Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial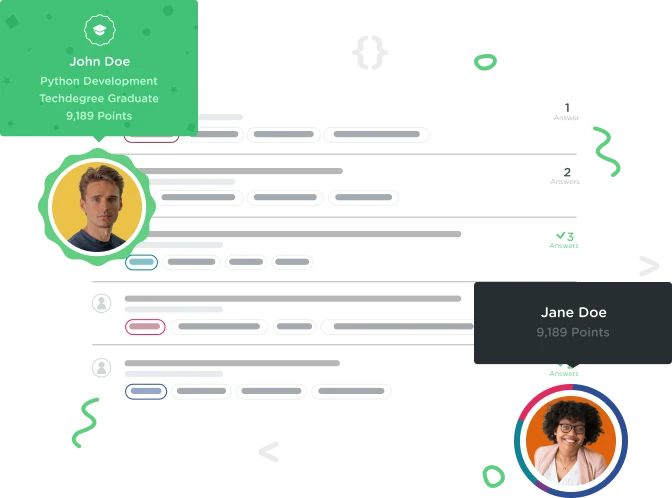

jennyellis
12,231 PointsJavaScript Foundations Arrays Methods - sorting by length?
In JavaScript Foundations Arrays Methods Part 2, the question is: "Sort the 'saying2' array, on about line 19, so that the words are listed in length order. The shortest first. Use the 'length' property on the strings in the sort function."
I think I have to do something with .length but I can't figure out how to write it. This is the last thing I tried: saying2.sort(function (a, b) { return saying2.length() - b; });
Now I'm getting a message that Task 1 is no longer working. I'm stumped and frustrated. Can anyone give me a hint as to what part is wrong?
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Jenny,
Sometimes when it says a previous task is wrong it's usually an error in your current task.
You are correct that the solution is going to involve the .length property.
The sort method is going to take two elements from the array and pass them into the compare function. So a
and b
will hold the values of two of the strings from the array. It's the length of those strings that we want to compare. So you want to use a.length
and b.length
.
The compare function is your way of telling the sort method whether a should come before b, or b needs to come before a, or a and b are equal and the order shouldn't change (this is not guaranteed though, they might be reversed).
Here's the long form of the compare function:
copied from https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort
function compare(a, b) {
if (a is less than b by some ordering criterion)
return -1;
if (a is greater than b by the ordering criterion)
return 1;
// a must be equal to b
return 0;
}
In the first if
condition we can see that we should return a negative number if a is less than b. Since we're sorting by lengths here that translates to a.length < b.length
So if a is shorter than b we'll return a negative number. This lets the sort method know that it has to keep a before b in the final sorted array.
The second if
condition would translate to a.length > b.length
So the final compare function would look like:
saying2.sort(function (a, b) {
if (a.length < b.length)
return -1;
if (a.length > b.length)
return 1;
// a must be equal to b
return 0;
}
So as long as you fill in the correct conditions for the if
's this will always work for you.
There is a shorter way to do it though. If you can find a simple expression that duplicates the functionality of the code above then you can use that instead.
LIke this:
saying2.sort(function (a, b) {
return a.length - b.length;
}
Notice that if a and b have the same length then we'll be returning 0 which is what we want. If a is shorter than b then the expression is going to return some negative number which is exactly what are first if
block is doing in the long form. And if a is longer than b then the expression will return some positive number which matches the second if
block. So this is functionally equivalent to the longer form.
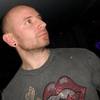
Philip Bradbury
33,368 Pointssaying2.sort(function(a,b) {
return a.length - b.length;
});

Jason Anello
Courses Plus Student 94,610 PointsI knew that was going to happen :)

jennyellis
12,231 PointsOoh. Got it. Thank you! I need to go back and review some JavaScript modules...