Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial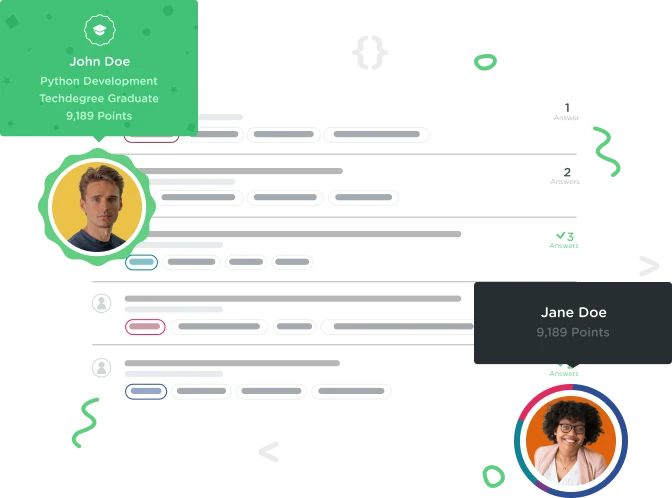

Karan Keelor
436 PointsRunning program in Eclipse..getting nullpointerException error..please help
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
String ageAsString = console.readLine("How old are you? \n" );
int age = Integer.parseInt(ageAsString);
if (age < 13) {
//Insert exit code for if statement
console.printf("Sorry you must be atleast 13 to use this program\n");
}
System.exit(0);
String name = console.readLine("Enter a name: ");
String adjective = console.readLine("Enter an adjective: ");
String noun = console.readLine("Enter a noun: ");
String adverb = console.readLine("Enter an adverb: ");
String verb = console.readLine("Enter a verb: ");
console.printf("Your Tree Story: \n------------\n");
console.printf("%s is a %s %s", name, adjective, noun);
console.printf("They are always %s %s.\n" ,adverb, verb );
}
}
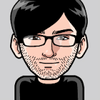
Alexandru Doru Grosu
2,753 PointsAh okay, that makes sense.
This a bug with Eclipse console, not your program. System.console()
returns null
sometimes in Eclipse.
https://bugs.eclipse.org/bugs/show_bug.cgi?id=122429
So when you try to do this
String ageAsString = console.readLine("How old are you? \n" );
The console object is null
, which is way you get a NullPointerException
.
If you want to read input through the console provided by Eclipse I suggest you use java.util.Scanner
.
You can find the documentation for it here: http://docs.oracle.com/javase/7/docs/api/java/util/Scanner.html
1 Answer
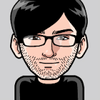
Alexandru Doru Grosu
2,753 PointsApologies for the delayed response.
Where exactly are you running this example? In the workspace or in one of the challenges? I am asking because I think the culprit might be again
Console console = System.console();
As I told you in an earlier post, whenever you write code that uses the console
object, you should just assume it was already assigned for you and just use it.
Also, in the javadoc for the Console class it is specified that "Unless otherwise specified, passing a null argument to any method in this class will cause a NullPointerException to be thrown."
So, if in any of the lines that assign input via readLine
, assign a null
value to the string
, you will get a NullPointerException
when you try to printf
.
In the same javadoc it is also mentioned that "The console-read methods return null when the end of the console input stream is reached, for example by typing control-D on Unix or control-Z on Windows. Subsequent read operations will succeed if additional characters are later entered on the console's input device.".
So your code might not be wrong, you just may be passing wrong arguments without realizing it. Make sure you type in at least one character for all the values you prompt for when you run the code.
Karan Keelor
436 PointsKaran Keelor
436 PointsIm trying to run it in Eclipse..but getting this error each time:
Exception in thread "main" java.lang.NullPointerException at TreeStory.main(TreeStory.java:8)