Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial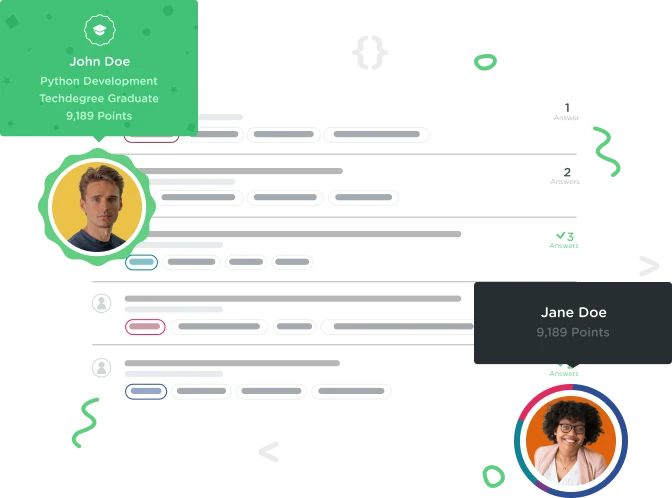
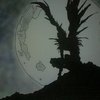
Lucas Santos
19,315 PointsWhat am I doing wrong on task 2
In the previous code challenge “Introducing Functions,” we called PHP’s array_sum function to calculate the sum of the values in an array. In this code challenge, we’ll create a function that mimics that one. First, create a function named mimic_array_sum. It should receive one argument, an array of numbers to be summed. Name that argument $array. (Be sure to specify a pair of curly brackets where the code this function executes will go.)
<?php
function mimic_array_sum( $array ){
$count = 0;
foreach( $array as $arrays ){
$count = $count + $array;
return $count;
}
}
return mimic_array_sum( $palindromic_primes );
$palindromic_primes = array(11, 757, 16361);
?>
5 Answers
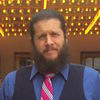
Adam Moore
21,956 PointsIn the foreach
function, you are breaking down your $array
and assigning each value to $arrays
to be passed each time foreach
is run. Therefore, you would need $count = $count + $arrays
. Like this:
<?php
function mimic_array_sum( $array ){
$count = 0;
foreach( $array as $arrays ){
$count = $count + $arrays;
return $count;
}
};
$palindromic_primes = array(11, 757, 16361);
echo mimic_array_sum( $palindromic_primes );
?>
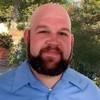
Sean Perryman
13,810 PointsFirst issue is declaring $palindromic_primes after you've tried to call it; when you call it during the call to mimic_array_sum, it doesn't exist.
Second issue I see is returning the output of mimic_array_sum; why not just echo or print it?
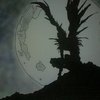
Lucas Santos
19,315 PointsTried this as well and it still not letting me pass.
<?php
function mimic_array_sum( $array ){
$count = 0;
foreach( $array as $arrays ){
$count = $count + $array;
return $count;
}
}
$palindromic_primes = array(11, 757, 16361);
echo mimic_array_sum( $palindromic_primes );
?>

Ari Winokur
Courses Plus Student 6,844 PointsSomething is seriously wrong here. I even copied and pasted Adam Moore's code in and it failed! This is the error: Bummer! The sum of the three numbers in the array should be 17129. (If you need assistance with the code inside the function, check out the starting code for the previous Introducing Functions challenge.)
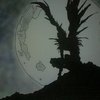
Lucas Santos
19,315 PointsFor those who haven't figured this out as well you just need to put return $count;
outside the mimic_array_sum function and it will pass.
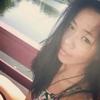
Rosey Liu
2,587 PointsNot sure if this problem is solved for you but I see what is wrong here. Make sure the return command is after the closing curly bracket of the foreach command, like this:
function mimic_array_sum($array) {
$count = 0;
foreach($array as $arrays) {
$count = $count + $arrays;
}
return $count;
};
$palindromic_primes = array(11, 757, 16261);
echo mimic_array_sum($palindromic_primes);
?>```
Hope this helps! :)
Sean Perryman
13,810 PointsSean Perryman
13,810 PointsYou beat me to the punch! Good job :)
Adam Moore
21,956 PointsAdam Moore
21,956 PointsHaha, thanks! :)
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsI tried that and it still doesn't pass me, it says: Bummer! The sum of the three numbers in the array should be 17129. (If you need assistance with the code inside the function, check out the starting code for the previous Introducing Functions challenge.)
Adam Moore
21,956 PointsAdam Moore
21,956 PointsMaybe refresh the page or close your browser and reopen it. When I copy and paste the code above into the code challenge, it passes.
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsStill no, very strange.
Sean Perryman
13,810 PointsSean Perryman
13,810 PointsI just copied and pasted the code that Adam provided into the code challenge window and it passed no problem.
daniwao
13,125 Pointsdaniwao
13,125 PointsHey Adam, would you mind explaining why you put
foreach($arrays as $array) { $count = $count + $arrays;
and why that works. That's one aspect of this foreach loop that I'm not quite understanding. To me, I thought I could just pass in the $array argument from my function and that it would work. Not sure about the placement or maybe you can give me an example with just different words instead of $array.
Thanks.
Adam Moore
21,956 PointsAdam Moore
21,956 PointsBasically, what
foreach()
does is this:You have to tell the
foreach()
function what array to pass in, which is the$the_main_array
argument. Then, the function uses$each_array_item_in_main_array
and runs the function "for each" item in the main array. So,foreach()
needs an array, and then it needs a variable to use within the function to run the function on; i.e., "for each"... "array item in the main array"... from "the main array". Without the variable that comes after the "as", the function wouldn't have a variable to use each time the function ran for each of the items within the array.At least this is how I understand it. I may be wrong on some parts of it, but this is how it makes sense to me.