Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial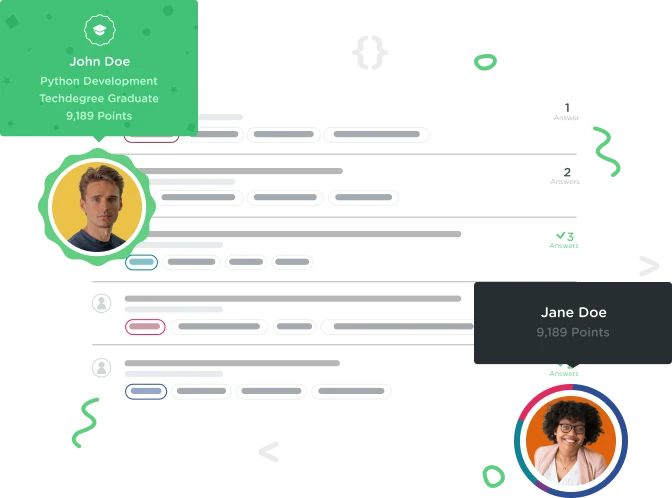

David Lett
7,483 PointsKnockKnock challenge about to give up :(
Can someone tell me what's wrong with my code I've tried passing this several times fixing multiple things without getting it right
import java.io.Console;
public class knockknock {
public static void main(String[] args) {
Console console = System.console();
console.printf("Knock knock. \n");
boolean isInvalid;
String who;
do {
who = console.readLine("Who's there?");
console.printf("%s who?\n", who);
isInvalid = (who.equalsIgnoreCase("banana"));
if (isInvalid) {
console.printf (who);
}
}
}
while (isInvalid);
console.printf("%s who?\n", who);
console.printf("%s you glad i didn't say banana? \n", who);
2 Answers

Ken Alger
Treehouse TeacherDavid;
Welcome to Treehouse!
Don't give up yet! Let's take a look at this challenge task, shall we?
Task 1
Now let's have our code make a joke. Read the code and comments below.
We want to move that prompting code into a do while loop. Wrap the code into a do while code block and check in the while condition to see if who is "banana" so that the loop continues.
HINT: Remember to move your who declaration outside of the do block so that it can be accessed.
// other code removed for brevity
// ====BEGIN PROMPTING CODE====
// Person A asks:
console.printf("Knock Knock.\n");
// Person B asks and Person A's response is stored in the String who:
String who = console.readLine("Who's there? ");
// Person B responds:
console.printf("%s who?\n", who);
// ==== END PROMPTING CODE ====
The task is to implement a do while
loop. You have one in your code, which is great, but let's take a look at what this specific challenge needs. Our loop needs to do
something, while
a specific condition exists. What do we need our code to do
? Ask the question, get the input, and respond. Under what condition? While
the input is "banana". Let's have at it:
do {
console.printf("Knock Knock.\n");
String who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
} while (who.equalsIgnoreCase("banana"));
That moves everything that needs to be done into the do
of the do while
loop, and the while
is that as long as the input equals "banana" it will keep asking the knock knock joke. However, the above code has an error, which is what the HINT in the challenge instructions states, and you remedied in your code as well, nice work!. The above code is defining the variable who
as a String each time it completes the do
statement. Java doesn't like that, it only needs to be told once that who
is a String and as such we need to move the declaration outside the do while
loop. We therefore wind up with:
String who;
do {
console.printf("Knock Knock.\n");
who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
} while (who.equalsIgnoreCase("banana"));
Does that make any sense and help at all? My apologies for being so verbose.
Happy coding,
Ken

Craig Dennis
Treehouse TeacherI've provided the boilerplate for you....its already wrapped in a method, your code looks good without the class and method definition. Your ahead of the class ;)