Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial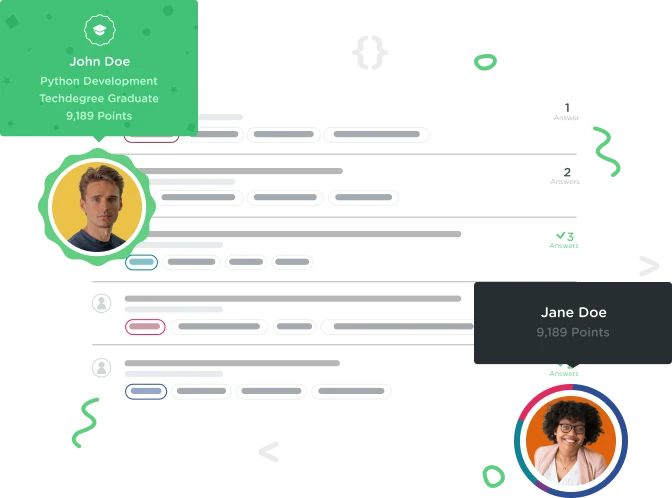
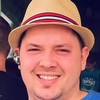
Allen Lamphear
Courses Plus Student 6,660 PointsPassing Variable To Ajax Into A Modal
I was trying to figure out how to pass a variable to a model. For example, I have a table that lists phone inventor. I want to click one of them and have a modal popup that is matching that phone. So I obviously need to pass some how the id in the table to the model. This is all in PHP. And then after having that model popup I would like to edit and resubmit which then would refresh the table. Perhaps even have a success message. I am using the bootstrap so I was thinking of using the alert to say Successfully Updated! or something.
Any help would be greatly appreciated. Thanks in advanced!
Btw, this is what my jquery looks like
$(function() {
$("button#view").click(function(){
var param = 1;
var data = 'param'+param;
$.ajax({
url: "getPhone.php",
data: data,
success: function(msg){
$("#modal-results").html(msg)
$("#myModal").modal('hide');
},
error:function(){
alert("failure");
}
});
});
});
The $("#modal-results").html(msg)
is where the div would be that would show the alert. Which I had above the table, so when the submit the alert would popup above the table.
10 Answers

Gregory Benner
5,732 PointsThe data would be best served from a html form incase js is off. Also the data should be serialized. Use e.preventDefault(); to stop form from default of submitting on click then the code you have is close. Here's an example http://stackoverflow.com/questions/18094816/how-to-get-the-response-data-in-ajax-call

Gregory Benner
5,732 PointsThe data would be best served from a html form incase js is off. Also the data should be serialized. Use e.preventDefault(); to stop form from default of submitting on click then the code you have is close. Here's an example http://stackoverflow.com/questions/18094816/how-to-get-the-response-data-in-ajax-call
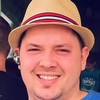
Allen Lamphear
Courses Plus Student 6,660 PointsThank you for your response. I think I might be thinking wrong or I am saying it wrong. I can post a form from a modal through ajax. But when I am trying to do is get information into the modal.
So say my table has a button for each entry. Lets call it "View" well this button has and ID of "viewPhone". When I click that it would open up a modal that would populate information based on the entry id. (ie. $row['id'];) (which would be 1) In that modal it has everything filled out already because it is echoing out the information based on the id.
This is what I am trying to say about passing a variable to ajax, so that modal would not post but get. I don't know if that makes sense or not.
Thank you again for the response..

Gregory Benner
5,732 PointsAhh well everything in your code is correct apart a missing semicolon after "$("#modal-results").html(msg) " What returns into msg? Double check the console in chrome, you can also see the network tab in chrome dev tools and see the request is made and the result. You are writing the javascript correct as long as the form is returning a response on the PHP side of things.
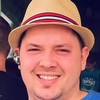
Allen Lamphear
Courses Plus Student 6,660 Pointshmm... maybe i am missing something. I'll post some stuff. Btw, I have set a default id as 1 in ajax but I need it to pull from the table id.
<td><a href="getPhone.php?id='.$row['id'].'" class="btn btn-xs btn-primary" id="view" data-toggle="modal" data-target="#myModal">View</a></td>
that is my button link
<div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
<h3 class="modal-title" id="myModalLabel"><b>Phone Profile - ID <?= $id ?></b></h3>
</div>
<div class="modal-body">
<form class="test" role="form">
<div class="form-group">
<label for="phone_number">Phone #
<input type="text" class="form-control" id="phone_number" name="phone_number" value="<?= $phoneNumber ?>"></label>
<label for="device_id">Device ID
<input type="text" class="form-control" id="device_id" name="device_id" value="<?= $deviceID ?>"></label>
<label for="device_manufacturer">Device Manufacturer
<input type="text" name="device_manufacturer" id="device_manufacturer" class="form-control" value="<?= $deviceMan ?>" /></label>
<label for="device_model">Device Model
<input type="text" name="device_model" id="device_model" class="form-control" value="<?= $deviceModel ?>"/></label>
<label for="phone_alias">Phone Alias
<input type="text" name="phone_alias" id="phone_alias" class="form-control" value="<?= $palias ?>"/></label>
<label for="chauffeur_number">Chauffeur #
<input type="text" name="chauffeur_number" id="chauffeur_number" class="form-control" value="<?= $chauffuerNumber ?>"/></label>
</div>
</form>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
<button type="button" class="btn btn-primary" id="update">Check Out</button>
</div>
</div>
</div>
</div>
That is the modal, maybe I am missing something or perhaps not doing it correctly. I get a faded screen as if the modal was to popup but nothing comes up.
Oh on my getPhone.php i have just set variables that define the rows. Here it is.
<?php
require_once $_SERVER['DOCUMENT_ROOT'] . "/production/includes/db_connect.php";
$page = "admin";
$id = $_GET['id'];
$pdo = Database::connect();
if($_SESSION['LoggedIn'] == 1){
}
else if($_SESSION['LoggedIn'] == "")
{
header("location: http://wcl-wamp/");
}
$sql = "SELECT * FROM phone_inventory WHERE id = '$id'";
foreach ($pdo->query($sql) as $row) {
$phoneNumber = $row['phone_number'];
$deviceID = $row['device_id'];
$deviceMan = $row['device_manufacturer'];
$deviceModel = $row['device_model'];
$palias = $row['phone_alias'];
$chauffuerNumber = $row['chauffeur_number'];
}
Database::disconnect();
?>

Gregory Benner
5,732 PointsI'm not an expert at PHP but it looks like you are passing: 'param'+param as data, SQL is probably looking at just a Integer value for looking up. Try hardcoding 1 as the value you post with ajax. Also the modal <a href="getPhone.php?id='.$row['id'].'"> I believe the '.$row['id'].' doesn't do anything at this point, it would be something like either <?php .$row['id'].' ?> or "getPhone.php?row=1" I'm not 100% a pro so I would double check stackoverflow for things like "posting a form with ajax in php" and "PHP get variables" Hope that helps

Alan Johnson
7,625 Pointsvar data = 'param'+param;
This should be:
var data = {"param": param};
I'm pretty sure.
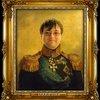
Barrie Freyre
23,301 PointsHello Allen Lamphear,
I am understanding that you want to show a message which is returned from your getPhone.php, isn't it ?
Watching your getPhone.php, I have the following doubt:
- In which format you want to return the value ? a simple text like "failure", "success", "error validation", "wrong id", "database doesn't connect", etc ? using XML ? or JSON ?
If is a simple message, I think you can uses a simple text, see below example;
<?php header("Content-Type:text/plain");
... manage code here with other messages status ...
echo 'Success'; exit(''); ?>
About the jquery, add the dataType, type, and cache to avoid any problems
$.ajax({
type:"GET",
cache:false,
url: "getPhone.php",
data: data,
dataType:"text",
success: function(msg){
alert( msg ) ; /* your code here */
},
error:function(){
alert("failure");
}
});
Don't forget to avoid sql injection attacks.
Regards, Barrie
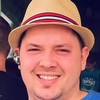
Allen Lamphear
Courses Plus Student 6,660 PointsI appreciate everything everyone is trying to do on helping me with this problem. :-) how about this. Can someone just post an example that works. This would be a table with data(generated by php) and a button to bring up a modal which retrieves data from a database. And I can work from there to see why my stuff isn't working.
TABLE
--------------------------------------------------------
[View = 1] | Bob | Doe | bob.doe@email.com
[View = 2] | John | Doe | john.doe@email.com
[View = 3] | April | Doe | april.doe@email.com
---------------------------------------------------------
Click View -> Modal -> Form with inputs [firstname] [lastname] [email]
If anyone can do that, i would be much appreciated. This can be the simplest php and ajax.
I am able to have a modal popup that has a form and when you submit the form, it places data into a database. I just cant seem to be able to retrieve data from the database.
Sorry for this. :(

John Dunnill
537 PointsDid you figure this out? I had the same problem a few years ago and found the best solution in this in my case as I was banging my head against the wall. I use it in all my apps and never had an issue.
http://dimsemenov.com/plugins/magnific-popup/
You need to use the AJAX one. He does all the AJAX code for you so you simply pass the variable into the modal via query string because you call the modal from a URL then Magnific automatically does an AJAX request as if the modal is loaded as a new page where you can then get the variable(s) from the query string.
You might want to encode the query string variable value itself to prevent people seeing what data you're passing in. They can only see it if they have the url link visible in their browser but encoding it means it will mean nothing to the rather than the insecure way of they seeing the ID passed in.
If you need any further help let me know and I can show you how to use it. Took a little while to get my head round it but I use it for all modals now.