Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial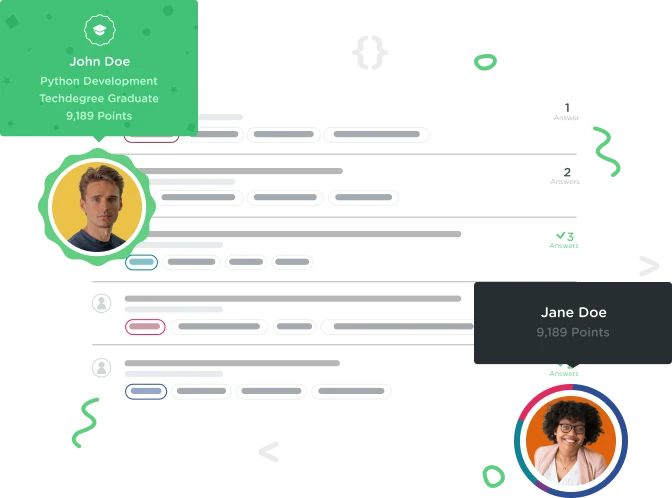
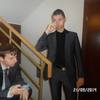
Aurelian Spodarec
7,369 PointsWhats wrong with my features boxes?
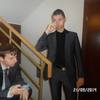
Aurelian Spodarec
7,369 PointsHi, I'm looking to make this.
I did figure out that the dash - and content is connected somewhere else but still, i don't get it , i mean this doesnt work like if i would do it alone. It works perfectly alone but here it doesnt .
Also what do you think about the banner code on CSS? do you think its good? it looks fairly good on webpage , but the code is messy i guess.
2 Answers

Ryan Duchene
Courses Plus Student 46,022 PointsI'm going to offer you a few tips on improving your code. I learned a lot of these lessons the hard way. :)
Forewarning, this is going to run a little long.
Honestly, it looks like you're making it display correctly, but your code is tripping over itself and some rules you defined earlier are coming back to bite you. Take a look at this, for instance:
Basically, you would like for those boxes to have a black background. So that's what you set. The problem is that you've already set any div
s within a .container
to have a gray background. And since the latter is more specific, it overrides the former.
CSS
Guild says this in his Modular CSS course, but you want to avoid using both broad and specific selectors as much as possible. Exactly how many div
s do you plan to have under a .container
class? Probably a lot. It's a very broad selector. Probably too broad. You probably plan on having more than one #box-container
, so maybe that selector (as an ID selector) is a little too specific.
The reasoning behind that rule is that broad selectors tend to leak out and get applied to elements you never intended them to be applied to. Conversely, overly specific selectors can never be reused.
For the most part, anytime you select an element by tag name, it's too broad. Exceptions to the rule include the typographic elements (p
, h1-6
, strong
, em
, u
, q
, blockquote
, cite
, etc.), as the purpose of those elements is always tied to the tag name. As long as you scope them (e.g., #article blockquote
), you should be good. Elements like body
and html
are also exceptions because there's only one of each on the page. Lastly, if you're setting styles you really, really want to be global (like changing the color of all links across the page and removing their underlines), then go right ahead.
To avoid selecting a whole lot of tag names, use a class or ID on everything related to layout. I like using IDs for high-level elements that any given page only has one of (my primary header, my primary footer, my primary navigation, etc.) and classes for everything else.
Be descriptive with your class names: rather than just .box
(which is a very generic name that could be a panel, a button, a blockquote, or a textarea) use .feature
, .col
, or .panel
. Also, don't use class names like .background-blue
; what will happen if you decide to change the background to red
?
Keep the naming scheme among your classes similar. Don't use .camelCasing
for some classes and .hyphens-between-words
for others. Using lowercase with hyphens is the globally accepted standard for CSS. Hint hint, you should probably use it. :)
Abbreviating your class names is generally frowned upon, but there are a ton of exceptions. Everyone knows that .bg
translates to "background"; everyone knows that .col
translates to "column." Personally, I get sick and tired of typing .container
all over the place, so I just shorten it to .cc
and add a comment in my CSS saying such.
Speaking of comments, commenting your CSS is always a good thing. If you comment your classes well, you can get away with breaking a few rules.
Don't be afraid to use pseudo-selectors in place of classes whenever you can. They're more flexible than classes are, as they'll adjust to the HTML where classes won't. Instead of adding the .last
class to the last link in your nav and changing it whenever you update the nav, use the :last-child
pseudo-selector.
Keep your indentation in CSS consistent. It might seem like a little paranoid right now, but it's much easier to read and edit when it's indented properly. When you upgrade to Sass in a few months, remembering this is especially important.
HTML
To add to that, you don't need so many div
s in your HTML. One of the big additions in HTML5 were all those semantic tags: header
, nav
, section
, hgroup
, footer
, article
, aside
, and main
, just to name a few. Use those in place of most of those div
s to indicate that this is a high-level element, not just a container or something. None of those elements have any display differences from div
; they just make your HTML a whole lot easer to read and understand.
As with CSS, keep your indentation in check. It's easier to let it slip away here, but it's all the more important to keep maintaining it, because losing track of it will cause your sense of the hierarchy to get messed up.
Tying in with the class-naming in CSS, try to keep your HTML self-documenting. It's very rare for me to comment through my HTML because of the way I've arranged my elements:
<section id="post-135" class="post format-standard author-duchenerc sticky">
<ul class="post-meta">
<li class="post-author">
Author: Ryan Duchene
</li>
<li class="post-date">
Date: November 30, 2014
</li>
<li class="post-cats">
Categories: HTML, CSS, Layout
</li>
</ul>
<article class="post-content">
<h1>Post title lorem ipsum</h1>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
</p>
</article>
</section>
When I write a new tag, its name should come first (obviously), but the second attribute should be id
, if you've assigned any, and the one after that should be class
. (That particular order is personal preference; if you have something else you like, go for it.) Between the semantic tag name, the IDs, the descriptive class names, and the content in the HTML, anybody can pick up the general idea of your code just by glancing through your HTML. If they want more info, the stylesheet beckons.
Of course, commenting in your HTML is perfectly fine, especially the end-of-element comments. I just prefer keeping it to a minimum.
Layout techniques
Guil covers all of these techniques and more in his Layout Techniques course, but I'll just go over the basics here. There are three main techniques that I find useful: floats, inline-block, and flexbox.
Floats
Floats were originally designed to allow elements like images to be positioned in such a manner that they would shift toward a certain direction and other elements (especially inline elements) would flow around them. An example would be floating an image inside an article; the image is stuck to the left side of the article, and the text flows around it.
Floats have become a very popular layout tool as they provide a lot of flexibility, and even some source-independent order if used properly. When used for layout, they're accompanied by the clearfix hack, as elements that contain only floated children will collapse without the hack.
Floats have solid browser support, even in browsers older than IE6. ("Fun" Fact: When IE6 was released, you could board a plane in the US while carrying a pocketknife. Yes, IE6 is older than 9/11. And to think it's still used today.)
I recommend using floats in large-scale layout situations where you have a lot of control over where the content will be. Column layouts, grids, navs, and a lot of other large-scale UI components fall in here.
Inline-block
Inline blocks are, ironically enough, a cross between inline elements and block elements. Inline elements wrap around each other very nicely, making them good for text. Block elements stack on top of each other, making them good for layout. Inline-block runs the gap, leaving you with block elements that wrap around well.
The downside to inline-block is that it just does not give you the control that floats do. Everything sticks to the left like glue; there's no way to center anything or reverse its order. The upside over floats is that no clearfix hack is required, and there are more than a few situations when the clearfix hack is impractical.
Inline-block layouts have solid browser support, missing only IE6 IIRC. Use them for smaller UI components where you don't have much fine control, such as buttons, form fields, and (usually) horizontal lists.
Flexbox
Flexbox is the layout system of the future. It lets you do just about anything, and gives you a ton of control over everything. Center the items vertically, align them right, reverse their order, and pull the second one to the end. All just seven lines of CSS, with no changes to the HTML. And, unlike floats, it works vertically as well as horizontally.
Flexbox eats floats for breakfast in all but one area: browser support. Between its three different specs, flexbox covers all modern browsers; only IE8 and IE9 are left out in the cold. Those two browsers have a boatload of users, though. Similarly to floats, you need to establish a flexbox context in order for it to work, which means that it's impractical to apply it to the inline-block stuff.
Use flexbox everywhere you'd use floats, and then some.
Sorry about the wall of text. Hope you enjoyed. And, as a parting gift, I'll leave you with your layout in a CodePen, with pretty code and a pretty face. The CSS file is about 50 lines longer than yours is.
EDIT: Fixed link to CodePen. No, not a joke. Not sure how that happened. :)
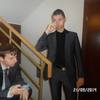
Aurelian Spodarec
7,369 PointsWow, thank you very much!
It seem too much information to suck in . Ill try to do something about it but umm, it seem pretty hard now . I though I'm pretty close to get over it . I mean as i 'can' layout a webpage , though the code is a bit mess , if i improve that , i think i can be ready .
Everything on this wall is useful. I think there should be different name for the topic for others to find about this too.
When using asidebar or footer header etc.. mostly about two sides, they need and id so one goes to the lest and other goes to the right no? so as header and similar if I'm right?
Is that CodePen a bug or a joke ? xD

Ryan Duchene
Courses Plus Student 46,022 PointsIt is a lot of information, I'll agree with you on that. :) Guil teaches most of his things in the various CSS courses around Treehouse. They're all gems.
Use IDs when you're sure that there's only ever going to be one of those elements on the page. There's only ever one header. There's only ever one post with the ID of 135.
Also, I fixed the link to the pen; it's in the original post above.
Happy coding!
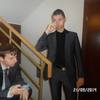
Aurelian Spodarec
7,369 PointsWow , i have a lot to learn now . Well, basicaly i have to learn what your wrote which might take some time . This is like one of the best ways to learn in my opinion . Study others code and how the effects is achieved.
Bdw, i dont know if you heared of brackets. Its a great editor.
Thank you very much!
Happy coding and Merry Christmas!

Ryan Duchene
Courses Plus Student 46,022 PointsAs Picasso (I believe) said,
Good artists copy. Great artists steal.
Learning by mimicking the designs of others is an art, and through it, you can begin to grasp an idea of how to best construct a website. :)
As for the topic of editors, I have used Brackets some, but that was a long time ago. I wanted to like it, but it just didn't have a lot of the features I was looking for. I should try it again.
I started out by using TextWrangler, which is still a great utility editor, very stable and lightweight. Then I switched to Aptana Studio, which is a web IDE. It had a few features that I wasn't used to in TextWrangler, including a lot (and I mean a lot) of automatic code completion. It was too slow, clunky, and rigid though, so I ended up ditching it. I moved on to TextMate, which is another incredible text editor. Very open and easy to extend, but a slow community that's more dedicated to the Mac platform than to the web platform.
My current text editor is GitHub Atom. I've been using it for about six months now, and I absolutely love it. A very open platform with a vibrant community around it. The packages you can install as add-ons are insanely awesome. We're talking Spotify and iTunes integration, StackOverflow being a few keystrokes away, an incredible Git GUI, and tons of others, all just a few clicks away. The only downsides are that it takes awhile to load up and that automatic updates are touchy on Macs and impossible on Windows and Linux (though coming soon).
Throughout all that, I used Sublime Text some and Brackets some as well.
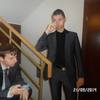
Aurelian Spodarec
7,369 PointsUm, 3 out of 5 , i never heared it. And there are a lot more of them : p
From when i started to code , it was Sublime Text. I just basicaly felt in love with it because of how it looks. Although i love xCode too, its just soo nice , and the code to .
You should definitly check out brackets now , in this days, the new one unless you are allready using : p. Maybe it doesnt close tags and that stuff, but if you interested in live view etc.. i would recomend it. I mean , its neary same as sublime text, though if some, just slightly changes were made to brakcets, i think it could dominate sublime in my opinion .
I would still prefere sublime text to write my HTML and the starting CSS blocks .

Ryan Duchene
Courses Plus Student 46,022 PointsI'll check it out, then, and see what's changed. :) I think the last version I tried was like Sprint 36 or something, and I see they don't even use that kind of versioning anymore.
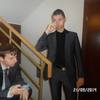
Aurelian Spodarec
7,369 PointsHi,
As i was learning the code, and i famed up with great ideas , i mean this teaches me soo much . Treehouse should have done something like this .
But to my point, i can't find the class .c not .cc (content) but the .c class thats on the nav side . If i delete it the nav goes bad but i can't find the class .c . Could you help me please?
EDIT: I just figured it out . Lol what a logic. This is soo new to me but its zoo easy your way or the one that made it . I mean the things how their done are just very very easy that i wouldn't think about it. Im not sure if it uses CSS3 or what but its just awesome ! Though sometimes the older method is better but best to know both :D
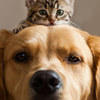
Devin Scheu
66,191 PointsHaha, was gonna respond to this post, but Ryan Duchene's answer is hard to beat. And something a little off topic, how did you guys get mod? If possible I would like to apply also but i don't know where to start.
-Kind Regards, Devin

Ryan Duchene
Courses Plus Student 46,022 PointsFor Aurelian: The .c
class is just a class that says "I'm some random container class, but I'm not the primary container, so don't style me like that." I also sometimes use .ccc
, which says "I am a primary container, but I extend the full width (100%) of the page, not 95% or whatever the regular containers get."
For Devin: Every now and again, I go a little haywire and write a really long forum post about stuff I've learned a lot about. My last one was on WordPress's Walker class with comments. It's quite fun to write longer posts like that, actually. I should really start a blog.
About becoming a mod. I was contacted via email by Treehouse with an invitation to join; I believe it's invite-only, but I'm not sure. There's a number of criteria for being asked (right from my manual):
- An in-depth understanding of the Treehouse app & learning experience
- Been learning with Treehouse for 3+ months
- At least 5,000 points on their Treehouse profile
- Demonstrated they are consistently helpful & engaging
- The ability to be patient and approachable in the forum
And, in describing moderators, this it what my manual has to say:
A Treehouse moderator is an engaging and valuable student from the community who stands out as exceptionally helpful and informative to others. As a Treehouse moderator you are a trusted voice in the community and we can rely on you to help and encourage others, while being proactive in the forum.
As a representation of Treehouse, the tone of a moderator is important and they are expected to be patient and friendly. Students should find moderators approachable and feel like they can ask questions with ease. We expect explanations to other students to be clear and helpful for both initial questions and ongoing conversations. The friendliness of moderators also carries over into their engagement with others. As well as answering questions, moderators enjoy being involved in conversations and sharing their thoughts and suggestions with the community.
Moderators have an in depth understanding of not only the content in the Treehouse library, but also the Treehouse app itself and the overall learning experience it offers. They’re often students who have already exhibited that they are extremely helpful in the forum; demonstrated extensive knowledge in a specific topic in the Library; or are dedicated to their Treehouse education and guiding others.
I can't put it any better than that. So, to answer your question, I guess that the best way to become a mod is to act like one. :)
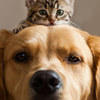
Devin Scheu
66,191 PointsAh I see, which you are all of the above. Thank you for the quick answer. :)
Ryan Duchene
Courses Plus Student 46,022 PointsRyan Duchene
Courses Plus Student 46,022 PointsWhat problems exactly do you seem to be having? I can see your boxes, but I'm not sure how you want them to appear. Are you looking for a four column layout?