Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial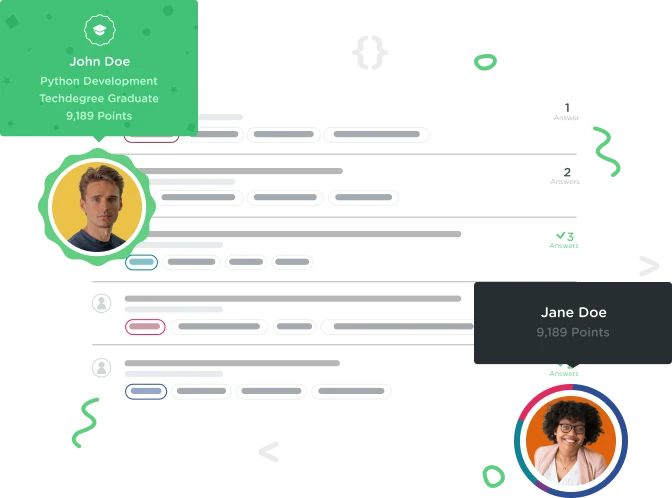
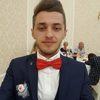
Argzon Haziraj
Courses Plus Student 14,994 PointsNow make a function named random_num that takes an int argument. Return a randint between 1 and the passed-in number.
I can't get of this challenge !! What i actually have to do ? Somebody explain it !!
3 Answers

Charlie L.
10,120 PointsMake a function using the "def" keyword. An int argument is any number that's not a float or string value. This function is to return a randint value which is short for random integer.
If any of these terms don't make sense, I would strongly encourage you to go back in previous lessons and re read before progressing.
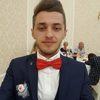
Argzon Haziraj
Courses Plus Student 14,994 PointsCharlie L. Thank you for your answer. I did the challenge now. Here is my code.
import random
bledari = 1
def random_num(bledari):
random.randint(1,bledari)
return bledari

Charlie L.
10,120 PointsThat's good progress! Alright now lets see what your code is doing right now.
import random
bledari = 1
You made a variable named "bledari" and assigned it a value of 1.
def random_num(bledari):
random.randint(1,bledari)
return bledari
You made a function named "random_num" that accepted your variable "bledari." Then you used random.randint(1,bledari). Now this seems a bit off. The randint method takes two arguments (at minimum) which are randint(start, end) and returns a value between the start and end value. In your code, you are starting at 1 and ending at 1. That's no good at all. You want the ending value to be the passed value. So let's backtrack all the way back to the beginning of the function. You want this function to take ANY number so instead of using a variable defined by you, we'll just use some placeholder variable like "some_num." Here's what I have:
import random
def random_num(some_num):
return random.randint(1, some_num)
The above code defines a function "random_num" that accepts one argument (hopefully an integer) and that argument "some_num" is used within the randint method.
So now if we wanted a random number between 1 and 6 we can just use:
print(random_num(6))
Wow this post ran a bit long but I hope it clears things up!
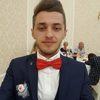
Argzon Haziraj
Courses Plus Student 14,994 PointsThanks a lot Charlie L. . That's all i wanted to know and to understand. It's clear now :)