Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial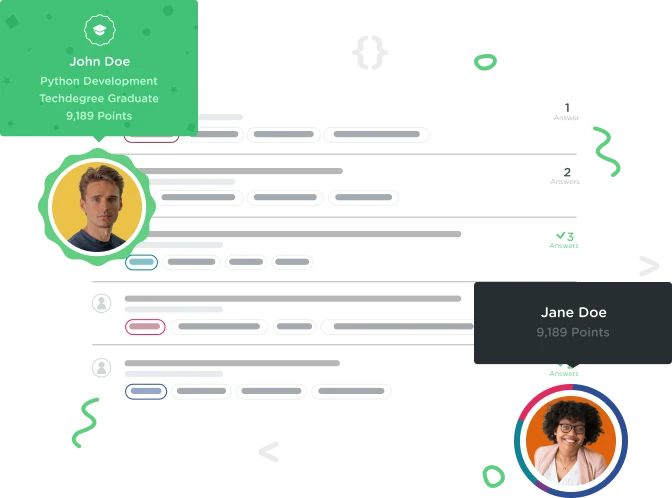
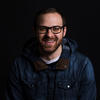
Josh Cummings
16,310 PointsMy Student Record Search Solution
Struggled with this for a little while, but finally came up with a solution for printing multiple student records and alerting the user when a name is not found.
All of the work was done in the while loop. I had to add another conditional statement to the for loop that looks to see if we have reached the end of the loop AND the message variable is blank (meaning no records found). If this is true, it alerts the user that there was no student found.
It then prints the contents of the message variable to the browser and resets the message variable before the while loop starts over.
while (true) {
search = prompt('Type the name of the student to search their records or type "quit" to exit.');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i++) {
student = students[i];
if (search.toLowerCase() === student.name.toLowerCase()) {
message += getStudentReport(student);
} else if (i === students.length -1 && message === '') {
alert('No student found.');
}
}
print(message);
message = '';
}
Feel free to give me some feedback if my code needs tweaking or if there is a more elegant solution.
Also, anyone else notice that Safari returns a blank string instead of null when canceling a prompt?
Another challenge would be dealing with cross-browser issues.

Gabriel Ward
20,222 PointsGreat, thank you Josh. I'm curious, are you a professional developer at all? How much experience do you have with programming?
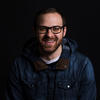
Josh Cummings
16,310 PointsHi Gabriel. I am a freelance web developer in San Diego, CA. I have been dabbling in programming for a few years, but started getting serious about front-end web development in January 2015.

Gabriel Ward
20,222 PointsNice I'm looking at getting into front end development. Trying to work out what I need to do to get into the industry? How long have you been doing it for?

Gabriel Ward
20,222 PointsHi Josh,
Do you have a website?
4 Answers
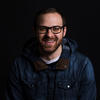
Josh Cummings
16,310 PointsHi Euan,
That line checks to see if we have reached the end of the array AND the message is still blank.
The "i" variable is what we declared in the for loop and represents a sort of "counter" for each iteration through the loop and students.length is the length of our array.
If the script doesn't find a match when iterating through the entire array, it writes nothing to the message variable and therefore displays an alert saying "No student found."
Hope that helps!

Gabriel Ward
20,222 PointsHi Josh, thanks for your helpful solution. I was wondering, could you offer some explanation to (i === students.length -1)?
Am I I correct in thinking that because the array index starts at 0, .length -1 is in fact the last item in the array?
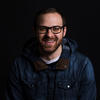
Josh Cummings
16,310 PointsHi Gabriel. Yes, you are correct. Students.length gives us the length of the array, but because arrays are zero indexed, we need to include the -1.

Euan Williams
2,673 PointsCould you explain this line 10 to me please, '''} else if (i === students.length -1 && message === '') {''' Thanks!!
Chuen Hong See
Courses Plus Student 2,693 PointsI think that it means: when for loop reaches the end and message is still blank, pop out the dialog box.

Michal Jankowski
4,051 PointsHey Josh,
Thank you very much for your post.
I was working on this challenge and solved the printing multiple student records problem using the array push() method.
I wrote the almost correct code, but it still didn't work.
I glanced around your code and realized that I forgot to add the "+" mark to my 'message' statetement and it should look like this: 'message += getStudentReport (student);'.
My solution I print below: I added the new variable 'table_report', I used the array push() method in the getStudentReport function and changed the next to last 'message' statement: '+= getStudentReport (student);'.
Josh, you put a smile on my face ;) Thx!
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport (student) {
var table_report = [];
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
table_report.push(report);
return table_report;
}
while (true) {
search = prompt('Please type the name');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search ) {
message += getStudentReport (student);
print(message);
}
}
}

Michal Jankowski
4,051 PointsHello Euan,
Regarding your question: else if (i === students.length -1 && message === '') means that if we reach the end of the array (remember that the array index starting with 0) and if we haven't received any student value matching to the searching value until then, it means that we haven't find any student.
RG Coders
3,990 PointsRG Coders
3,990 PointsNice work, Josh. I looked through your code to learn what I was missing: the "+=" from the following line message += getStudentReport(student);
this allowed me to solve the problem with my twist. Thanks for sharing your solution.