Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial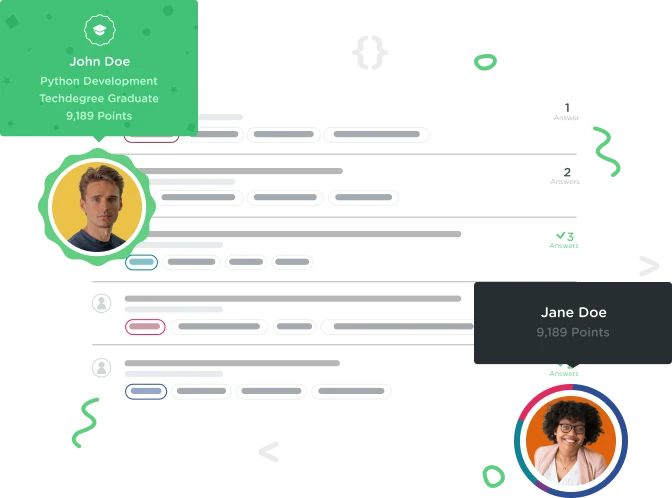

Martin Turjak
3,062 PointsQuery string removed from the url in HttpUrlConnection connection after any get method called
I noticed that using the code provided in the Android Blog Reader App tutorials (no matter what url I set in the URL string) the query string in HttpUrlConnection connection
gets lost after any "get" method gets called on it. Let me show you what I get while debugging (inside the doInBackground
method):
URL blogFeedUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=20");
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();
connection.connect();
If I set a breakpoint here and look into the connection
the url
is still the same as set above, i.e. the query string is count=20
. But if we resume the execution and get past this:
responseCode = connection.getResponseCode();
the query string gets set to null
so the url gets reset to "http://blog.teamtreehouse.com/api/get_recent_summary/"
(which in this case results in the default count of 10 posts). The question is ... am I doing something obvious wrong ... or is it some kind of bug (as I have read that HttpURLConnection
was, at least some time ago, considered quite buggy)?
Android Studio (Beta) 0.8.9
Build #AI-135.1404660, built Sep 3, 2014
JRE: 1.6.0_65-b14-462-11M4609 x86_64
JVM: Java HotSpot(TM) 64-Bit Server VM
1 Answer

Martin Turjak
3,062 PointsPlaying with it a bit more I found that using the DefaultHttpClient
and HttpGet
you can get around the problem. So I get the expected behavior if I substitute the `` method from the tutorial with this:
@Override
protected String doInBackground(Object... atr0) {
int responseCode = -1;
JSONObject jsonResponse = null;
StringBuilder builder = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpget = new HttpGet("http://blog.teamtreehouse.com/api/get_recent_summary/?count=20");
try {
HttpResponse response = client.execute(httpget);
StatusLine statusLine = response.getStatusLine();
responseCode = statusLine.getStatusCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while((line = reader.readLine()) != null){
builder.append(line);
}
jsonResponse = new JSONObject(builder.toString());
JSONArray jsonPosts = jsonResponse.getJSONArray("posts");
for(int i=0; i<jsonPosts.length(); i++) {
JSONObject jsonPost = jsonPosts.getJSONObject(i);
String title = jsonPost.getString("title");
Log.v(TAG, "Post " + i + ": " + title);
}
}
else {
Log.i(TAG, String.format("Unsuccessful HTTP response code: %d", responseCode));
}
}
catch (JSONException e) {
Log.e(TAG, "Exception Caught", e);
}
catch (Exception e) {
Log.e(TAG, "Exception Caught", e);
}
return "Code: " + responseCode;
}
This is adopted after this answer by Ben Jakuben (+ adjustment of imports required), to fit the examples in Stage 3 of the Blog Reader App Course.
This gives the expected output. However, if anyone knows what causes my problem in the original code - any insight would be much appreciated.