Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial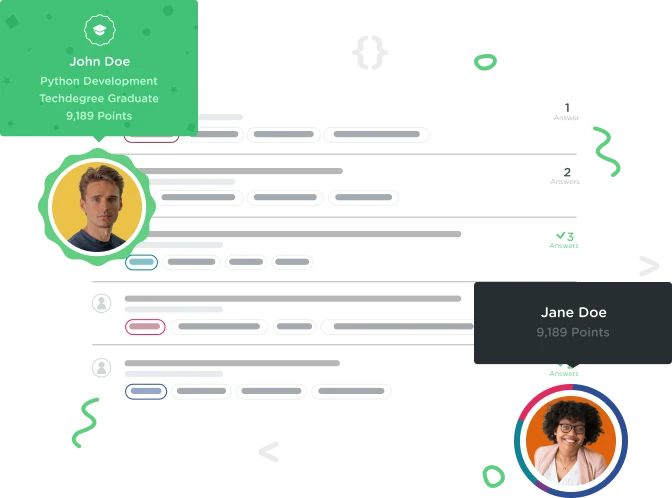
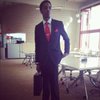
Deontre Jones
Courses Plus Student 2,625 PointsReally need some help , tried everything
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.
13 Answers

Scott Sheldon
6,942 PointsWithout seeing the entire exercise, I cannot be super specific, but basically what this is asking you to do is to create a function with one parameter: array. We can call the length method on an array by using .length
We'll also need to use an if / else statement to make sure the function returns 0 if a string, number, or undefined value is given.
So the basic code should look something like:
function arrayCounter(array) {
if (typeof array === "string") {
return 0;
} else if (typeof array === "number") {
return 0;
} else if (typeof array === "undefined") {
return 0;
} else {
return array.length;
}
}
Richard Glover
10,068 PointsI would like to add that I too was very confused as to what the question wanted. In previous challenges the challenge would have all to do with what you have just learned in that section. But with this challenge and most of the JavaScript is different to what Jim has been teaching. In a way it is good as it is a good challenge but the question doesn't mention that we will have to use script that was not in the last video. We could maybe have a little bit of a clue thrown in somewhere in the question. I have had to track this answer down and there is no way I would have got this answer.

Andrew Stover
5,172 PointsTry doing it w/out the elseif statements, since we haven't learned them before this lesson. If you pass an if statement and it fails, it automatically goes on to the next action in the function. So, my answer was something to the effect of (not correct syntax)
function (a) { if a is string return zero; if a is undefined return zero; if a is number return zero; return a.length; }

Andrew Stover
5,172 PointsTry doing it w/out the elseif statements, since we haven't learned them before this lesson. If you pass an if statement and it fails, it automatically goes on to the next action in the function. So, my answer was something to the effect of (not correct syntax)
function (a) { if a is string return zero; if a is undefined return zero; if a is number return zero; return a.length; }
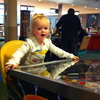
Paul Magnuson
5,965 PointsFeeling like Treehouse should incorporate a hint function .... But thanks to answer above I can at least move on.

Javier Rodriguez
6,317 PointsYeah my bad. I copied it wrong
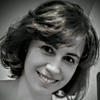
Miriam Tocino
14,201 PointsHey guys! Great help :) Thanks!
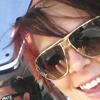
Heather Anderson
12,591 PointsThank you, guys!

Tony Nguyen
24,934 Pointsfunction arrayCounter (myArray) {
if (typeof myArray === 'string'){
return 0;
} else if (typeof myArray === 'number'){
return 0;
} else if (typeof myArray ==='undefined') {
return 0;
} else {
return myArray.length;
}
How come my code didn't work?
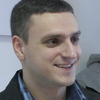
Dragisa Dragisic
8,391 Pointsfunction arrayCounter (myArray) { if (typeof myArray === 'string'){ return 0; } else if (typeof myArray === 'number'){ return 0; } else if (typeof myArray ==='undefined') { return 0; } else { return myArray.length;} }
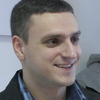
Dragisa Dragisic
8,391 Pointsfunction arrayCounter (myArray) {
if (typeof myArray === 'string'){
return 0;
} else if (typeof myArray === 'number'){
return 0;
} else if (typeof myArray ==='undefined') {
return 0;
} else {
return myArray.length;
}
}
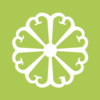
juan reyna
4,907 Pointsthis test is too difficult i just copied :(

James Barnett
39,199 PointsMy unsolicited advice about getting stuck on the code challenges ...
Treehouse is kinda like a book with videos instead of text and code challenges instead of exercises. So you can skip around just like you do in a book.
However, learning to program is kinda like learning algebra, each piece builds on the last one. If you move on without fully understanding it, it might come back to bite you. YMMV.

Airrick Dunfield
18,921 PointsI really agree with Juan on this one actually. I usually understand the challenges as soon as I get there (sometimes with a little research), but not this one. I ended up coming here and seeing how to answer it. Although I get why the if / else separation works, I feel the difference in the code Jim showed us and code that worked for me after many other tries was pretty big.
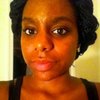
Alexandria Brown
10,648 PointsI will say James has a point. With HTML and CSS I flew through all of the videos b/c I had prior classroom knowledge before starting treehouse. The challenges were straight forward and literal. However Javascript has always been difficult for me and the whole breezing through the videos and wanting to jump behind the big guns when you're a novice is not a good idea. Specifically on this challenge you have to think just a tad, it's not handed to you like on the Web Design track. I actually watched the vids for this challenge in March 2014 multiple times but I got stuck and frustrated b/c it felt like Jim would talk about one thing in the videos then throw us a challenge on a whole 'nother subject. Looking back I was rushing, I was expecting it to be straightforward, and I was trying to get fancy too soon. But now that it's May, I came back and I rewatched the two videos and it makes sense, it's nothing from left field. I finally grasped the idea of how a function works and how a return factors into it. I still ended up needing help with the syntax but I'm glad I caught the big idea on my own. So yea if you're fresh to javascript be ready to go really, really, really slow it's not straight forward.

John Enderby
11,730 PointsJames does have a point, but to be honest I have read some JS books and completed the whole course on codecademy and this course is difficult to understand in parts. I don't doubt that Jim knows JS inside out and backwards, but the teaching style, and perhaps wording, is letting this particular section down.
Dave McFarland's course is much easier to progress through and understand.
I don't think that this code challenge is in itself overtly challenging, however the phrasing of the question does make it more difficult.

John Enderby
11,730 PointsJames does have a point, but to be honest I have read some JS books and completed the whole course on codecademy and this course is difficult to understand in parts. I don't doubt that Jim knows JS inside out and backwards, but the teaching style, and perhaps wording, is letting this particular section down.
Dave McFarland's course is much easier to progress through and understand.
I don't think that this code challenge is in itself overtly challenging, however the phrasing of the question does make it more difficult.

Dana Leventhal
Courses Plus Student 13,120 PointsTook a little bit but got it:
function arrayCounter(myArray) {
if(typeof myArray==='string'){
return 0;
}
if(typeof myArray==='number'){
return 0;
}
if(typeof myArray==='undefined'){
return 0;
}
else { return myArray.length;}
}
Hope this helps

John Enderby
11,730 PointsThis also works:
var arrayCounter = function(input) {
if (typeof input != "object"){
return 0;
} else {
return input.length;
}
};
As arrays are "special" kinds of objects this would pass the test. Just as an FYI and an alternative solution for those that might be looking for help ;)

Leisa Clark
4,531 PointsThat challenge nearly killed me!!! Thanks for all of your help
Javier Rodriguez
6,317 PointsJavier Rodriguez
6,317 PointsI tried that, doesn't work. =/ ...
Scott Sheldon
6,942 PointsScott Sheldon
6,942 PointsTry again :) I found the exercise and my above code allowed me to pass it without alterations. Make sure you check to see if you are missing parentheses or curly braces or similar somwhere.
Oliver Weber
4,868 PointsOliver Weber
4,868 PointsA simpler or more straightforward solution would be this:
Chad Ridings
1,002 PointsChad Ridings
1,002 PointsPerfect! Works like a charm with the "else if" statements, or without them.
I think the question was confusing to understand because it uses the words "string" and "number"... which leads you to believe that you should be checking against their type.
If it would have said check against something like "Jim", "Andrew", and "undefined" then it would have made perfect sense.