Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial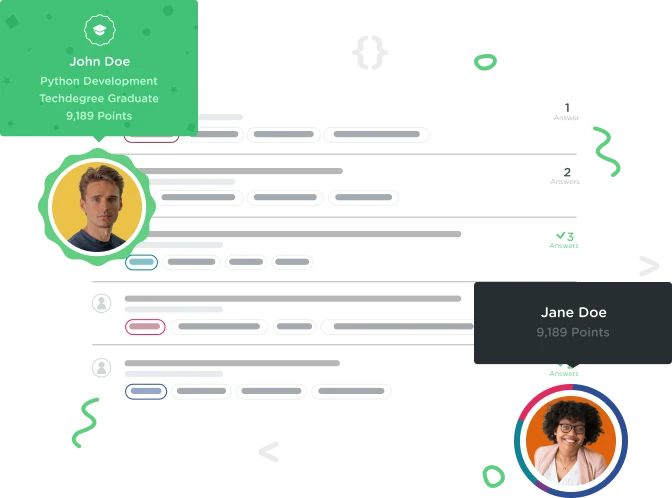

Wali Ali
2,040 PointsTreebook app: First_name, Last_name, profile_name nat saving
when I visit the /registration page and try to create a new user, I get the following error message: 3 errors prohibited this user from being saved: First name can't be blank Last name can't be blank Profile name can't be blank
Here is my devise generated view: views/devise/registrations/new.html.erb
<h2>Sign up</h2>
<%= form_for(resource, :as => resource_name, :url => registration_path(resource_name)) do |f| %>
<%= devise_error_messages! %>
<div><%= f.label :first_name %><br />
<%= f.text_field :first_name %></div>
<div><%= f.label :last_name %><br />
<%= f.text_field :last_name %></div>
<div><%= f.label :profile_name %><br />
<%= f.text_field :profile_name %></div>
<div><%= f.label :email %><br />
<%= f.email_field :email, :autofocus => true %></div>
<div><%= f.label :password %><br />
<%= f.password_field :password %></div>
<div><%= f.label :password_confirmation %><br />
<%= f.password_field :password_confirmation %></div>
<div><%= f.submit "Sign up" %></div>
<% end %>
<%= render "devise/shared/links" %>
HERE IS MY USER'SMODEL:
class User < ActiveRecord::Base
# Include default devise modules. Others available are:
# :confirmable, :lockable, :timeoutable and :omniauthable
devise :database_authenticatable, :registerable,
:recoverable, :rememberable, :trackable, :validatable
has_many :statuses, dependent: :destroy
validates :first_name, :last_name, presence: true
validates :profile_name, presence: true,
uniqueness: true
def full_name
first_name + " " + last_name
end
end
USER'S MIGRATION TABLES
class DeviseCreateUsers < ActiveRecord::Migration
def change
create_table(:users) do |t|
t.string :first_name
t.string :last_name
t.string :profile_name
## Database authenticatable
t.string :email, :null => false, :default => ""
t.string :encrypted_password, :null => false, :default => ""
## Recoverable
t.string :reset_password_token
t.datetime :reset_password_sent_at
## Rememberable
t.datetime :remember_created_at
## Trackable
t.integer :sign_in_count, :default => 0, :null => false
t.datetime :current_sign_in_at
t.datetime :last_sign_in_at
t.string :current_sign_in_ip
t.string :last_sign_in_ip
## Confirmable
# t.string :confirmation_token
# t.datetime :confirmed_at
# t.datetime :confirmation_sent_at
# t.string :unconfirmed_email # Only if using reconfirmable
## Lockable
# t.integer :failed_attempts, :default => 0, :null => false # Only if lock strategy is :failed_attempts
# t.string :unlock_token # Only if unlock strategy is :email or :both
# t.datetime :locked_at
t.timestamps
end
add_index :users, :email, :unique => true
add_index :users, :reset_password_token, :unique => true
# add_index :users, :confirmation_token, :unique => true
# add_index :users, :unlock_token, :unique => true
end
end
class AddUserIdToStatuses < ActiveRecord::Migration
def change
add_column :statuses, :user_id, :integer
add_index :statuses, :user_id
remove_column :statuses, :name
end
end
When I try to create a new user in the console, I do succeed. as the following irb output shows: => #<User id: 7, first_name: "Wali", last_name: "ali", profile_name: "WaliAli", email: "abdishwak@gmail.com", encrypted_password: "$2a$10$KJhklRFhI9TYk8ASg3hte.MpNwfp9yh18yAoY5mbx5fi...", reset_password_token: nil, reset_password_sent_at: nil, remember_created_at: nil, sign_in_count: 0, current_sign_in_at: nil, last_sign_in_at: nil, current_sign_in_ip: nil, last_sign_in_ip: nil, created_at: "2014-02-25 22:53:08", updated_at: "2014-02-25 22:53:08">
user.valid? User Exists (0.3ms) SELECT 1 AS one FROM "users" WHERE ("users"."profile_name" = 'WaliAli' AND "users"."id" != 7) LIMIT 1 => true User.count (0.3ms) SELECT COUNT(*) FROM "users" => 1
7 Answers
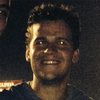
Rodrigo Soares
2,460 PointsI find out a solution for this problem. We have to modify some code on the app controller in order to allow those new field to be assign.
Go to controllers/application_controller.rb
before_filter :configure_permitted_parameters, if: :devise_controller?
protected
def configure_permitted_parameters
devise_parameter_sanitizer.for(:sign_up) { |u| u.permit(:first_name, :last_name, :profile_name, :email, :password, :password_confirmation, :remember_me) }
end
end
Type that in.
Notice that in my case I have created 3 new fields just like you first_name , last_name and profile_name , but if you might have any additional field you'll have to place it after the parentheses.
Hope it will work for you too.
For more details on that just read the Devise wikis : https://github.com/plataformatec/devise/wiki/How-To:-Allow-users-to-sign-in-using-their-username-or-email-address
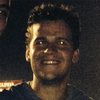
Rodrigo Soares
2,460 PointsAdd that line of code into your User's model ''' attr_accessible :email, :password, :password_confirmation, :remember_me, :first_name, :last_name, :profile_name '''
See if it works, I had the same problem, I gave access to the mass assignment and it worked out for me.

Wali Ali
2,040 PointsI can't do that. I'm using Rails 4 which prohibits attributes to be placed in models. If I do that, I'll get the following error message:
attr_accessible':
attr_accessibleis extracted out of Rails into a gem. Please use new recommended protection model for params(strong_parameters) or add
protected_attributes` to your Gemfile to use old one. (RuntimeError)
They moved it to controllers where you can whitelist the specific attributes but then I don't have controller for user. at least yet.
Are you using Rails 4?
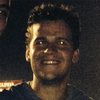
Rodrigo Soares
2,460 PointsGo to your Gem file and add this : gem 'protected-attributes' Go to your terminal and type: bundle
After that you can add the code I mentioned above it should work just fine.

Wali Ali
2,040 Pointstried that. installed the gem and ran bundle. but I still can't register a user. here is the error form the Sign Up page:
Sign up 3 errors prohibited this user from being saved: First name can't be blank Last name can't be blank Profile name can't be blank First name
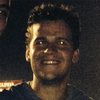
Rodrigo Soares
2,460 PointsThis is the exactly the same place I'm stuck... I'm searching on internet if I find any solution I'll let you know.

Wali Ali
2,040 PointsWonderful. that worked. Thanks man!
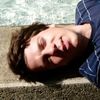
Matej Lukášik
27,440 PointsWhat worked?
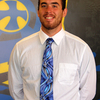
Robert Cekay
12,460 Points???
Zach Krasner
846 PointsZach Krasner
846 PointsTHANK YOU!