Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial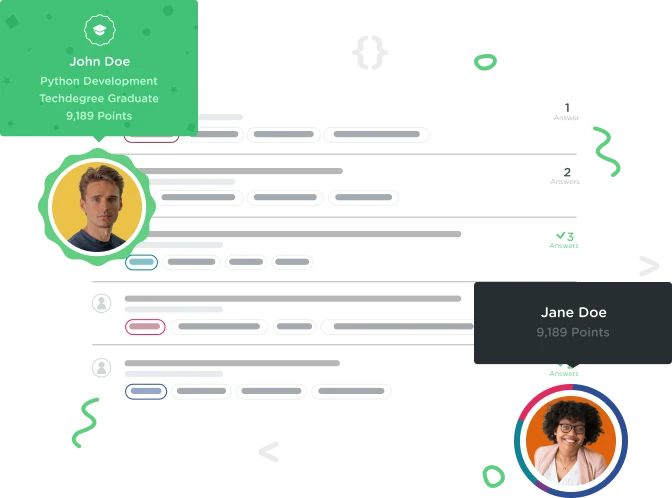

Riley MacDonald
2,542 PointsUsing SetText for Tabs
I have having trouble with the following code challenge from the "Implementing Designs in Android" course.
Here is the challenge:
This music app has three tabs. In MainActivity below, set the text for each tab using the method setText(). As the parameter, use the value returned by the PagerAdapter's getPageTitle() method.#
Here is my code:
import android.support.v4.app.FragmentActivity;
public class MainActivity extends FragmentActivity implements
ActionBar.TabListener {
// Some code has been omitted for brevity!
public ActionBar mActionBar;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mActionBar = getActionBar();
mActionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
PagerAdapter pagerAdapter = new PagerAdapter(getSupportFragmentManager());
// Some code omitted for brevity...
for (int i = 0; i < pagerAdapter.getCount(); i++) {
ActionBar.Tab tab = mActionBar.newTab();
tab.setTabListener(this);
tab.setText(PagerAdapter.getPageTitle());
/*
* Add your code here to set the text!
*/
mActionBar.addTab(tab);
}
}
// These listener methods are intentionally blank
@Override
public void onTabSelected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
@Override
public void onTabUnselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
@Override
public void onTabReselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
}
Here is the error I receive:
./MainActivity.java:28: cannot find symbol
symbol : method getPageTitle()
location: class PagerAdapter
tab.setText(PagerAdapter.getPageTitle());
^
1 error
Thanks, Riley
6 Answers

james white
78,399 PointsPoor Riley!
You never got an answer in this thread.
There are two other threads that do give the answer:
https://teamtreehouse.com/forum/what-am-i-doing-wrong-22 https://teamtreehouse.com/forum/adding-icons-to-tabs-code-challenge
I also kinda wonder why isn't there more cross referencing between posts/threads referring to the same challenge, so someone who is already frustrated (turning to the forum for help after failing to come up with the answer on their own after multiple attempts) doesn't become frustrated further by encountering forum threads/posts that are basically just "dead ends" (providing no viable/useful answer to the original poster).
However this forum thread/post is the most important (of the three referring to this challenge) because it has the full text of the challenge itself at the top and therefore comes up first in a forum search..
..and thus, just so anyone finding this thread gets "closure" (a viable answer), the line needed is actually:
tab.setText(pagerAdapter.getPageTitle(i));
..and it goes inside this class code:
public class MainActivity extends FragmentActivity implements
ActionBar.TabListener {
// Some code has been omitted for brevity!
public ActionBar mActionBar;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mActionBar = getActionBar();
mActionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
PagerAdapter pagerAdapter = new PagerAdapter(getSupportFragmentManager());
// Some code omitted for brevity...
for (int i = 0; i < pagerAdapter.getCount(); i++) {
ActionBar.Tab tab = mActionBar.newTab();
tab.setTabListener(this);
/*
* Add your code here to set the text!
*/
tab.setText(pagerAdapter.getPageTitle(i));
mActionBar.addTab(tab);
}
}

kristofferfreiholtz2
5,983 PointsOk theres one more thing that is wrong with the code.
You're trying to call the getPageTitle() method on a class not an instance.
Hint: Classes starts with Capital letters. Instances don't.

Chris Wolchesky
12,025 PointsVariable names are case sensitive. You declare the variable here as
PagerAdapter pagerAdapter = new PagerAdapter(getSupportFragmentManager());
but then you statically call the class itself by calling the function on PagerAdapter
instead of pagerAdapter
.

kristofferfreiholtz2
5,983 PointsHi Riley.
The method getPageTitle() requires a parameter of type int to know which tab it is.
Hope that helps you.

Riley MacDonald
2,542 PointsHi thanks for your answer but I believe I also already tried this but it did not work either. I tried the exact same code as above except I changed the following line:
tab.setText(PagerAdapter.getPageTitle(i));
Leads to the following error:
./MainActivity.java:28: non-static method getPageTitle(int) cannot be referenced from a static context
tab.setText(PagerAdapter.getPageTitle(i));
^
1 error
Thank you, Riley

Adiel Danda
6,375 PointsThank you,
tab.setText(PagerAdapter.getPageTitle(i));
was all that was required for me. I was omitting the "tab" object.
Thanks again

William Juste
7,822 PointsRiley, by writing "PagerAdapter" instead of "pagerAdapter" you are calling on the class instead of the method or instance of the object (I believe).
Your code is fine, just lowercase the capital P in "PagerAdapter" to "pagerAdapter" and it should work.
Ian Z
14,584 PointsIan Z
14,584 PointsThank you!!!