Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial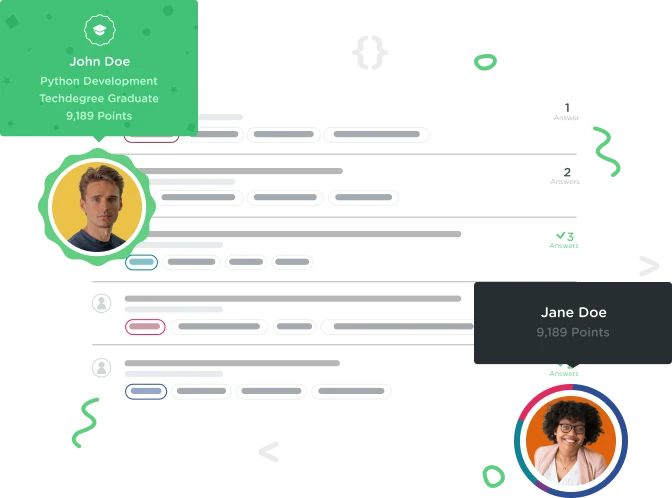
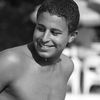
Hossam Khalifa
17,200 PointsStuck here
Can not start anything with this challenge
angular.module('myApp', [])
.controller('myController', function($scope) {
$scope.user = {
name: 'Alex'
};
})
.directive('myDirective', function () {
return {
require: 'ngModel',
priority: 1,
link: function ($scope, $element, $attrs, ngModelCtrl) {
// YOUR CODE HERE
}
}
});
<!DOCTYPE html>
<html ng-app="myApp">
<head>
<title>Angular.js</title>
<script src="js/angular.js"></script>
<script src="app.js"></script>
</head>
<body ng-controller="myController">
<p>Hello {{user.name}}</p>
<input type="text" ng-model="user.name" />
<div my-directive ng-model="user.name"></div>
</body>
</html>
1 Answer
jaredcowan
11,808 PointsI think you need to inject $scope then the function. I made a plunker to show you it working. You can move the code inside the script tag to your js file. http://plnkr.co/edit/suOZoGvKN8XTukJBJO32?p=preview
angular.module('myApp', [])
.controller('myController', ['$scope', function($scope) {
$scope.user = {
name: 'Alex'
}
}]);
.directive('myDirective', function () {
return {
require: 'ngModel',
priority: 1,
link: function ($scope, $element, $attrs, ngModelCtrl) {
// YOUR CODE HERE
}
}
});
So your html can be this
<!doctype html>
<html lang="en" ng-app="myApp">
<head>
<title>Angular.js</title>
<script src="js/angular.js"></script>
<script src="app.js"></script>
</head>
<body ng-controller="myController">
<div>
Enter name: <input type="text" ng-model="user.name"><br>
Hello <span ng-bind="user.name"></span>!
</div>
</body>
</html>
Hossam Khalifa
17,200 PointsHossam Khalifa
17,200 Pointsnot working
jaredcowan
11,808 Pointsjaredcowan
11,808 PointsAre you loading the libraries correctly? You have angular in a js folder and not your app file?
Prefixed with js/ <script src="js/angular.js"></script>
not prefixed with js/ <script src="app.js"></script>