Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial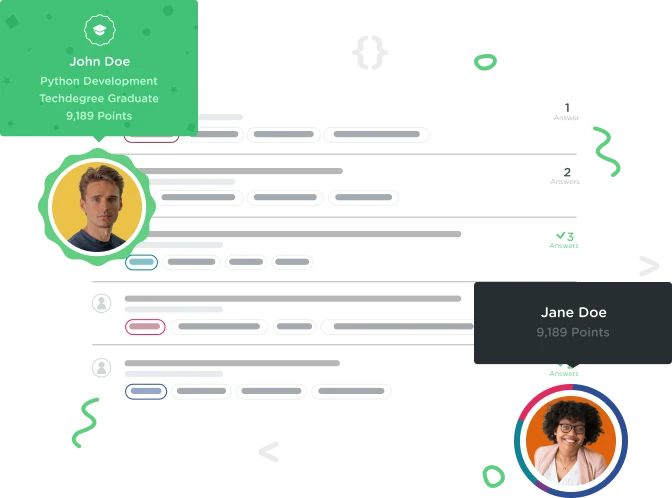

William J. Terrell
17,403 PointsUnderstanding the Concept
So I passed this challenge, but I'm still having some difficulty grasping the concept here (maybe I'm making it out to be more complicated than it really is?)
I think I have a general understanding of what is going on, but if someone could explain in greater detail, I would really appreciate :)
Also, just out of curiosity, for the "if (equals(blog))", could we just as well use "if (BlogPost == blog)", or is there a different concept?
Thanks!
package com.example;
import java.util.Date;
public class BlogPost implements Comparable{
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
@Override
public int compareTo(Object obj){
BlogPost blog = (BlogPost) obj;
if (equals(blog)){
return 0;
}
int DateCreated = mCreationDate.compareTo(blog.mCreationDate);
if (DateCreated == 0) {
return mTitle.compareTo(blog.mTitle);
}
return DateCreated;
}
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
1 Answer
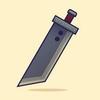
Allan Clark
10,810 Points@Override
public int compareTo(Object obj){
BlogPost blog = (BlogPost) obj;
if (equals(blog)){
return 0;
}
int DateCreated = mCreationDate.compareTo(blog.mCreationDate);
if (DateCreated == 0) {
return mTitle.compareTo(blog.mTitle);
}
return DateCreated;
}
@Override is the keyword used to denote methods that are required by the Interface. compareTo() method accepts and Object obj to compare to the BlogPost it was called on. We use Object because everything in Java is an Object and ideally we want to be able to compare it to any other Objects.
BlogPost blog = (BlogPost) obj;
if (equals(blog)){
return 0;
}
Here we cast the parameter from a generic Object into the more specific BlogPost variable. This gives us access to the BlogPost methods. Then we check if that BlogPost (named blog) is equal to this BlogPost. It could be written explicitly: "if(this.equals(blog)){". If the BlogPosts are the same we return 0.
As for your side question, it could not be written like this "if (BlogPost == blog)" for 2 reasons. First BlogPost is the Class name and doesn't refer to any specific Object so the compiler won't know what you are trying to compare. The keyword you would use here is "this" to have a method refer to "this" object it was called on. Second "if(this == blog)" also wouldn't work because the binary operator == is less "smart" than an equals method. == works great on Primitive values like ints, but when using it with 2 Objects it will only return true if the actual memory reference for each Object is exactly the same. Basically you have to be comparing literally the exact same Object to itself (which can be more useful than it sounds).
int DateCreated = mCreationDate.compareTo(blog.mCreationDate);
if (DateCreated == 0) {
return mTitle.compareTo(blog.mTitle);
}
return DateCreated;
}
*side note here by convention the variable here should be camelCased i.e. dateCreated
Here we take the member variable "mCreationDate" of each BlogPost ("this" and "blog"), we then compare each of those storing the value in the dateCreated variable. Basically this will tell you blog's mCreationDate is before (-1) or after (1) this.mCreationDate. If the mCreationDate(s) are the same (0), meaning both BlogPosts happened on the same Date at the same Time, then we return a comparison of the mTitle(s) since we need another metric to compare.
Hope this helps. Please let me know if I can clear any of it up.