Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial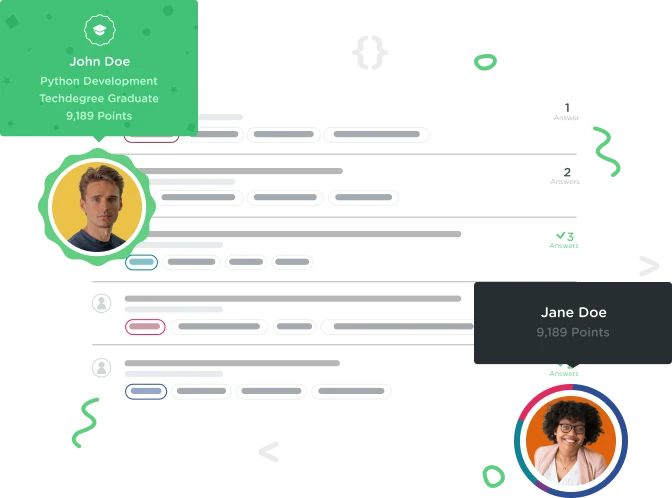

orange sky
Front End Web Development Techdegree Student 4,945 Pointssorting numbers Ascending, Descending, and equal????
Hello!
Thank you for all your help guys. I just have to finish "Arrays" lesson, and I will get to my mails:)
Anyway, the instructor says when sorting numbers in an array, we use an anonymous function. This function can sort array 3 ways:
1) if a (5) is smaller than b(10), then it sorts from small to big 2) if a(10) is bigger than b(5), this returned positive value , causes the array to sort from big to small(descending order) 3) if a and b are equal, it returns??? I can't imagine this one. Is it an array like this [32,32,32,32]. Why kind of assignment would need to sort of sorting?
I manage to write code to check a sort done in either ascending and descending manner. I am not sure of the third one or how to execute it.
Thank you all!!
This code shows the decending or ascending versions of a number array:
<!DOCTYPE html>
<head>
</head>
<body>
<script>
var myA = [10,44,32, 100, 2];
//myA.sort(function(a, b) { return b-a; }); descending order
myA.sort(function(a,b){
return a - b;
});// ascending order
console.log( myA);
</script>
</body>
</html>
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi orange sky,
The sort method will pass in two elements from the array into the compare function and the compare function will return a value letting the sort method know where it should place those two elements.
If the compare function returns a negative value then a
should come before b
in the final sorted array.
If a positive value is returned then b
should come before a
.
And if zero is returned then a
and b
should be treated equal and their original order may or may not be preserved.
So looking at your return value return b-a
if 10 and 44 were passed in for a
and b
respectively we would get a positive return value. A positive return value indicates that b
should come before a
. Meaning that 44 should come before 10 in the final sorted array. This means that you have set up your compare function to sort the array in descending order. Largest to smallest.
If you change your return statement to return a-b
then it will sort in ascending order.

orange sky
Front End Web Development Techdegree Student 4,945 PointsHello Jason,
I have posted the code properly so that you can see that I am able to write a code to put an array in descending and ascending order; however I would like to write a similar code for this scenario:
"And if zero is returned then a and b should be treated equal and their original order may or may not be preserved." <-- you wrote this.
Can you please help me write a small code to test when 'a and' be are equal. In fact, would you happen to know when to write an array where a and b are equal. I can't seem to uderstand why and array would have all equal values

Dino Paškvan
Courses Plus Student 44,108 PointsAn example:
var arr = [0, 1, 5, 1, 7, 5, 0, 3];
A scenario where all the elements in the array are equal is less likely, but it's also not impossible.
However, a scenario where some of the elements of the array are equal is quite common.
Imagine you're writing a computer game, and your player character has an inventory. To keep track of all his possessions, you could use an array.
That array could look something like this:
var mainInventory = ["axe", "potion of health", "food ration", "food ration", "potion of health", "common shirt", "potion of health"];
In the scenario of this imaginary game, as your character picks items up, they're added into the mainInventory
array. The character could easily have picked up more than one food ration or potion of health, so the array reflects that.
Maybe, when graphically showing the inventory to the player, you'd want to sort the inventory so that the player can find items more easily. And this is where you might potentially be sorting an array where some elements are equal.

Jason Anello
Courses Plus Student 94,610 PointsThanks for the assistance Dino. As Dino mentions it's unlikely you will have an array with all equal items. Even if you did then it's technically already sorted. I realize though that you may not know they're all equal and still have to run through the sort process anyways.
When you do have some equal items and the sort method passes two of those equal items into the compare function then it should return 0 to let the sort method know that those two items are equal.
I think where the confusion lies is that you think there are 3 ways to sort but there are only two ways to sort. You can sort in either descending or ascending order. You have covered both in your original code. There isn't some 3rd option that I'm aware of.
You wrote 1) if a (5) is smaller than b(10), then it sorts from small to big 2) if a(10) is bigger than b(5), this returned positive value , causes the array to sort from big to small(descending order) 3) if a and b are equal, it returns??? I can't imagine this one. Is it an array like this [32,32,32,32]. Why kind of assignment would need to sort of sorting?
You seem to be suggesting that if a
is smaller than b
then that determines an ascending sort order. It's the compare function that determines the sort order not the relative values of a
and b
When you use return a - b;
that sets the sort order to be ascending. The sort order doesn't have anything to do with the relative values of a
and b
that are passed in.
To say it another way using your numbers, if 5 and 10 are passed in for a
and b
, that does not mean the array will be sorted in ascending order. Which of the 3 values (positive, negative, or zero) that you return from the compare function will determine the order.
The sort method is going to call the compare function multiple times during the sorting process. It's going to pass 2 elements from the array into the compare function. For any given array, sometimes a
will be less than b
, or b
will be less than a
, or a
and b
will be equal.
All 3 of these possibilities can happen on the same array whether it's being sorted in ascending or descending order. In my answer I explained each of these 3 possibilities and what value the compare function should return in each case.
Does that clear it up for you or have I made it more confusing?

orange sky
Front End Web Development Techdegree Student 4,945 PointsThank you Dino and Jason! I now understand what the lecturer meant by 'equal values ' in an array.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsThis link shows how to post code in the forums: https://teamtreehouse.com/forum/posting-code-to-the-forum