Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial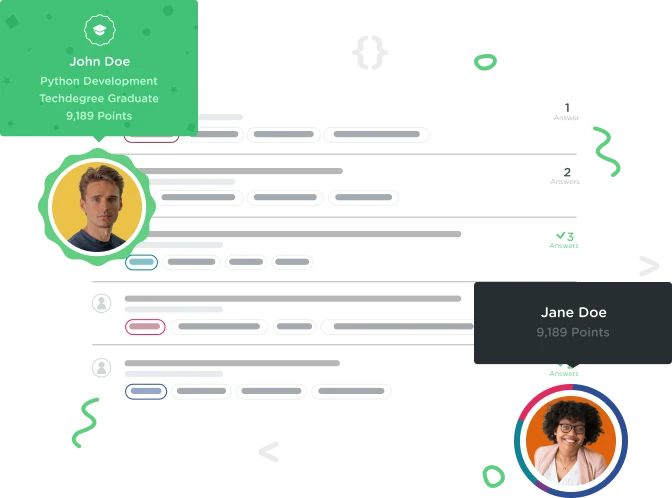

Sujith Vishwajith
8,904 PointsNoMethodError in Statuses#new after installing Devise and setting up sign up page
I get an error saying NoMethodError in Statuses#new for app/views/statuses/form.html.erb:16:in `block in _app_views_statusesform_html_erb2042816324958819823_70362386048980' app/views/statuses/_form.html.erb:1:in `_app_views_statusesform_html_erb2042816324958819823_70362386048980' app/views/statuses/new.html.erb:3:in `_app_views_statuses_new_html_erb_3666238745882068199_70362386271420' in my program. The errors points to my f.first_name, f.last_name, and f.profile_name. If i delete these lines it seems to work fine but i need them continue.
<h2>Sign up</h2>
<%= form_for(resource, :as => resource_name, :url => registration_path(resource_name)) do |f| %>
<%= devise_error_messages! %>
<div><%= f.label :first_name %><br />
<%= f.text_field :first_name %></div>
<div><%= f.label :last_name %><br />
<%= f.text_field :last_name %></div>
<div><%= f.label :profile_name %><br />
<%= f.text_field :profile_name %></div>
<div><%= f.label :email %><br />
<%= f.email_field :email, :autofocus => true %></div>
<div><%= f.label :password %><br />
<%= f.password_field :password %></div>
<div><%= f.label :password_confirmation %><br />
<%= f.password_field :password_confirmation %></div>
<div><%= f.submit "Sign up" %></div>
<% end %>
<%= render "devise/shared/links" %>
My Routes:
Treebook::Application.routes.draw do
devise_for :users
resources :statuses
root to: 'statuses#index'
end
19 Answers

Nick Fuller
9,027 PointsIf you open the db/migrate directory there are 2 things that aren't good in here.
First it looks like you've accidentally created a devise model called View
. See the third file down called 20140220021440_devise_create_views.rb
? It looks like you accidentally created this model go ahead and run this command in terminal:
rails destroy devise View
Next, and this is the one that is causing you grief!
Look at your first migration in the file 20140218220021_create_statuses.rb
. Here you are creating the statuses table and giving it the columns :name and :context. This is good, leave this file alone.
Now look two files down in 20140219055415_add_user_id_to_statuses.rb
. There is a line of code in there that is really hurting you! Once you find this line of code, delete the line of code, not the file!
Once you have done all this, reset your database
bundle exec rake db:drop
bundle exec rake db:create
bundle exec rake db:migrate
Then try it out!

Nick Fuller
9,027 PointsI'm assuming this is for a User model?
Did you create a migration to add the first_name, last_name and profile_name columns to the database?

Sujith Vishwajith
8,904 PointsWhat do you mean by create a migration? do you mean use the rake db:migrate command?

Nick Fuller
9,027 PointsI have not done the Treebook series so I'm not sure how you created the fields on the User model
When you created the User it would have been something like
rails generate devise User first_name:string last_name:string profile_name:string
Did you use that when you created the model?
Can you copy and paste your migration file from when you created the user table? It would be in the directory like db/migrate/XXXXXXXXXX_create_user.rb

Sujith Vishwajith
8,904 PointsI tried doing that but I cannot run rake db:migrate anymore as i get the error: == AddDeviseToUsers: migrating =============================================== -- change_table(:users) rake aborted! An error has occurred, this and all later migrations canceled:
SQLite3::SQLException: duplicate column name: email: ALTER TABLE "users" ADD "email" varchar(255) DEFAULT '' NOT NULL/Users/sujith/Desktop/Programs/Rails/treebook/db/migrate/20140220055902_add_devise_to_users.rb:5:in block in up'
/Users/sujith/Desktop/Programs/Rails/treebook/db/migrate/20140220055902_add_devise_to_users.rb:3:in
up'
Tasks: TOP => db:migrate
(See full trace by running task with --trace)
here is my create_user.rb
class DeviseCreateUsers < ActiveRecord::Migration
def change
create_table(:users) do |t|
t.string :first_name
t.string :last_name
t.string :profile_name
## Database authenticatable
t.string :email, :null => false, :default => ""
t.string :encrypted_password, :null => false, :default => ""
## Recoverable
t.string :reset_password_token
t.datetime :reset_password_sent_at
## Rememberable
t.datetime :remember_created_at
## Trackable
t.integer :sign_in_count, :default => 0, :null => false
t.datetime :current_sign_in_at
t.datetime :last_sign_in_at
t.string :current_sign_in_ip
t.string :last_sign_in_ip
## Confirmable
# t.string :confirmation_token
# t.datetime :confirmed_at
# t.datetime :confirmation_sent_at
# t.string :unconfirmed_email # Only if using reconfirmable
## Lockable
# t.integer :failed_attempts, :default => 0, :null => false # Only if lock strategy is :failed_attempts
# t.string :unlock_token # Only if unlock strategy is :email or :both
# t.datetime :locked_at
t.timestamps
end
add_index :users, :email, :unique => true
add_index :users, :reset_password_token, :unique => true
end
end

Nick Fuller
9,027 PointsWhat does your statuses controller look like?

Sujith Vishwajith
8,904 Pointshere is my statuses controller
class StatusesController < ApplicationController
before_action :set_status, only: [:show, :edit, :update, :destroy]
# GET /statuses
# GET /statuses.json
def index
@statuses = Status.all
end
# GET /statuses/1
# GET /statuses/1.json
def show
end
# GET /statuses/new
def new
@status = Status.new
end
# GET /statuses/1/edit
def edit
end
# POST /statuses
# POST /statuses.json
def create
@status = Status.new(status_params)
respond_to do |format|
if @status.save
format.html { redirect_to @status, notice: 'Status was successfully created.' }
format.json { render action: 'show', status: :created, location: @status }
else
format.html { render action: 'new' }
format.json { render json: @status.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /statuses/1
# PATCH/PUT /statuses/1.json
def update
respond_to do |format|
if @status.update(status_params)
format.html { redirect_to @status, notice: 'Status was successfully updated.' }
format.json { head :no_content }
else
format.html { render action: 'edit' }
format.json { render json: @status.errors, status: :unprocessable_entity }
end
end
end
# DELETE /statuses/1
# DELETE /statuses/1.json
def destroy
@status.destroy
respond_to do |format|
format.html { redirect_to statuses_url }
format.json { head :no_content }
end
end
private
# Use callbacks to share common setup or constraints between actions.
def set_status
@status = Status.find(params[:id])
end
# Never trust parameters from the scary internet, only allow the white list through.
def status_params
params.require(:status).permit(:name, :context)
end
end

Nick Fuller
9,027 PointsSo, Sujith I may have confused myself help me understand really quick.
The url you are viewing in the browser is localhost:3000/statuses/new, correct?
But the html.erb form that you have linked on the original post, where is that from? What directory is it in? That looks like it has to do with a user, not a status.

Sujith Vishwajith
8,904 PointsYes but after running the command you mentioned earlier, I can no longer go to the homepage or run rake db:migrate due to the error
rake aborted!
An error has occurred, this and all later migrations canceled:
SQLite3::SQLException: duplicate column name: email: ALTER TABLE "users" ADD "email" varchar(255) DEFAULT '' NOT NULL/Users/sujith/Desktop/Programs/Rails/treebook/db/migrate/20140220055902_add_devise_to_users.rb:5:in `block in up'
/Users/sujith/Desktop/Programs/Rails/treebook/db/migrate/20140220055902_add_devise_to_users.rb:3:in `up'
Tasks: TOP => db:migrate
(See full trace by running task with --trace)

Sujith Vishwajith
8,904 Pointshere is a link to the github repo: https://github.com/sujithv28/treebook

Nick Fuller
9,027 PointsAh Sujith, I'm sorry I didn't want you to run the command. I was asking you if that was the command you ran before. But, to remove that error run this
rm /Users/sujith/Desktop/Programs/Rails/treebook/db/migrate/20140220055902_add_devise_to_users.rb
Then try your rake db:migrate

Sujith Vishwajith
8,904 PointsThanks that command worked but I am still back to the same error. Everything runs fine but i get the error i first mentioned when i navigate to /users/sign_up. The new code is pushed to git. Sorry, I am kinda new to Rails.

Nick Fuller
9,027 PointsDon't be sorry! I feel bad for sending you down a bad path. This is part of learning :)
I'm forking your repository i'll pull it down 1 minute

Nick Fuller
9,027 PointsI pulled down your repository, the page loads just fine for me

Sujith Vishwajith
8,904 Pointsreally? I get an error when i open up /users/sign_up. I tried restarting my rails server but it didnt seem to help. Do you have any recommendations for me to try?

Nick Fuller
9,027 PointsI have found the problem!

Sujith Vishwajith
8,904 PointsTHANKS SOO MUCH! I guess i was removing the column after I added it. Just out of curiosity where did you learn ROR if you didnt use treehouse. Did you do onemonthrails?

Nick Fuller
9,027 PointsI actually started using rails about 3 years ago. It's what got me into coding :) I love the convention > configuration philosophy, MVC, and ruby is just oh so fun to code! I actually didn't do the rails treehouse courses because I didn't know about them at the time; however, I would have if I did! I am 85% self taught and 15% mentored by someone I greatly respect whom I am lucky to work with.
I use Treehouse because my html, css, design and wordpress skills are not very good :(
But I really enjoy reading the forums and helping people with Ruby and Rails questions when I can!
Sorry it took so long, I felt bad for sending you down a bad route there. But, there are two MAJORLY important lessons from this:
ALWAYS use source control. See how easy it was to fix the problem? github is an amazing tool and you should always use it! Heck I even have my wife's homework for her masters degree in source control!
NEVER give up! You will get down, you will get frustrated, you will want to bang your head against the desk.. and when that time comes... take a 10 minute break (I play video games to clear my head) then come back to it. Because the solution is always there, it doesn't matter how you find it, but you will find it as long as you don't give up!
I don't want to advertise anything here, but I will recommend this book... I have zero affiliation with the author or publisher... but I learned SO MUCH from this book. It's my favorite Ruby book and I have great respect for the author

Sujith Vishwajith
8,904 PointsThanks a lot for the pointers, I'll make sure to check out the book after my SAT's. I started off with Java and HTML, so I am familiar with OOP but not so much with Ruby's syntax. Thanks again and have fun with rails!