Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial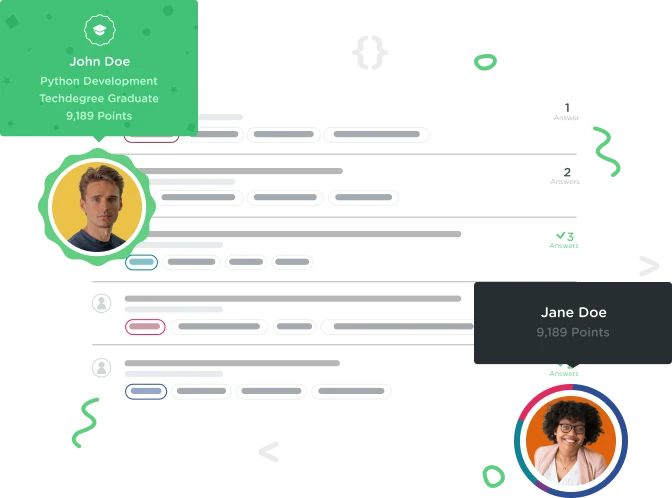
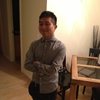
Chris Sicam
4,482 PointsJavaScript Methods help: "On 'andrew' and 'ryan', set the 'greet' method as the 'genericGreet' function".
<script>
var genericGreet = function(person) {
return "Hello, my name is " + this.name;
}
var andrew = {
name: "Andrew"
Andrew.genericgreet();
}
var ryan = {
name: "Ryan"
Ryan.genericgreet();
}
</script>
11 Answers

Adam Fichman
9,502 Pointsyou're almost there bud. Here's what it should look like:
<script>
var genericGreet = function() {
return "Hello, my name is " + this.name;
}
var andrew = {
name: "Andrew",
greet: genericGreet
}
var ryan = {
name: "Ryan",
greet: genericGreet
}
</script>
Alright, so what are we doing differently here? Well, first just some basic syntax errors right away. You'll notice that there needs to be a comma separating the methods name and greet and no semicolon is needed after the last method, in this case, greet.
So what else? Well, the parameter that you have set for the genericGreet function, person, is not needed. Why? Well, when we call either andrew or ryan's greet method, we use the this keyword inside the genericGreet function so that we know that we are calling the name method from within the correct scope.
The other key thing that you're missing here is how you're calling genericGreet. Right now you are calling genericGreet like a method, however it is a function. So what you need to do is set the greet method to genericGreet, as I did above, so that when you call either andrew.greet or ryan.greet it will call the genericGreet function and correctly display the correct string.
Hope I wasn't too confusing!
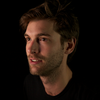
Zachary Rolland
16,465 PointsThe video on methods is pretty complicated, but the challenge is more simple, in a way. That can be confusing sometimes. Thanks for the answers!
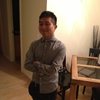
Chris Sicam
4,482 PointsYou're awesome Aaron Cochran . I've never touched JavaScript in my life pre-TeamTreehouse...so this is all very new to me. I'm absorbing it all slowly! Thanks buddy
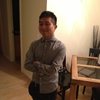
Chris Sicam
4,482 PointsAaron Cochran so I just tried the code you gave me and it didn't work

Adam Fichman
9,502 PointsYou're welcome, and I would look carefully just to make sure you are typing it correctly. I just redid the challenge using the code I gave above and it did work. A lot of times with programming our errors turn out to be very simple ones such as a missed punctuation mark somewhere or an incorrectly spelled variable name.

anthony de alwis
4,146 Pointsdont forget the comma after the name key
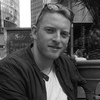
Nicholas Read
2,958 PointsThanks Aaron Cochran, my only mistake was adding the parentheses calling the genericGreet function. So i had
greet : genericGreet()
I'm still a bit confused - I thought when we called a function, we needed the parentheses e.g.
jimGreet();
Why are the parentheses not needed when calling the genericGreet function within the Andrew / Ryan objects??
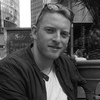
Nicholas Read
2,958 PointsI think I have a answer! genericGreeting is actually a variable that contains a function, not a function in itself, therefore we only need to call the variable, which doesn't need the parentheses. Sound right?

Adam Fichman
9,502 Pointsthat is exactly right! Good job on digging in and finding the answer!
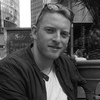
Nicholas Read
2,958 PointsI think I have a answer! genericGreeting is actually a variable that contains a fucntion, not a function in itself, therefore we only need to call the variable, which doesn't need the parentheses. Sound right?

Prabhakar Undurthi
4,846 Pointsvar genericGreet = function() { return "Hello, my name is " + this.name; }
var andrew = { name: "Andrew", greet: genericGreet }
var ryan = { name: "Ryan", greet: genericGreet }
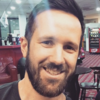
Brad Bucceri
7,102 PointsMy error was missing the commas separating the methods name and greet. Thanks for the explanation after your answer too.
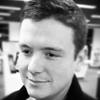
Joshua Bivens
8,586 PointsMe too!
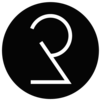
Ritesh Singh
Courses Plus Student 5,503 PointsThanks guys, this helped me too.
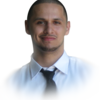
Armando Flores
10,059 PointsAwesome Aaron I thank you for helping me to understand without just giving me the answer!!! Treehouse, this such a great idea to use, that'll help teach us! The whole here is the answer without giving the answer.

Thomas Wright
13,747 PointsI'm not sure why, but it wouldn't work until I did this (adding the "this" to genericGreet):
<script>
var genericGreet = function() {
return "Hello, my name is " + this.name;
}
var andrew = {
name: "Andrew",
greet: this.genericGreet
}
var ryan = {
name: "Ryan",
greet: this.genericGreet
}
</script>
Kaitlin OConnell
3,204 PointsKaitlin OConnell
3,204 PointsGreat explanation, thanks!!
Ryan Bent
6,433 PointsRyan Bent
6,433 PointsHelped me as well!