Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial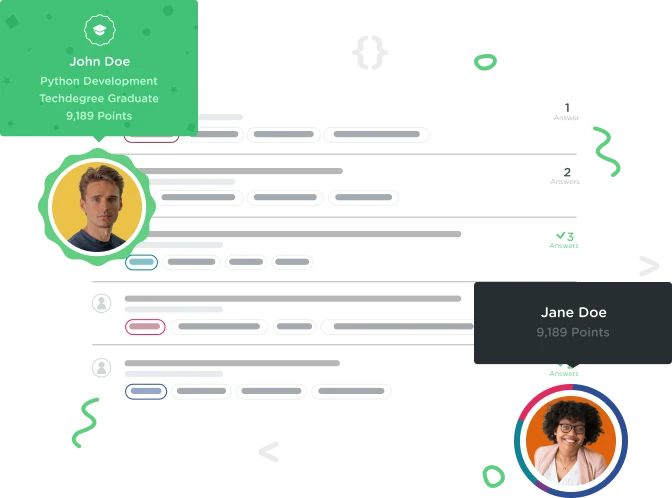

demetrios brinkmann
4,112 PointsRibbit app code challenge- sotryboard with tabBarController
hello out there,
i am having a really difficult time figuring out how to complete part 2 of this code challenge can anyone throw me a hint or two.
http://teamtreehouse.com/library/a-storyboard-with-a-tab-bar-controller
basically this is what it says:
Tabs have a property named 'badgeValue' that displays text in a red circle in the upper-right corner of the tab, like the number badges on the App Store or Messages apps. The tabs (UITabBarItems) are already stored in an array named 'tabBarItems'. Access the item in the array that corresponds to the selected index and then set the badge value to '3' for that UITabBarItem. Remember that 'badgeValue' must be an NSString!
not really sure how i go about solving this one, and i think some of my answers have been really far off.
i understand that i have to access the selected number in the array which i did in part 1
self.tabBarController.selectedIndex = 2;
i guess i am lost on how to access that object, and then to add the property to it and give the property a number.
thanks for any help!
4 Answers

Michael Reining
10,101 PointsI was also stuck here for a really long time. What I am just now starting to realize is that there are often many possible solutions to achieve the same thing. I have come up with four by now. Seeing all the workable solutions together has helped me understand this challenge a lot better so I wanted to share it in case it might help others too.
// 1st solution
UITabBarItem *item =
tabBarItems[self.tabBarController.selectedIndex];
item.badgeValue = @"3";
// 2nd solution
[[tabBarItems objectAtIndex:2]
setBadgeValue:[NSString stringWithFormat:@"3"]];
// 3rd solution
UITabBarItem *newItem = [tabBarItems objectAtIndex:2];
[newItem setBadgeValue:@"3"];
// 4th solution - cleanest, shortest, and simplest.
[[tabBarItems objectAtIndex:2] setBadgeValue:@"3"];

Stone Preston
42,016 Pointsit tells you the tabs are already stored in an array called tabBarItems. You need to access the item in the array that corresponds with the selected index
self.tabBarItems[self.tabBarController.selectedIndex]
The above code is the object that you need to modify the badgeValue of
so you could set a property of it like so
self.tabBarItems[self.tabBarController.selectedIndex].propertyName = whatever
see if that helps any. Also note that the badgeValue must set to a string.

demetrios brinkmann
4,112 Pointsthanks for the clues, so i modify the first code to set the badgeValue to a string @"3" so something like this?
((NSString *) [self.tabBarItems selectedIndex]).badgeValue = @"3";
or like this?
self.tabBarItems[self.tabBarItems.selectedIndex].badgeValue = @"3";
can you tell i'm still confused?
im not sure how to modify the badge value..?

Stone Preston
42,016 Pointsyour second code snippet should do the trick.
self.tabBarItems[self.tabBarItems.selectedIndex].badgeValue = @"3";
Have you done the objective C foundations course?

demetrios brinkmann
4,112 Pointsno haven't quite made it to objective c foundations yet.
i am still not getting the right answer on the code challenge is there something i am missing after that piece of code? thought it should be good..

Stone Preston
42,016 Pointssorry there was something wrong with your second code snippet. you had
self.tabBarItems[self.tabBarItems.selectedIndex].badgeValue = @"3";
the selected index is a property of the tab bar controller, not your array of tab bar items
self.tabBarItems[self.tabBarController.selectedIndex].badgeValue = @"3";
Does that work?

demetrios brinkmann
4,112 PointsWhat you say makes sense but im still not able to get the code challenge to say its correct... so no not working, anything else you can think of?

Stone Preston
42,016 Pointsweird. hmm try this
UITabBarItem *item = tabBarItems[self.tabBarController.selectedIndex];
item.badgeValue = @"3";

demetrios brinkmann
4,112 Pointsyep that did it!! thanks for all the patience and help!