Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial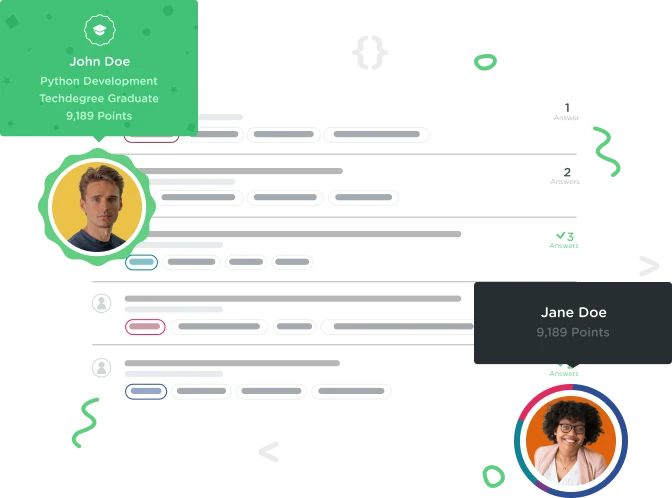
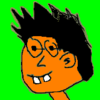
michaelangelo owildeberry
18,172 Pointsregister() view so that the form is validated on submission. If it's valid, use the models.User.new() method to create a
register() view so that the form is validated on submission. If it's valid, use the models.User.new() method to create a new User from the form data and flash the message "Thanks for registering!". You'll need to import flash().
task 1 is no longer passing
please help
what more can i do?
=)
from flask import Flask, render_template, flash, g
from flask.ext.login import LoginManager
import forms
import models
app = Flask(__name__)
app.secret_key = 'this is our super secret key. do not share it with anyone!'
login_manager = LoginManager()
login_manager.init_app(app)
@app.route('/register', methods=('GET', 'POST'))
def register():
form = forms.SignUpForm()
flash("Thanks for registering!")
if form.validate_on_submit():
models.User.new(
email=form.email.data,
password=form.password.data,
)
return render_template('register.html', form=form)
@login_manager.user_loader
def load_user(userid):
try:
return models.User.select().where(
models.User.id == int(userid)
).get()
except models.DoesNotExist:
return None
@app.before_request
def before_request():
g.db = models.DATABASE
g.db.connect()
@app.after_request
def after_request(response):
g.db.close()
return response
import datetime
from flask.ext.bcrypt import generate_password_hash
from flask.ext.login import UserMixin
from peewee import *
DATABASE = SqliteDatabase(':memory:')
class User(Model):
email = CharField(unique=True)
password = CharField(max_length=100)
join_date = DateTimeField(default=datetime.datetime.now)
bio = CharField(default='')
class Meta:
database = DATABASE
@classmethod
def new(cls, email, password):
cls.create(
email=email,
password=generate_password_hash(password)
)
def initialize():
DATABASE.connect()
DATABASE.create_tables([User], safe=True)
DATABASE.close()
from flask_wtf import Form
from wtforms import StringField, PasswordField
from wtforms.validators import DataRequired, Email, Length
class SignUpForm(Form):
email = StringField(validators=[DataRequired(), Email()])
password = PasswordField(validators=[DataRequired(), Length(min=8)])
join_date = DateTimeField(default=datetime.datetime.now)
bio = CharField(default='')
3 Answers
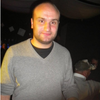
Vittorio Somaschini
33,371 PointsHello Michaelangelo,
I see a couple of things that would need to be adjusted here:
- The main one: the return statement does not look updated, it seems like you skipped task 2/3, please have another look at it
2.the flash message has to come AFTER the form validation on submit.
3.I think also, you only used the create.User.new() line without setting it equal to User in fact; that needs to be sorted as well.
I think that would be pretty much it, code should then pass the challenge. Let me know if it does not.
;) Vittorio
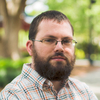
Kenneth Love
Treehouse Guest TeacherIsn't register()
supposed to render a template?
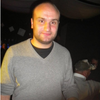
Vittorio Somaschini
33,371 PointsHello Michaelangelo.
I am sorry I made a mistake in my reply as well. I wrote this: create.User.new() but I meant to write this: models.User.new
I think the flash message can be inserted there even if in the teacher's video we placed it right after the if statement if I remember it correctly, so before the actual creation of the the User instance.
Last thing:
the return statement has to render a template; so something like:
return render_ ...
where after render_ you need to put the right code in order to render a template and the right one. You may want to have a look at the basic flask course videos to double check how this is done.
It should then work
Vittorio