Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial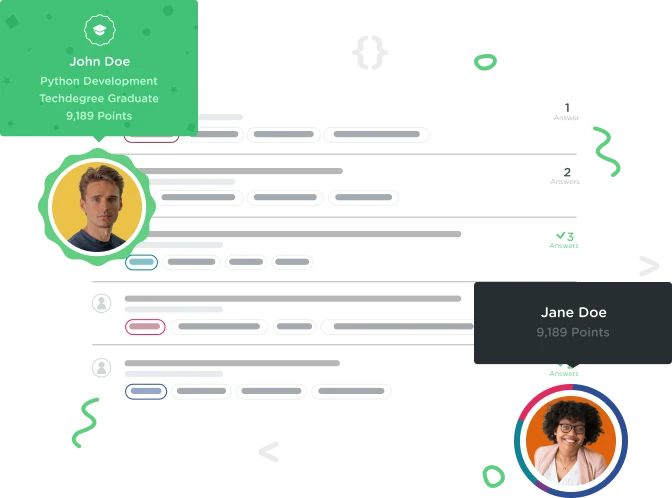

Jason Zeall
7,860 PointsNot sure on the Tuple Question (iOS - Swift). To modify the greeting to return both language and greeting.
"Currently our greeting function only returns a single value. Modify it to return both the greeting and the language as a tuple. Make sure to name each item in the tuple: greeting and language. We will print them out in the next task."
4 Answers

Chris Shaw
26,676 PointsHi Jason,
So if you remember back to the previous video tuples are constructed using opening and closing parentheses along with comma separated values inside them, for example.
let myVar = (10, 20, 30)
So what does the above actually do?
Well it creates a tuple we can extract values from using the dot notation, by default unnamed tuples carry indexes whereas if we name them we access the values using the name we give them.
For example.
let myVar = (10, 20, 30)
let aValue = myVar.0 // results in 10 as 10 is the zeroth value in the tuple
How do we apply this to the task?
Very simply, we know that we can declare any return type and a value of that chosen type so we just need to take that and apply it to the greeting
function.
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
// greeting("John").greeting = "Hello John"
// greeting("John").language = "English"
So here we're using named tuples which is what the challenge is asking for but remember that they don't have any requirement to have names therefore we could even write the following but it wouldn't pass the challenge.
func greeting(person: String) -> (String, String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
// greeting("John").0 = "Hello John"
// greeting("John").1 = "English"
There's also one other thing to know and that is named tuples don't have to be set in the order of the return type, for example.
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (language: language, greeting: greeting)
}
This would result in the same outcome as the first code example above as our return type has the order set therefore they will appear in that order even though our return
statement is backwards.

Matthew Chana
1,063 PointsThis challenge was pretty different from the example in the video so I found it to be confusing. Great answer but the example and how it aligns to the challenge are very poorly done.

Dave Abalos
6,976 PointsI was having the same problem for couple of hours, did return watching the videos but couldn't make it right.
I was very skeptical thinking that I can only do {Bool, Strings //with the functions} That's why I didn't included the 'greeting' since it's String... I was fooled!
I just learnt that I can also do {Strings, Strings}
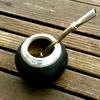
Cole Enabnit
3,414 PointsThank you SO much for this answer.
Jason Zeall
7,860 PointsJason Zeall
7,860 PointsHey Chris,
I think this is most awesome answer and reply I have seen. Thank you for putting in the time.
Absolutely awesome.
Richard Nash
24,862 PointsRichard Nash
24,862 PointsGreat work Chris Upjohn :-) The question itself, as far as I can tell, is rather non-sensical, as I cannot think of a real world situation where this kind of query would realistic and useful. Because of this, trying to figure out how to do it is difficult, because the intent is foggy. This, of course, could simply be my ignorance, so if you have a real world example where I would want to pass a name and get back the greeting, as well as the language that has been set as a variable, then please help me out. It just seems odd to me, which makes it difficult to complete the function, at least for me.
Chris Shaw
26,676 PointsChris Shaw
26,676 PointsHi Richard,
The above is strictly related to the challenge so it's not a real world of example of how you would write a function using tuples as the return structure but it gives you insight into the syntax required to do so, the example as a whole was written in a way that makes this syntax easy to understand while at the same time might cause confusion considering it doesn't serve any real world purposes.
Kevin Frostad
3,483 PointsKevin Frostad
3,483 PointsHelped me out a lot! Thank you.
Dallas Matthews
1,099 PointsDallas Matthews
1,099 PointsThank you for the time and effort you put into this response! It cleared up the challenge and Tuples a lot for me.