Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial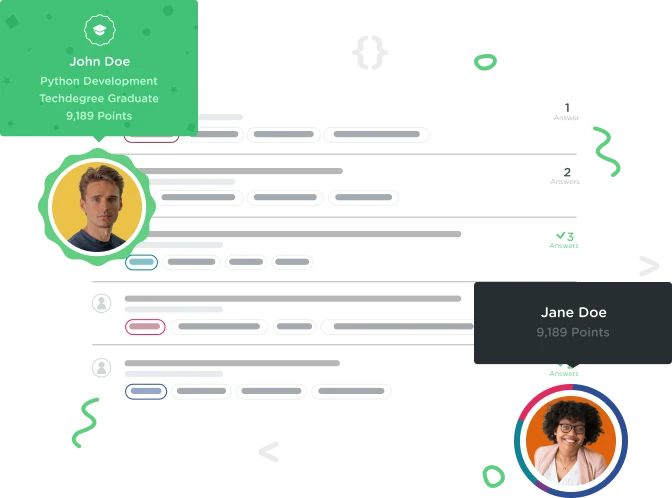

CHIRAG SARAOGI
1,280 PointsPassing object as a parameter
When creating a new object of ForumPost, I cannot figure out how to pass the author name of type user. it is the first parameter passed while creating a new object of type ForumPost
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public ForumPost(User author, String title, String description)
{
mAuthor = author;
mTitle = title;
mDescription = description;
}
public String getDescription()
{
return mDescription;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName, mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName()
{
return mFirstName;
}
public String getLastName()
{
return mLastName;
}
}
public class Forum {
private String mTopic;
public Forum(String topic)
{
mTopic = topic;
}
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
User author = new User("Chirag","Saraogi" );
ForumPost post = new ForumPost(author,"love", "yayy");
forum.addPost(post);
}
}
2 Answers
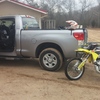
Ryan Ruscett
23,309 PointsHey,
Thanks for requesting me to answer this. You are soo close. Just missed a few keywords there.
The description it is giving is "I expected to see the names I passed in through the args" , but I didn't pass anything into the agrs. At least not that I know of. Take at look at this sneeky section.
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
It's appears to be checking for arguments that are coming into the main method. Well, I am not supplying those so the teacher must be. This tells me that it's checking for two arguments being passed into the main. This we can't see which makes this really tuff. So when I create a user, I need to make a user of not myself. But as the agruments being passed in. I need to access these arguments when I create a user.
User author = new User(args[0], args[1]);
See, now the user is being created with the passed in arguments. So change that one line in your Example.java and it should work.
Please let me know if you have additional questions I can try to clear up for you.
Thanks!
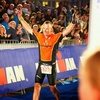
Steve Hunter
57,712 PointsHi there,
It isn't very clear, I don't think, but the name has been passed in using the args[]
of main
.
So, if you change your code:
User author = new User("Chirag","Saraogi" );
To pick up the first two args[]
like this:
User author = new User(args[0], [args[1]);
That should work for you!
Steve.

CHIRAG SARAOGI
1,280 PointsHey, Thanks a lot for taking out time to answer my question, I truly appreciate it. It really helps to understand it is important to pass arguments to the main when creating a new user which will help the user to enter his first name and last name. There is one more thing which I need clarity towards.
public ForumPost(User author, String title, String description) { mAuthor = author; mTitle = title; mDescription = description; }
this is my ForumPost constructor. Now when creating a new object what should I pass as parameters ForumPost post = new ForumPost();
The error requires me to enter an author of type user, title and description.
Regards Chirag Saraogi.
CHIRAG SARAOGI
1,280 PointsCHIRAG SARAOGI
1,280 PointsHey, Thanks a lot for taking out time to answer my question, I truly appreciate it. It really helps to understand it is important to pass arguments to the main when creating a new user which will help the user to enter his first name and last name. There is one more thing which I need clarity towards.
public ForumPost(User author, String title, String description) { mAuthor = author; mTitle = title; mDescription = description; }
this is my ForumPost constructor. Now when creating a new object what should I pass as parameters ForumPost post = new ForumPost();
The error requires me to enter an author of type user, title and description.
Regards Chirag Saraogi.
Ryan Ruscett
23,309 PointsRyan Ruscett
23,309 PointsHey Chirag,
The data you send to the constructor is defined in ForumPost. ForumPost constructor wants 3 items. ForumPost can not be created without receiving 3 peices of data. When you receive an argument. You want to know what type of argument you are going to be getting.
You wouldn't give someone 1000 dollars without knowing what you were going to get back.
public ForumPost(User author, String title, String description)
The ForumPost defines what it needs. We have no choice but to give it what it needs if we wish to use this class.
The constructor has told us it wants a User data type, and two strings. The user you created by accepting the arguments being passed to the main.
User author = new User(args[0], args[1]);
Now you have a user object. The first piece of the ForumPost Constructor. Next we need a title and a description.
We do not set title and or description anywhere else in any other class. So it's pretty much up to use to use whatever we would like, so long as it's a string data type.
ForumPost post = new ForumPost(author, "Title whatever I want", "Give a description of my choice");
This creates a ForumPost.
Does this help? Let me know!
CHIRAG SARAOGI
1,280 PointsCHIRAG SARAOGI
1,280 PointsHey, This helped a ton. Its silly of me to not look deeper to understand what its asking. Slowly getting a grasp of it.
Thanks a lot Have a great day.