Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial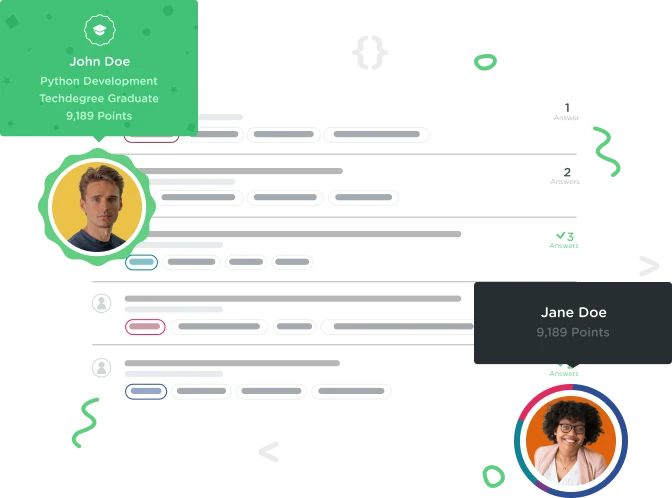

Josh Chou
Courses Plus Student 1,267 PointsWhen to use self.
Im writing my first app, I have about 400 lines of code but I am still confused on using "self." Is there an easy example of when to and not to use "self."?
3 Answers

Aaron Daub
Courses Plus Student 96 PointsDot notation calls getters/setters on the object that you use the dot notation on.
Imagine you want an object to store your name.
@interface TTHMyName : NSObject{
NSString* name;
}
TTHMyName* myName = [[TTHMyName alloc] init];
myName->name = @"Aaron";
But that isn't very good, because if something else cared about what your name was it wouldn't be able to tell that it was changed. So now you write a method for setting the name. You could call it setName:.
- (void)setName:(NSString*)aName{
if(name != aName){
name = aName;
}
}
If you wanted, you could make that setName method more complicated to let people know when it is being changed. This is the basic premise behind KVO.
Anyway, it would make sense for all of your "setters" to follow this pattern of setVariableName:. That way you wouldn't have to remember what classes define what setters. So that's the rule, setters are setX:.
There are also getters, which just return the current value in an instance variable (or calculate a value dynamically in some cases). Why not just read it directly? While sometimes the class that defines a getter might want to keep an internal representation of data different than the data that they expose. For example, a date inside of a class might be represented as a number of seconds while the getter might return a string in the form of @"2013-08-23". Getters are a great place for the internal representation to be transformed into the external representation (and setters are a great place for the inverse to happen)
If a setter is setX: is a getter getX? No, a getter is just X. The getter to match the setName: setter would simply be "name".
Anyway, it might get really messy to have a lot of square brackets around. Especially if you want to get an instance variable from an instance variable from an instance variable, etc.
What is more readable?
NSMutableArray* subviews = [[[[[self navigationController] presentingViewController] view] superview] subviews] mutableCopy];
or
NSMutableArray* subviews = self.navigationController.presentingViewController.view.superview.subviews.mutableCopy;
The compiler will transform the dot notation into the appropriate message sends for you, making your life easier.
How does it know whether to use a getter or a setter? Simply put, it can infer your meaning based on the context the dot notation is used in.
If you use dot notation to the left of an assignment operator (=) it will resolve to a setter. Otherwise it will be a getter.
self.view = [[UIView alloc] initWithFrame:CGRectZero];
That would be a setter.
self.view.backgroundColor = [UIColor redColor];
The self.view resolves to a getter and the .backgroundColor resolves to a setter.
Fun tidbit: you can use dot notation to call methods on CLASSES as well! That's because, under the hood, UIColor is an object!
UIColor.redColor; // This is the same as [UIColor redColor];
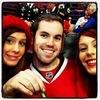
Patrick Cooney
12,216 PointsShort answer, "self" refers to the current instance of the class. Self makes it so that you're returning the value for the current instance.

Aaron Daub
Courses Plus Student 96 PointsI think he meant "self.". But Patrick is right, mostly. "self" refers to the object that is responding to the message. It doesn't necessarily need to be an instance of a class in the traditional sense. "NSObject" is an instance of a meta-class. But self in the context of a class method on NSObject refers to the NSObject class object.
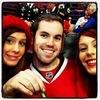
Patrick Cooney
12,216 PointsHa! Sorry. I saw the dot in the self in the title and thought it was a period and I apparently missed the periods after the other times he wrote self.

Jon Harvell
3,870 PointsIn the iOS Foundations Course there is a video called "Strong vs Weak" that is 7:25 long. In the Book.m file it defines an instance method for "description" that passes the variables "self.title" and "self.author" through the %@ tokens as arguments. Then the "ViewController.m" file gives those variables values: @"Brave New World" and @"Aldous Huxely." Then, under the button pressed action "self.book" is passed through the %@ token as the argument.
Does the "self" in "self.book" refer to that specific classes (ViewController.m) definition of "book?" So, if you had another class that defined a different title and author of the book class, self would then reference that particular class's definition of self.
I'm just confused at to why you need to reference self for "self.title" and "self.author" if your referencing self in the "self.book", which contains the title and author variables?