPrice - wholesalePrice ) * quantity
also check case sensitive in wholesalePrice