Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial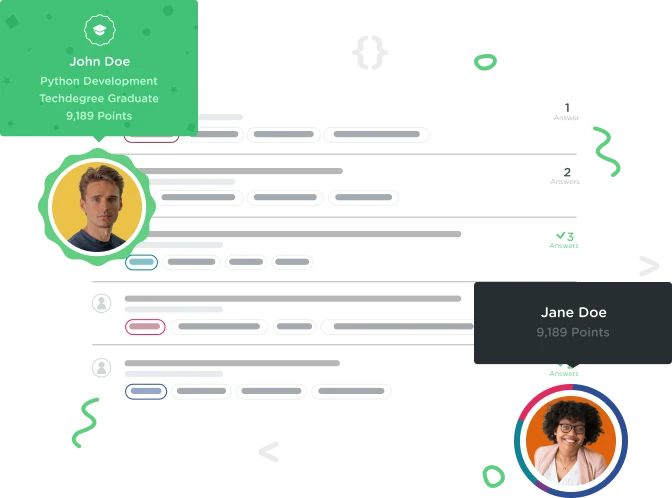
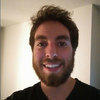
Ryan Hemrick
12,759 PointsValidation Code Challenge
So I was wondering if you could help me with some of these code challenges. As you might've seen when a member field name doesn't match the style we've talked about, I send an error about it. Let's write some code to validate the style of the field name.
I've added the method validatedFieldName it will return the validated field name. If the value passed in doesn't meet the requirements, throw an IllegalArgumentException. I'll keep you posted on the results every time you press check work.
Not sure where to go from here. I thought that the following code would pass:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
char secondLetter = fieldName.charAt(1);
// 1. Member fields must start with an 'm'
if (fieldName.charAt(0) != 'm') {
throw new IllegalArgumentException("There was an error!");
}
// 2. The second letter in the field name must be uppercased to ensure camel-casing
if (secondLetter != secondLetter.toUpperCase()) {
throw new IllegalArgumentException("There was an error!");
}
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}
6 Answers

Axel McCode
13,869 PointsYour code/syntax are well written the problem I had with this challenge is that the program entered a value that was similar to "m_test_variable", as you can see the second character in this string is not a letter. So your if statement would validate it. To prevent the function from validating this string you need to make sure that the second character in the string is a character while also validating that it is capitalized.
Try the following code, and take a look at the second if statement to see how I went about doing this.
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if(fieldName.charAt(0) != 'm' ){
throw new IllegalArgumentException("Error!");
}
if(!Character.isLetter(fieldName.charAt(1)) || fieldName.charAt(1) != Character.toUpperCase(fieldName.charAt(1))){
throw new IllegalArgumentException("Error!");
}
return fieldName;
}
}
The line:
!Character.isLetter(fieldName.charAt(1))
returns true, if the character at index 1 of the string "fieldName" is not a character, in which case the code would throw and IllegalArgumentException.
Hope this helps! :)
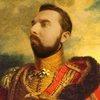
Bradley Demerich
11,688 PointsI'm a big fan of looking for the simplest and cleanest way of writing things out. Here's what I've got, in case you are looking for any additional way of doing things:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
if (fieldName.charAt(0) != 'm') {
throw new IllegalArgumentException("Must Start with M");
}
if (!Character.isLetter(fieldName.charAt(1)) ||
!Character.isUpperCase(fieldName.charAt(1))) {
throw new IllegalArgumentException("Second Letter must be upper Case!");
}
return fieldName;
}
}
I saw you use toUpperCase in your code. Would that make the character at position 1 an upper case letter?
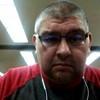
micram1001
33,833 PointsBetter to quote the requirements then just say error. The first letter 'm' must pass or throw an Illegal Argument Exception and the second letter must be uppercased.

Craig Dennis
Treehouse TeacherYour code looks great. This looks like the missing info you needed:
// This will return false
Character.isUpperCase('_');
char
datatypes do not have a method called toUpperCase
, so I assume you were getting a compiler error. Great job thinking it through!
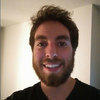
Ryan Hemrick
12,759 PointsThanks for the response Craig. I've managed to get the program running a few different ways thanks to the responses here. Also want to thank you for the great Java course. You really nailed the whole object/class ideas. It is also nice to work through a project rather than reading from a textbook. This course taught me more than an entire semester of Java programming in school. Treehouse's method of teaching is outstanding. Thanks guys!
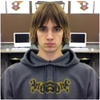
Ian Z
14,584 PointsCraig Dennis, you have the hardest code challenges :(
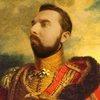
Bradley Demerich
11,688 PointsI'm glad he does. The more I have to work at figuring things out myself, the better at programing I'll become.

Craig Dennis
Treehouse Teacher(High five)

Justin Carothers
12,598 PointsI tried to minimize everything and this worked for me. Cheers!
if ((fieldName.charAt(0) !='m') || (!Character.isUpperCase(fieldName.charAt(1)))) {
throw new IllegalArgumentException("ILLEGAL!");
}
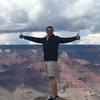
Andrew Wynyard
3,718 PointsI like this solution the best. It is much more simple than the others. I had this but separated out into two if statements. This allowed two illegal arguments for the two different issues. All of the answers above seem to be writing too much code for the problem, however I have just begun, and am probably wrong.
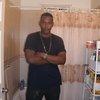
Otis Mason
794 PointsThis works public static String validatedFieldName(String fieldName) {
if(fieldName.charAt(0)!= 'm') { throw new IllegalArgumentException(" "); }
if(!Character.isUpperCase(fieldName.charAt(1))) {
throw new IllegalArgumentException(" ");
} return fieldName; }
}

Jess Sanders
12,086 Pointsprivate char validateGuess(char letter) {
if (!Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required."
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed") }
return letter;
}
- The above code is the example to follow.
- We care about charAt for this challenge, rather than indexOf
- In pseudocode, we want to check for the following condition, and throw an error: " if the character at index 0 of fieldName does not equal 'm' OR the character at index 1 of fieldName is not upper case"
Ryan Hemrick
12,759 PointsRyan Hemrick
12,759 PointsThanks so much for the response and explanation. Makes sense now!
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsSo this line:
if(!Character.isLetter(fieldName.charAt(1)) || fieldName.charAt(1) != Character.toUpperCase(fieldName.charAt(1)))
checks to see if 1.) is the character at index 1 a letter, and 2.) is the character at index 1 equal to the uppercase version of itself?