Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial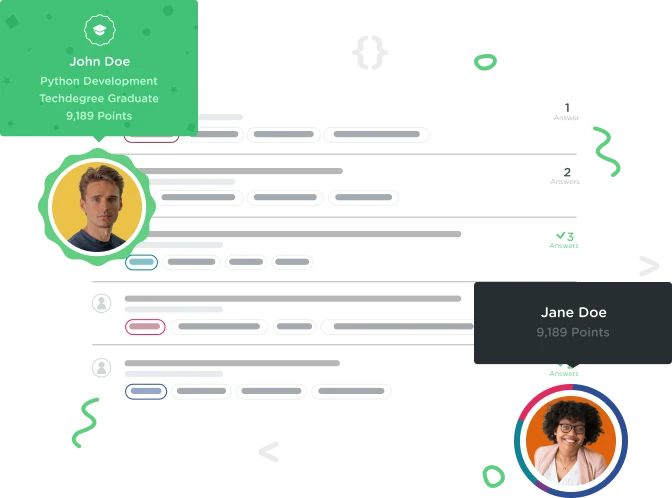

hello world
346 PointsSelf destructing message app - Inbox ios7 error
Here is a screenshot of my code: http://imgur.com/4Kabekg. Do anyone now how to change that so it works with ios7?
9 Answers

Ben Jakuben
Treehouse TeacherIt's unfortunately a bit tricky to get working. I have yet to find a way that makes it work exactly like it did in iOS 6, but it doesn't need to work exactly the same. If you want to get a thumbnail for use somewhere, follow these steps:
Step 1<br/>
Paste the method from the answer Amit linked to in your view controller implementation file as a new method. Use this code as I added a line for appliesPreferredTrackTransform
to maintain portrait videos:
- (UIImage *)thumbnailFromVideoAtURL:(NSURL *)url
{
AVAsset *asset = [AVAsset assetWithURL:url];
// Get thumbnail at the very start of the video
CMTime thumbnailTime = [asset duration];
thumbnailTime.value = 0;
// Get image from the video at the given time
AVAssetImageGenerator *imageGenerator = [[AVAssetImageGenerator alloc] initWithAsset:asset];
imageGenerator.appliesPreferredTrackTransform = YES;
CGImageRef imageRef = [imageGenerator copyCGImageAtTime:thumbnailTime actualTime:NULL error:NULL];
UIImage *thumbnail = [UIImage imageWithCGImage:imageRef];
CGImageRelease(imageRef);
return thumbnail;
}
Step 2<br/> Add AVFoundation framework to your project. Click on the Project > Build Phases > Link Binary with Libraries and then click on the plus sign to add AVFoundation.framework.
Step 3<br/> Import the framework header in your view controller:
#import <AVFoundation/AVFoundation.h>
Step 4<br/>
Get a UIImage view using the new method. Put this where you currently have the line for thumbnailImageAtTime
:
UIImage *thumbnail = [self thumbnailFromVideoAtURL:self.moviePlayer.contentURL];
Step 5<br/> Use the thumbnail wherever you need it. I haven't yet gotten it to load instead of the black screen yet, but you can kind of do it by adding this code:
UIImageView *imageView = [[UIImageView alloc] initWithImage:thumbnail];
[self.moviePlayer.backgroundView addSubview:imageView];
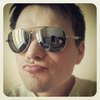
Alexey Kazantsev
4,310 PointsWhat about:
[self.moviePlayer requestThumbnailImagesAtTimes:[NSArray arrayWithObjects:0, nil] timeOption:MPMovieTimeOptionNearestKeyFrame];

Jason Edstrom
8,976 PointsThat method does work. The only change that your code needs is that the playback times needs to be a NSNumber.
Like so:
[self.moviePlayer requestThumbnailImagesAtTimes:@[@0] timeOption:MPMovieTimeOptionNearestKeyFrame];

hello world
346 PointsThank you I saw that. But i don't understand. :(
Should i just replace: [self.moviePlayer thumbnailImageAtTime:0 timeOption:MPMovieTimeOptionNearestKeyFrame];
With:
- (UIImage *)thumbnailFromVideoAtURL:(NSURL *)url
{
AVAsset *asset = [AVAsset assetWithURL:url];
// Get thumbnail at the very start of the video
CMTime thumbnailTime = [asset duration];
thumbnailTime.value = 0;
// Get image from the video at the given time
AVAssetImageGenerator *imageGenerator = [[AVAssetImageGenerator alloc] initWithAsset:asset];
CGImageRef imageRef = [imageGenerator copyCGImageAtTime:thumbnailTime actualTime:NULL error:NULL];
UIImage *thumbnail = [UIImage imageWithCGImage:imageRef];
CGImageRelease(imageRef);
return thumbnail;
}
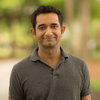
Amit Bijlani
Treehouse Guest TeacherYou can add the above method to your view controller and wherever you need a thumbnail image call the above method by passing the url of the location of your movie.

hello world
346 PointsThanks. I'm doing the Self destructing message app so I want it like that. I get an error when i delete the old code with this new one. :( How would it look like in code with the Self destructing message app?

hello world
346 PointsThank you so much Ben! It works perfectly!

Scott Taylor
7,089 PointsSo, I'm a bit lost - do we have a conclusive answer for including video in the app for iOS7?

Ben Jakuben
Treehouse TeacherThe video works fine, and the solution above works for getting a thumbnail. The specific behavior that's a little different between iOS 6 and 7 right now is that when the video first loads in iOS 7, it spreads to full screen in all black before starting playing. In iOS 6 it spreads with the thumbnail. I got a partial solution working for this, but it wasn't perfect and also wasn't that important, in my opinion. If you're having other trouble, though, post a new thread and we'll help you get situated. :)

Scott Taylor
7,089 PointsGreat thanks Ben Jakuben, I'm going to continue - nearly completed :)

James Lin
4,601 PointsJust replace the line with [self.moviePlayer requestThumbnailImagesAtTimes:@[@0] timeOption:MPMovieTimeOptionNearestKeyFrame];