Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial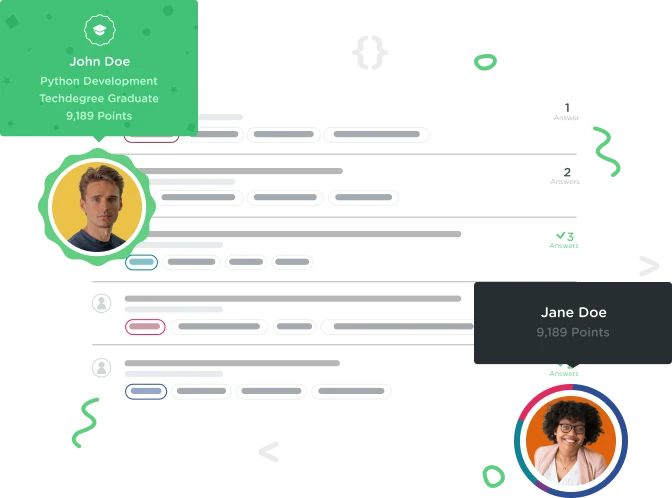

Martin Stryffeler
1,744 PointsRibbit crashes on startup
I've just finished the first Stage, Starting the App, and Ribbit crashes whenever I try to run it in the emulator. I get these errors in the log:
04-15 19:35:58.131: E/AndroidRuntime(1366): FATAL EXCEPTION: main
04-15 19:35:58.131: E/AndroidRuntime(1366): Process: com.stryffeler.ribbit, PID: 1366
04-15 19:35:58.131: E/AndroidRuntime(1366): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.stryffeler.ribbit/com.stryffeler.ribbit.LoginActivity}: java.lang.NullPointerException
04-15 19:35:58.131: E/AndroidRuntime(1366): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2195)
04-15 19:35:58.131: E/AndroidRuntime(1366): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2245)
04-15 19:35:58.131: E/AndroidRuntime(1366): at android.app.ActivityThread.access$800(ActivityThread.java:135)
04-15 19:35:58.131: E/AndroidRuntime(1366): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1196)
04-15 19:35:58.131: E/AndroidRuntime(1366): at android.os.Handler.dispatchMessage(Handler.java:102)
04-15 19:35:58.131: E/AndroidRuntime(1366): at android.os.Looper.loop(Looper.java:136)
04-15 19:35:58.131: E/AndroidRuntime(1366): at android.app.ActivityThread.main(ActivityThread.java:5017)
04-15 19:35:58.131: E/AndroidRuntime(1366): at java.lang.reflect.Method.invokeNative(Native Method)
04-15 19:35:58.131: E/AndroidRuntime(1366): at java.lang.reflect.Method.invoke(Method.java:515)
04-15 19:35:58.131: E/AndroidRuntime(1366): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:779)
04-15 19:35:58.131: E/AndroidRuntime(1366): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:595)
04-15 19:35:58.131: E/AndroidRuntime(1366): at dalvik.system.NativeStart.main(Native Method)
04-15 19:35:58.131: E/AndroidRuntime(1366): Caused by: java.lang.NullPointerException
04-15 19:35:58.131: E/AndroidRuntime(1366): at com.stryffeler.ribbit.LoginActivity.onCreate(LoginActivity.java:24)
04-15 19:35:58.131: E/AndroidRuntime(1366): at android.app.Activity.performCreate(Activity.java:5231)
04-15 19:35:58.131: E/AndroidRuntime(1366): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1087)
04-15 19:35:58.131: E/AndroidRuntime(1366): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2159)
04-15 19:35:58.131: E/AndroidRuntime(1366): ... 11 more
Hopefully this is enough for somebody to figure out what I've overlooked. Let me know what other information I can provide to help figure this out.
Thanks.
Edit:
Here is the code. I tried to find the uninitialized variable, but I couldn't find anything wrong with it myself.
package com.stryffeler.ribbit;
import android.app.Activity; import android.app.Fragment; import android.content.Intent; import android.os.Bundle; import android.view.LayoutInflater; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.view.ViewGroup; import android.widget.TextView;
public class LoginActivity extends Activity {
protected TextView mSignUpTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
mSignUpTextView = (TextView)findViewById(R.id.signUpText);
mSignUpTextView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(LoginActivity.this, SignUpActivity.class);
startActivity(intent);
}
});
if (savedInstanceState == null) {
getFragmentManager().beginTransaction()
.add(R.id.container, new PlaceholderFragment()).commit();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.login, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
/**
* A placeholder fragment containing a simple view.
*/
public static class PlaceholderFragment extends Fragment {
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_login,
container, false);
return rootView;
}
}
}
4 Answers
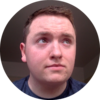
Liam Peters
8,792 PointsLooking at your code I suspect that if you look in your R.layout.activity_login file then there won't be an id called signUpText (maybe capitalisation?).
I think from my attempt at counting, the line in question is where you are assigning the on click listener. This is failing because the previous line is not finding the button by id and thus it's null when you come to add the onClickListener.
This once happened to me when I had multiple layouts for different screen sizes (you can define a layout for landscape and another for portrait for instance) and I had forgotten to add an ID for the portrait layout.
Have a check of your IDs!
If this doesn't solve it, are you in eclipse or android studio? If eclipse, I find that cleaning my projects helps (Project -> Clean... -> Ok)!

Martin Stryffeler
1,744 PointsThis was the solution. I had accidentally placed those ids in fragment_login.xml instead of activity_login. Thank you!

Ernest Grzybowski
Treehouse Project ReviewerAs Liam Peters stated, your problem is a null pointer exception on line 24 of LoginActivity.java
This usually means that you have declared the variable, but have not yet initialized it to anything. If you need any more help then edit your question and add your code from your LoginActivity.java and it should be fairly trivial to solve your problem.

Martin Stryffeler
1,744 PointsI've added the code as you suggested, if you don't mind taking another look. I haven't been able to find the uninitialized variable myself.
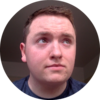
Liam Peters
8,792 PointsYou have a null pointer exception on line 24 of your Login Activity. This generally means that you haven't given something a value or Instantiated it's value. Have a look over that line and make sure everything is given a value before that line is reached in execution/event order

william parrish
13,774 PointsAre you using the project files included with the lessons, or did you setup everything line by line yourself ( including the AndroidManifest.xml file)?
Martin Stryffeler
1,744 PointsMartin Stryffeler
1,744 PointsAdded the LoginActivity.java code.